toystack
Version:
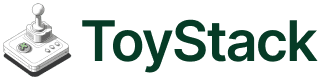
102 lines (89 loc) • 2.46 kB
text/typescript
import { exec, execSync } from "child_process";
import fs from "fs";
import path from "path";
function getCurrentRepositoryAndBranch() {
try {
const repositoryName = execSync("basename `git rev-parse --show-toplevel`")
.toString()
.trim();
const branchName = execSync("git rev-parse --abbrev-ref HEAD")
.toString()
.trim();
return { repositoryName, branchName };
} catch (error) {
console.error("Error fetching repository or branch:", error);
return null;
}
}
function getGitUserName() {
try {
return execSync("git config user.name").toString().trim();
} catch (error) {
console.error("Error fetching git user name:", error);
return null;
}
}
function getCurrentRepositoryName() {
try {
const repositoryName = execSync("basename `git rev-parse --show-toplevel`")
.toString()
.trim();
return repositoryName;
} catch (error) {
console.error("Error fetching repository name :", error);
return null;
}
}
async function buildProject(directory: string) {
return new Promise<void>((resolve, reject) => {
const buildCommand = "npm run build";
exec(buildCommand, { cwd: directory }, (error, stdout) => {
if (error) {
console.error("Build failed:", error.message);
reject(`Build failed: ${error.message}`);
return;
}
console.log(stdout);
resolve();
});
});
}
function pathExists(targetPath: string): boolean {
try {
return fs.existsSync(targetPath);
} catch (error) {
console.error(`Error checking path: ${error}`);
return false;
}
}
function identifyFramework(projectPath: string) {
try {
const packageJsonPath = path.join(projectPath, "package.json");
if (!fs.existsSync(packageJsonPath)) {
throw new Error("package.json not found in the specified directory.");
}
const packageJson = JSON.parse(fs.readFileSync(packageJsonPath, "utf-8"));
const dependencies = packageJson.dependencies || {};
const devDependencies = packageJson.devDependencies || {};
// Detect frameworks
const isReact = "react" in dependencies || "react" in devDependencies;
const isAstro = "astro" in dependencies || "astro" in devDependencies;
// Determine framework type
if (isAstro) {
return "Astro";
} else if (isReact) {
return "React";
}
} catch (error) {
console.error("Error:", error);
return false;
}
}
export {
getCurrentRepositoryAndBranch,
getGitUserName,
getCurrentRepositoryName,
buildProject,
pathExists,
identifyFramework,
};