react-native-gradients
Version:
A simple gradient library for React Native.
90 lines (64 loc) • 2.36 kB
Markdown
//badge.fury.io/js/react-native-gradients.svg)](https://badge.fury.io/js/react-native-gradients)
> A React Native alternative to Linear and Radial gradients, using SVG!
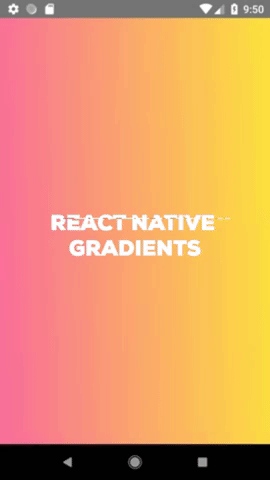 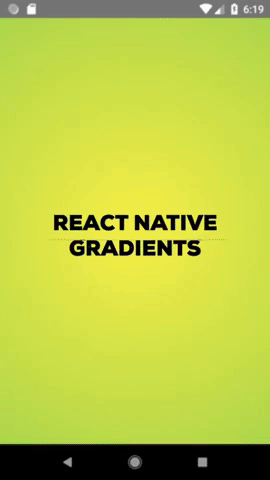 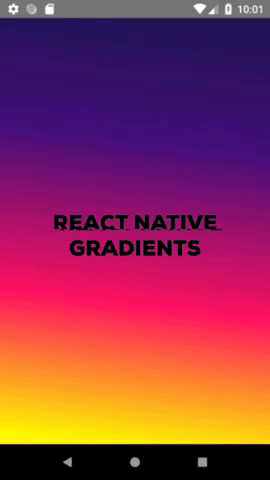
**Note**: This library supports only React Native 0.60+ versions
Install `react-native-gradients` using your package manager
```sh
npm install --save react-native-gradients react-native-svg
```
```sh
yarn add react-native-gradients react-native-svg
```
As it uses [React Native SVG](https://github.com/react-native-svg/react-native-svg), you need to install the pod dependencies using following command
```sh
cd ios
pod install
```
```js
const colorList = [
{offset: '0%', color: '#231557', opacity: '1'},
{offset: '29%', color: '#44107A', opacity: '1'},
{offset: '67%', color: '#FF1361', opacity: '1'},
{offset: '100%', color: '#FFF800', opacity: '1'}
]
```
```jsx
<LinearGradient colorList={colorList} angle={90}/>
```
```js
const colorList = [
{offset: '0%', color: '#231557', opacity: '1'},
{offset: '29%', color: '#44107A', opacity: '1'},
{offset: '67%', color: '#FF1361', opacity: '1'},
{offset: '100%', color: '#FFF800', opacity: '1'}
]
```
```jsx
<RadialGradient x="50%" y="50%" rx="50%" ry="50%" colorList={colorList}/>
```
This library uses `<svg />` components. An option for other formats of "images" is using the React Native component `<ImageBackground />` but for `svg`s that is not possible (at least for now).
A good option is wrap your Gradient component into a `<View />` component and make that component has `position: absolute` style such as
```jsx
import { RadialGradient } from 'react-native-gradients';
const BackgroundGradient = ({ style, children }) => (
<View style={[styles.gradientBg, style]}>
<RadialGradient />
</View>
);
const styles = StyleSheet.create({
gradientBg: {
position: absolute,
width: "100%",
height: "100%",
},
});
```
[![npm version](https: