lambda-api
Version:
Lightweight web framework for your serverless applications
901 lines (667 loc) • 82.9 kB
Markdown
[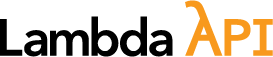](https://serverless-api.com/)
[](https://github.com/jeremydaly/lambda-api/actions/workflows/build.yml)
[](https://www.npmjs.com/package/lambda-api)
[](https://www.npmjs.com/package/lambda-api)
[](https://coveralls.io/github/jeremydaly/lambda-api?branch=main)
### Lightweight web framework for your serverless applications
Lambda API is a lightweight web framework for AWS Lambda using AWS API Gateway Lambda Proxy Integration or ALB Lambda Target Support. This closely mirrors (and is based on) other web frameworks like Express.js and Fastify, but is significantly stripped down to maximize performance with Lambda's stateless, single run executions.
## Using AWS SDK v2?
lambda-api@v1 is using AWS SDK v3.
If you are using AWS SDK v2, please use lambda-api@v0.12.0.
## Simple Example
```javascript
// Require the framework and instantiate it
const api = require('lambda-api')();
// Define a route
api.get('/status', async (req, res) => {
return { status: 'ok' };
});
// Declare your Lambda handler
exports.handler = async (event, context) => {
// Run the request
return await api.run(event, context);
};
```
For a full tutorial see [How To: Build a Serverless API with Serverless, AWS Lambda and Lambda API](https://www.jeremydaly.com/build-serverless-api-serverless-aws-lambda-lambda-api/).
## Why Another Web Framework?
Express.js, Fastify, Koa, Restify, and Hapi are just a few of the many amazing web frameworks out there for Node.js. So why build yet another one when there are so many great options already? One word: **DEPENDENCIES**.
These other frameworks are extremely powerful, but that benefit comes with the steep price of requiring several additional Node.js modules. Not only is this a bit of a security issue (see Beware of Third-Party Packages in [Securing Serverless](https://www.jeremydaly.com/securing-serverless-a-newbies-guide/)), but it also adds bloat to your codebase, filling your `node_modules` directory with a ton of extra files. For serverless applications that need to load quickly, all of these extra dependencies slow down execution and use more memory than necessary. Express.js has **30 dependencies**, Fastify has **12**, and Hapi has **17**! These numbers don't even include their dependencies' dependencies.
Lambda API has **ZERO** dependencies. _None_. _Zip_. _Zilch_.
Lambda API was written to be _extremely lightweight_ and built specifically for **SERVERLESS** applications using AWS Lambda and API Gateway. It provides support for API routing, serving up HTML pages, issuing redirects, serving binary files and much more. Worried about observability? Lambda API has a built-in logging engine that can even periodically sample requests for things like tracing and benchmarking. It has a powerful middleware and error handling system, allowing you to implement just about anything you can dream of. Best of all, it was designed to work with Lambda's Proxy Integration, automatically handling all the interaction with API Gateway for you. It parses **REQUESTS** and formats **RESPONSES**, allowing you to focus on your application's core functionality, instead of fiddling with inputs and outputs.
### Single Purpose Functions
You may have heard that a serverless "best practice" is to keep your functions small and limit them to a single purpose. I generally agree since building monolith applications is not what serverless was designed for. However, what exactly is a "single purpose" when it comes to building serverless APIs and web services? Should we create a separate function for our "create user" `POST` endpoint and then another one for our "update user" `PUT` endpoint? Should we create yet another function for our "delete user" `DELETE` endpoint? You certainly could, but that seems like a lot of repeated boilerplate code. On the other hand, you could create just one function that handled all your user management features. It may even make sense (in certain circumstances) to create one big serverless function handling several related components that can share your VPC database connections.
Whatever you decide is best for your use case, **Lambda API** is there to support you. Whether your function has over a hundred routes, or just one, Lambda API's small size and lightning fast load time has virtually no impact on your function's performance. You can even define global wildcard routes that will process any incoming request, allowing you to use API Gateway or ALB to determine the routing. Yet despite its small footprint, it gives you the power of a full-featured web framework.
## Table of Contents
- [Simple Example](#simple-example)
- [Why Another Web Framework?](#why-another-web-framework)
- [Single Purpose Functions](#single-purpose-functions)
- [Table of Contents](#table-of-contents)
- [Installation](#installation)
- [Requirements](#requirements)
- [Configuration](#configuration)
- [Recent Updates](#recent-updates)
- [v0.11: API Gateway v2 payload support and automatic compression](#v011-api-gateway-v2-payload-support-and-automatic-compression)
- [v0.10: ALB support, method-based middleware, and multi-value headers and query string parameters](#v010-alb-support-method-based-middleware-and-multi-value-headers-and-query-string-parameters)
- [Routes and HTTP Methods](#routes-and-http-methods)
- [Returning Responses](#returning-responses)
- [Async/Await](#asyncawait)
- [Promises](#promises)
- [A Note About Flow Control](#a-note-about-flow-control)
- [Route Prefixing](#route-prefixing)
- [Debugging Routes](#debugging-routes)
- [REQUEST](#request)
- [RESPONSE](#response)
- [status(code)](#statuscode)
- [sendStatus(code)](#sendstatuscode)
- [header(key, value [,append])](#headerkey-value-append)
- [getHeader(key [,asArray])](#getheaderkey-asarray)
- [getHeaders()](#getheaders)
- [hasHeader(key)](#hasheaderkey)
- [removeHeader(key)](#removeheaderkey)
- [getLink(s3Path [, expires] [, callback])](#getlinks3path--expires--callback)
- [send(body)](#sendbody)
- [json(body)](#jsonbody)
- [jsonp(body)](#jsonpbody)
- [html(body)](#htmlbody)
- [type(type)](#typetype)
- [location(path)](#locationpath)
- [redirect([status,] path)](#redirectstatus-path)
- [cors([options])](#corsoptions)
- [error([code], message [,detail])](#errorcode-message-detail)
- [cookie(name, value [,options])](#cookiename-value-options)
- [clearCookie(name [,options])](#clearcookiename-options)
- [etag([boolean])](#etagboolean)
- [cache([age] [, private])](#cacheage--private)
- [modified(date)](#modifieddate)
- [attachment([filename])](#attachmentfilename)
- [download(file [, filename] [, options] [, callback])](#downloadfile--filename--options--callback)
- [sendFile(file [, options] [, callback])](#sendfilefile--options--callback)
- [Enabling Binary Support](#enabling-binary-support)
- [Path Parameters](#path-parameters)
- [Wildcard Routes](#wildcard-routes)
- [Logging](#logging)
- [Logging Configuration](#logging-configuration)
- [Log Format](#log-format)
- [Access Logs](#access-logs)
- [Logging Levels](#logging-levels)
- [Custom Logging Levels](#custom-logging-levels)
- [Adding Additional Detail](#adding-additional-detail)
- [Serializers](#serializers)
- [Sampling](#sampling)
- [Middleware](#middleware)
- [Restricting middleware execution to certain path(s)](#restricting-middleware-execution-to-certain-paths)
- [Specifying multiple middleware](#specifying-multiple-middleware)
- [Method-based middleware](#method-based-middleware)
- [Clean Up](#clean-up)
- [Error Handling](#error-handling)
- [Error Types](#error-types)
- [Error Logging](#error-logging)
- [Namespaces](#namespaces)
- [CORS Support](#cors-support)
- [Compression](#compression)
- [Execution Stacks](#execution-stacks)
- [Lambda Proxy Integration](#lambda-proxy-integration)
- [ALB Integration](#alb-integration)
- [Configuring Routes in API Gateway](#configuring-routes-in-api-gateway)
- [Reusing Persistent Connections](#reusing-persistent-connections)
- [TypeScript Support](#typescript-support)
- [Contributions](#contributions)
- [Are you using Lambda API?](#are-you-using-lambda-api)
## Installation
```
npm i lambda-api --save
```
## Requirements
- AWS Lambda running **Node 8.10+**
- AWS API Gateway using [Proxy Integration](#lambda-proxy-integration)
## Configuration
Require the `lambda-api` module into your Lambda handler script and instantiate it. You can initialize the API with the following options:
| Property | Type | Description |
| -------------------- | --------------------- | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| base | `String` | Base path for all routes, e.g. `base: 'v1'` would prefix all routes with `/v1` |
| callbackName | `String` | Override the default callback query parameter name for JSONP calls |
| logger | `boolean` or `object` | Enables default [logging](#logging) or allows for configuration through a [Logging Configuration](#logging-configuration) object. |
| mimeTypes | `Object` | Name/value pairs of additional MIME types to be supported by the `type()`. The key should be the file extension (without the `.`) and the value should be the expected MIME type, e.g. `application/json` |
| serializer | `Function` | Optional object serializer function. This function receives the `body` of a response and must return a string. Defaults to `JSON.stringify` |
| version | `String` | Version number accessible via the `REQUEST` object |
| errorHeaderWhitelist | `Array` | Array of headers to maintain on errors |
| s3Config | `Object` | Optional object to provide as config to S3 sdk. [S3ClientConfig](https://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/clients/client-s3/interfaces/s3clientconfig.html) |
```javascript
// Require the framework and instantiate it with optional version and base parameters
const api = require('lambda-api')({ version: 'v1.0', base: 'v1' });
```
## Recent Updates
For detailed release notes see [Releases](https://github.com/jeremydaly/lambda-api/releases).
### v0.11: API Gateway v2 payload support and automatic compression
Lambda API now supports API Gateway v2 payloads for use with HTTP APIs. The library automatically detects the payload, so no extra configuration is needed. Automatic [compression](#compression) has also been added and supports Brotli, Gzip and Deflate.
### v0.10: ALB support, method-based middleware, and multi-value headers and query string parameters
Lambda API now allows you to seamlessly switch between API Gateway and Application Load Balancers. New [execution stacks](execution-stacks) enables method-based middleware and more wildcard functionality. Plus full support for multi-value headers and query string parameters.
## Routes and HTTP Methods
Routes are defined by using convenience methods or the `METHOD` method. There are currently eight convenience route methods: `get()`, `post()`, `put()`, `patch()`, `delete()`, `head()`, `options()` and `any()`. Convenience route methods require an optional _route_ and one or more handler functions. A _route_ is simply a path such as `/users`. If a _route_ is not provided, then it will default to `/*` and will execute on every path. Handler functions accept a `REQUEST`, `RESPONSE`, and optional `next()` argument. These arguments can be named whatever you like, but convention dictates `req`, `res`, and `next`.
Multiple handler functions can be assigned to a path, which can be used to execute middleware for specific paths and methods. For more information, see [Middleware](#middleware) and [Execution Stacks](#execution-stacks).
Examples using convenience route methods:
```javascript
api.get('/users', (req,res) => {
// do something
})
api.post('/users', (req,res) => {
// do something
})
api.delete('/users', (req,res) => {
// do something
})
api.get('/users',
(req,res,next) => {
// do some middleware
next() // continue execution
}),
(req,res) => {
// do something
}
)
api.post((req,res) => {
// do something for ALL post requests
})
```
Additional methods are support by calling `METHOD`. Arguments must include an HTTP method (or array of methods), an optional _route_, and one or more handler functions. Like the convenience methods above, handler functions accept a `REQUEST`, `RESPONSE`, and optional `next` argument.
```javascript
api.METHOD('trace','/users', (req,res) => {
// do something on TRACE
})
api.METHOD(['post','put'],'/users', (req,res) => {
// do something on POST -or- PUT
})
api.METHOD('get','/users',
(req,res,next) => {
// do some middleware
next() // continue execution
}),
(req,res) => {
// do something
}
)
```
All `GET` methods have a `HEAD` alias that executes the `GET` request but returns a blank `body`. `GET` requests should be idempotent with no side effects. The `head()` convenience method can be used to set specific paths for `HEAD` requests or to override default `GET` aliasing.
Routes that use the `any()` method or pass `ANY` to `api.METHOD` will respond to all HTTP methods. Routes that specify a specific method (such as `GET` or `POST`), will override the route for that method. For example:
```javascript
api.any('/users', (req, res) => {
res.send('any');
});
api.get('/users', (req, res) => {
res.send('get');
});
```
A `POST` to `/users` will return "any", but a `GET` request would return "get". Please note that routes defined with an `ANY` method will override default `HEAD` aliasing for `GET` routes.
## Returning Responses
Lambda API supports both `callback-style` and `async-await` for returning responses to users. The [RESPONSE](#response) object has several callbacks that will trigger a response (`send()`, `json()`, `html()`, etc.) You can use any of these callbacks from within route functions and middleware to send the response:
```javascript
api.get('/users', (req, res) => {
res.send({ foo: 'bar' });
});
```
You can also `return` data from route functions and middleware. The contents will be sent as the body:
```javascript
api.get('/users', (req, res) => {
return { foo: 'bar' };
});
```
### Async/Await
If you prefer to use `async/await`, you can easily apply this to your route functions.
Using `return`:
```javascript
api.get('/users', async (req, res) => {
let users = await getUsers();
return users;
});
```
Or using callbacks:
```javascript
api.get('/users', async (req, res) => {
let users = await getUsers();
res.send(users);
});
```
### Promises
If you like promises, you can either use a callback like `res.send()` at the end of your promise chain, or you can simply `return` the resolved promise:
```javascript
api.get('/users', (req, res) => {
getUsers().then((users) => {
res.send(users);
});
});
```
OR
```javascript
api.get('/users', (req, res) => {
return getUsers().then((users) => {
return users;
});
});
```
**IMPORTANT:** You must either use a callback like `res.send()` **OR** `return` a value. Otherwise the execution will hang and no data will be sent to the user. Also, be sure not to return `undefined`, otherwise it will assume no response.
### A Note About Flow Control
While callbacks like `res.send()` and `res.error()` will trigger a response, they will not necessarily terminate execution of the current route function. Take a look at the following example:
```javascript
api.get('/users', (req, res) => {
if (req.headers.test === 'test') {
res.error('Throw an error');
}
return { foo: 'bar' };
});
```
The example above would not have the intended result of displaying an error. `res.error()` would signal Lambda API to execute the error handling, but the function would continue to run. This would cause the function to `return` a response that would override the intended error. In this situation, you could either wrap the return in an `else` clause, or a cleaner approach would be to `return` the call to the `error()` method, like so:
```javascript
api.get('/users', (req, res) => {
if (req.headers.test === 'test') {
return res.error('Throw an error');
}
return { foo: 'bar' };
});
```
`res.error()` does not have a return value (meaning it is `undefined`). However, the `return` tells the function to stop executing, and the call to `res.error()` handles and formats the appropriate response. This will allow Lambda API to properly return the expected results.
## Route Prefixing
Lambda API makes it easy to create multiple versions of the same api without changing routes by hand. The `register()` method allows you to load routes from an external file and prefix all of those routes using the `prefix` option. For example:
```javascript
// handler.js
const api = require('lambda-api')();
api.register(require('./routes/v1/products'), { prefix: '/v1' });
api.register(require('./routes/v2/products'), { prefix: '/v2' });
module.exports.handler = (event, context, callback) => {
api.run(event, context, callback);
};
```
```javascript
// routes/v1/products.js
module.exports = (api, opts) => {
api.get('/product', handler_v1);
};
```
```javascript
// routes/v2/products.js
module.exports = (api, opts) => {
api.get('/product', handler_v2);
};
```
Even though both modules create a `/product` route, Lambda API will add the `prefix` to them, creating two unique routes. Your users can now access:
- `/v1/product`
- `/v2/product`
You can use `register()` as many times as you want AND it is recursive, so if you nest `register()` methods, the routes will build upon each other. For example:
```javascript
module.exports = (api, opts) => {
api.get('/product', handler_v1);
api.register(require('./v2/products.js'), { prefix: '/v2' });
};
```
This would create a `/v1/product` and `/v1/v2/product` route. You can also use `register()` to load routes from an external file without the `prefix`. This will just add routes to your `base` path. **NOTE:** Prefixed routes are built off of your `base` path if one is set. If your `base` was set to `/api`, then the first example above would produce the routes: `/api/v1/product` and `/api/v2/product`.
## Debugging Routes
Lambda API has a `routes()` method that can be called on the main instance that will return an array containing the `METHOD` and full `PATH` of every configured route. This will include base paths and prefixed routes. This is helpful for debugging your routes.
```javascript
const api = require('lambda-api')();
api.get('/', (req, res) => {});
api.post('/test', (req, res) => {});
api.routes(); // => [ [ 'GET', '/' ], [ 'POST', '/test' ] ]
```
You can also log the paths in table form to the console by passing in `true` as the only parameter.
```javascript
const api = require('lambda-api')()
api.get('/', (req,res) => {})
api.post('/test', (req,res) => {})
api.routes(true)
// Outputs to console
╔═══════════╤═════════════════╗
║ METHOD │ ROUTE ║
╟───────────┼─────────────────╢
║ GET │ / ║
╟───────────┼─────────────────╢
║ POST │ /test ║
╚═══════════╧═════════════════╝
```
## REQUEST
The `REQUEST` object contains a parsed and normalized request from API Gateway. It contains the following values by default:
- `app`: A reference to an instance of the app
- `version`: The version set at initialization
- `id`: The awsRequestId from the Lambda `context`
- `interface`: The interface being used to access Lambda (`apigateway`,`alb`, or `edge`)
- `params`: Dynamic path parameters parsed from the path (see [path parameters](#path-parameters))
- `method`: The HTTP method of the request
- `path`: The path passed in by the request including the `base` and any `prefix` assigned to routes
- `query`: Querystring parameters parsed into an object
- `multiValueQuery`: Querystring parameters with multiple values parsed into an object with array values
- `headers`: An object containing the request headers (properties converted to lowercase for HTTP/2, see [rfc7540 8.1.2. HTTP Header Fields](https://tools.ietf.org/html/rfc7540)). Note that multi-value headers are concatenated with a comma per [rfc2616 4.2. Message Headers](https://www.w3.org/Protocols/rfc2616/rfc2616-sec4.html#sec4.2).
- `rawHeaders`: An object containing the original request headers (property case preserved)
- `multiValueHeaders`: An object containing header values as multi-value arrays
- `body`: The body of the request. If the `isBase64Encoded` flag is `true`, it will be decoded automatically.
- If the `content-type` header is `application/json`, it will attempt to parse the request using `JSON.parse()`
- If the `content-type` header is `application/x-www-form-urlencoded`, it will attempt to parse a URL encoded string using `querystring`
- Otherwise it will be plain text.
- `rawBody`: If the `isBase64Encoded` flag is `true`, this is a copy of the original, base64 encoded body
- `route`: The matched route of the request
- `requestContext`: The `requestContext` passed from the API Gateway
- `pathParameters`: The `pathParameters` passed from the API Gateway
- `stageVariables`: The `stageVariables` passed from the API Gateway
- `isBase64Encoded`: The `isBase64Encoded` boolean passed from the API Gateway
- `auth`: An object containing the `type` and `value` of an authorization header. Currently supports `Bearer`, `Basic`, `OAuth`, and `Digest` schemas. For the `Basic` schema, the object is extended with additional fields for username/password. For the `OAuth` schema, the object is extended with key/value pairs of the supplied OAuth 1.0 values.
- `namespace` or `ns`: A reference to modules added to the app's namespace (see [namespaces](#namespaces))
- `cookies`: An object containing cookies sent from the browser (see the [cookie](#cookiename-value-options) `RESPONSE` method)
- `context`: Reference to the `context` passed into the Lambda handler function
- `coldStart`: Boolean that indicates whether or not the current invocation was a cold start
- `requestCount`: Integer representing the total number of invocations of the current function container (how many times it has been reused)
- `ip`: The IP address of the client making the request
- `userAgent`: The `User-Agent` header sent by the client making the request
- `clientType`: Either `desktop`, `mobile`, `tv`, `tablet` or `unknown` based on CloudFront's analysis of the `User-Agent` header
- `clientCountry`: Two letter country code representing the origin of the requests as determined by CloudFront
- `stack`: An array of function names executed as part of a route's [Execution Stack](#execution-stack), which is useful for debugging
The request object can be used to pass additional information through the processing chain. For example, if you are using a piece of authentication middleware, you can add additional keys to the `REQUEST` object with information about the user. See [middleware](#middleware) for more information.
## RESPONSE
The `RESPONSE` object is used to send a response back to the API Gateway. The `RESPONSE` object contains several methods to manipulate responses. All methods are chainable unless they trigger a response.
### status(code)
The `status` method allows you to set the status code that is returned to API Gateway. By default this will be set to `200` for normal requests or `500` on a thrown error. Additional built-in errors such as `404 Not Found` and `405 Method Not Allowed` may also be returned. The `status()` method accepts a single integer argument.
```javascript
api.get('/users', (req, res) => {
res.status(304).send('Not Modified');
});
```
### sendStatus(code)
The `sendStatus` method sets the status code and returns its string representation as the response body. The `sendStatus()` method accepts a single integer argument.
```javascript
res.sendStatus(200); // equivalent to res.status(200).send('OK')
res.sendStatus(304); // equivalent to res.status(304).send('Not Modified')
res.sendStatus(403); // equivalent to res.status(403).send('Forbidden')
```
**NOTE:** If an unsupported status code is provided, it will return 'Unknown' as the body.
### header(key, value [,append])
The `header` method allows for you to set additional headers to return to the client. By default, just the `content-type` header is sent with `application/json` as the value. Headers can be added or overwritten by calling the `header()` method with two string arguments. The first is the name of the header and then second is the value. You can utilize multi-value headers by specifying an array with multiple values as the `value`, or you can use an optional third boolean parameter and append multiple headers.
```javascript
api.get('/users', (req, res) => {
res.header('content-type', 'text/html').send('<div>This is HTML</div>');
});
// Set multiple header values
api.get('/users', (req, res) => {
res.header('someHeader', ['foo', 'bar']).send({});
});
// Set multiple header by adding to existing header
api.get('/users', (req, res) => {
res
.header('someHeader', 'foo')
.header('someHeader', 'bar', true) // append another value
.send({});
});
```
**NOTE:** Header keys are converted and stored as lowercase in compliance with [rfc7540 8.1.2. HTTP Header Fields](https://tools.ietf.org/html/rfc7540) for HTTP/2. Header convenience methods (`getHeader`, `hasHeader`, and `removeHeader`) automatically ignore case.
### getHeader(key [,asArray])
Retrieve a specific header value. `key` is case insensitive. By default (and for backwards compatibility), header values are returned as a `string`. Multi-value headers will be concatenated using a comma (see [rfc2616 4.2. Message Headers](https://www.w3.org/Protocols/rfc2616/rfc2616-sec4.html#sec4.2)). An optional second boolean parameter can be passed to return header values as an `array`.
**NOTE:** The ability to retrieve the current header object by calling `getHeader()` is still possible, but the preferred method is to use the `getHeaders()` method. By default, `getHeader()` will return the object with `string` values.
### getHeaders()
Retrieve the current header object. Values are returned as `array`s.
### hasHeader(key)
Returns a boolean indicating the existence of `key` in the response headers. `key` is case insensitive.
### removeHeader(key)
Removes header matching `key` from the response headers. `key` is case insensitive. This method is chainable.
### getLink(s3Path [, expires] [, callback])
This returns a signed URL to the referenced file in S3 (using the `s3://{my-bucket}/{path-to-file}` format). You can optionally pass in an integer as the second parameter that will changed the default expiration time of the link. The expiration time is in seconds and defaults to `900`. In order to ensure proper URL signing, the `getLink()` must be asynchronous, and therefore returns a promise. You must either `await` the result or use a `.then` to retrieve the value.
There is an optional third parameter that takes an error handler callback. If the underlying `getSignedUrl()` call fails, the error will be returned using the standard `res.error()` method. You can override this by providing your own callback.
```javascript
// async/await
api.get('/getLink', async (req, res) => {
let url = await res.getLink('s3://my-bucket/my-file.pdf');
return { link: url };
});
// promises
api.get('/getLink', (req, res) => {
res.getLink('s3://my-bucket/my-file.pdf').then((url) => {
res.json({ link: url });
});
});
```
### send(body)
The `send` methods triggers the API to return data to the API Gateway. The `send` method accepts one parameter and sends the contents through as is, e.g. as an object, string, integer, etc. AWS Gateway expects a string, so the data should be converted accordingly.
### json(body)
There is a `json` convenience method for the `send` method that will set the headers to `application/json` as well as perform `JSON.stringify()` on the contents passed to it.
```javascript
api.get('/users', (req, res) => {
res.json({ message: 'This will be converted automatically' });
});
```
### jsonp(body)
There is a `jsonp` convenience method for the `send` method that will set the headers to `application/json`, perform `JSON.stringify()` on the contents passed to it, and wrap the results in a callback function. By default, the callback function is named `callback`.
```javascript
res.jsonp({ foo: 'bar' });
// => callback({ "foo": "bar" })
res.status(500).jsonp({ error: 'some error' });
// => callback({ "error": "some error" })
```
The default can be changed by passing in `callback` as a URL parameter, e.g. `?callback=foo`.
```javascript
// ?callback=foo
res.jsonp({ foo: 'bar' });
// => foo({ "foo": "bar" })
```
You can change the default URL parameter using the optional `callback` option when initializing the API.
```javascript
const api = require('lambda-api')({ callback: 'cb' });
// ?cb=bar
res.jsonp({ foo: 'bar' });
// => bar({ "foo": "bar" })
```
### html(body)
There is also an `html` convenience method for the `send` method that will set the headers to `text/html` and pass through the contents.
```javascript
api.get('/users', (req, res) => {
res.html('<div>This is HTML</div>');
});
```
### type(type)
Sets the `content-type` header for you based on a single `String` input. There are thousands of MIME types, many of which are likely never to be used by your application. Lambda API stores a list of the most popular file types and will automatically set the correct `content-type` based on the input. If the `type` contains the "/" character, then it sets the `content-type` to the value of `type`.
```javascript
res.type('.html'); // => 'text/html'
res.type('html'); // => 'text/html'
res.type('json'); // => 'application/json'
res.type('application/json'); // => 'application/json'
res.type('png'); // => 'image/png'
res.type('.doc'); // => 'application/msword'
res.type('text/css'); // => 'text/css'
```
For a complete list of auto supported types, see [mimemap.js](lib/mimemap.js). Custom MIME types can be added by using the `mimeTypes` option when instantiating Lambda API
### location(path)
The `location` convenience method sets the `Location:` header with the value of a single string argument. The value passed in is not validated but will be encoded before being added to the header. Values that are already encoded can be safely passed in. Note that a valid `3xx` status code must be set to trigger browser redirection. The value can be a relative/absolute path OR a FQDN.
```javascript
api.get('/redirectToHome', (req, res) => {
res.location('/home').status(302).html('<div>Redirect to Home</div>');
});
api.get('/redirectToGithub', (req, res) => {
res
.location('https://github.com')
.status(302)
.html('<div>Redirect to GitHub</div>');
});
```
### redirect([status,] path)
The `redirect` convenience method triggers a redirection and ends the current API execution. This method is similar to the `location()` method, but it automatically sets the status code and calls `send()`. The redirection URL (relative/absolute path, a FQDN, or an S3 path reference) can be specified as the only parameter or as a second parameter when a valid `3xx` status code is supplied as the first parameter. The status code is set to `302` by default, but can be changed to `300`, `301`, `302`, `303`, `307`, or `308` by adding it as the first parameter.
```javascript
api.get('/redirectToHome', (req, res) => {
res.redirect('/home');
});
api.get('/redirectToGithub', (req, res) => {
res.redirect(301, 'https://github.com');
});
// This will redirect a signed URL using the getLink method
api.get('/redirectToS3File', (req, res) => {
res.redirect('s3://my-bucket/someFile.pdf');
});
```
### cors([options])
Convenience method for adding [CORS](https://en.wikipedia.org/wiki/Cross-origin_resource_sharing) headers to responses. An optional `options` object can be passed in to customize the defaults.
The six defined **CORS** headers are as follows:
- Access-Control-Allow-Origin (defaults to `*`)
- Access-Control-Allow-Methods (defaults to `GET, PUT, POST, DELETE, OPTIONS`)
- Access-Control-Allow-Headers (defaults to `Content-Type, Authorization, Content-Length, X-Requested-With`)
- Access-Control-Expose-Headers
- Access-Control-Max-Age
- Access-Control-Allow-Credentials
The `options` object can contain the following properties that correspond to the above headers:
- origin _(string)_
- methods _(string)_
- headers _(string)_
- exposeHeaders _(string)_
- maxAge _(number in milliseconds)_
- credentials _(boolean)_
Defaults can be set by calling `res.cors()` with no properties, or with any combination of the above options.
```javascript
res.cors({
origin: 'example.com',
methods: 'GET, POST, OPTIONS',
headers: 'content-type, authorization',
maxAge: 84000000,
});
```
You can override existing values by calling `res.cors()` with just the updated values:
```javascript
res.cors({
origin: 'api.example.com',
});
```
### error([code], message [,detail])
An error can be triggered by calling the `error` method. This will cause the API to stop execution and return the message to the client. The status code can be set by optionally passing in an integer as the first parameter. Additional detail can be added as an optional third parameter (or second parameter if no status code is passed). This will add an additional `detail` property to error logs. Details accepts any value that can be serialized by `JSON.stringify` including objects, strings and arrays. Custom error handling can be accomplished using the [Error Handling](#error-handling) feature.
```javascript
api.get('/users', (req, res) => {
res.error('This is an error');
});
api.get('/users', (req, res) => {
res.error(403, 'Not authorized');
});
api.get('/users', (req, res) => {
res.error('Error', { foo: 'bar' });
});
api.get('/users', (req, res) => {
res.error(404, 'Page not found', 'foo bar');
});
```
### cookie(name, value [,options])
Convenience method for setting cookies. This method accepts a `name`, `value` and an optional `options` object with the following parameters:
| Property | Type | Description |
| -------- | --------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| domain | `String` | Domain name to use for the cookie. This defaults to the current domain. |
| expires | `Date` | The expiration date of the cookie. Local dates will be converted to GMT. Creates session cookie if this value is not specified. |
| httpOnly | `Boolean` | Sets the cookie to be accessible only via a web server, not JavaScript. |
| maxAge | `Number` | Set the expiration time relative to the current time in milliseconds. Automatically sets the `expires` property if not explicitly provided. |
| path | `String` | Path for the cookie. Defaults to "/" for the root directory. |
| secure | `Boolean` | Sets the cookie to be used with HTTPS only. |
| sameSite | `Boolean` or `String` | Sets the SameSite value for cookie. `true` or `false` sets `Strict` or `Lax` respectively. Also allows a string value. See https://tools.ietf.org/html/draft-ietf-httpbis-cookie-same-site-00#section-4.1.1 |
The `name` attribute should be a string (auto-converted if not), but the `value` attribute can be any type of value. The `value` will be serialized (if an object, array, etc.) and then encoded using `encodeURIComponent` for safely assigning the cookie value. Cookies are automatically parsed, decoded, and available via the `REQUEST` object (see [REQUEST](#request)).
**NOTE:** The `cookie()` method only sets the header. A execution ending method like `send()`, `json()`, etc. must be called to send the response.
```javascript
res.cookie('foo', 'bar', { maxAge: 3600 * 1000, secure: true }).send();
res
.cookie(
'fooObject',
{ foo: 'bar' },
{ domain: '.test.com', path: '/admin', httpOnly: true }
)
.send();
res
.cookie('fooArray', ['one', 'two', 'three'], { path: '/', httpOnly: true })
.send();
```
### clearCookie(name [,options])
Convenience method for expiring cookies. Requires the `name` and optional `options` object as specified in the [cookie](#cookiename-value-options) method. This method will automatically set the expiration time. However, most browsers require the same options to clear a cookie as was used to set it. E.g. if you set the `path` to "/admin" when you set the cookie, you must use this same value to clear it.
```javascript
res.clearCookie('foo', { secure: true }).send();
res
.clearCookie('fooObject', {
domain: '.test.com',
path: '/admin',
httpOnly: true,
})
.send();
res.clearCookie('fooArray', { path: '/', httpOnly: true }).send();
```
**NOTE:** The `clearCookie()` method only sets the header. A execution ending method like `send()`, `json()`, etc. must be called to send the response.
### etag([boolean])
Enables Etag generation for the response if at value of `true` is passed in. Lambda API will generate an Etag based on the body of the response and return the appropriate header. If the request contains an `If-No-Match` header that matches the generated Etag, a `304 Not Modified` response will be returned with a blank body.
### cache([age] [, private])
Adds `cache-control` header to responses. If the first parameter is an `integer`, it will add a `max-age` to the header. The number should be in milliseconds. If the first parameter is `true`, it will add the cache headers with `max-age` set to `0` and use the current time for the `expires` header. If set to false, it will add a cache header with `no-cache, no-store, must-revalidate` as the value. You can also provide a custom string that will manually set the value of the `cache-control` header. And optional second argument takes a `boolean` and will set the `cache-control` to `private` This method is chainable.
```javascript
res.cache(false).send(); // 'cache-control': 'no-cache, no-store, must-revalidate'
res.cache(1000).send(); // 'cache-control': 'max-age=1'
res.cache(30000, true).send(); // 'cache-control': 'private, max-age=30'
```
### modified(date)
Adds a `last-modified` header to responses. A value of `true` will set the value to the current date and time. A JavaScript `Date` object can also be passed in. Note that it will be converted to UTC if not already. A `string` can also be passed in and will be converted to a date if JavaScript's `Date()` function is able to parse it. A value of `false` will prevent the header from being generated, but will not remove any existing `last-modified` headers.
### attachment([filename])
Sets the HTTP response `content-disposition` header field to "attachment". If a `filename` is provided, then the `content-type` is set based on the file extension using the `type()` method and the "filename=" parameter is added to the `content-disposition` header.
```javascript
res.attachment();
// content-disposition: attachment
res.attachment('path/to/logo.png');
// content-disposition: attachment; filename="logo.png"
// content-type: image/png
```
### download(file [, filename] [, options] [, callback])
This transfers the `file` (either a local path, S3 file reference, or Javascript `Buffer`) as an "attachment". This is a convenience method that combines `attachment()` and `sendFile()` to prompt the user to download the file. This method optionally takes a `filename` as a second parameter that will overwrite the "filename=" parameter of the `content-disposition` header, otherwise it will use the filename from the `file`. An optional `options` object passes through to the [sendFile()](#sendfilefile--options--callback) method and takes the same parameters. Finally, a optional `callback` method can be defined which is passed through to [sendFile()](#sendfilefile--options--callback) as well.
```javascript
res.download('./files/sales-report.pdf')
res.download('./files/sales-report.pdf', 'report.pdf')
res.download('s3://my-bucket/path/to/file.png', 'logo.png', { maxAge: 3600000 })
res.download(<Buffer>, 'my-file.docx', { maxAge: 3600000 }, (err) => {
if (err) {
res.error('Custom File Error')
}
})
```
### sendFile(file [, options] [, callback])
The `sendFile()` method takes up to three arguments. The first is the `file`. This is either a local filename (stored within your uploaded lambda code), a reference to a file in S3 (using the `s3://{my-bucket}/{path-to-file}` format), or a JavaScript `Buffer`. You can optionally pass an `options` object using the properties below as well as a callback function `callback(err)` that can handle custom errors or manipulate the response before sending to the client.
| Property | Type | Description | Default |
| ------------ | --------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | ------- |
| maxAge | `Number` | Set the expiration time relative to the current time in milliseconds. Automatically sets the `Expires` header | 0 |
| root | `String` | Root directory for relative filenames. | |
| lastModified | `Boolean` or `String` | Sets the `last-modified` header to the last modified date of the file. This can be disabled by setting it to `false`, or overridden by setting it to a valid `Date` object | |
| headers | `Object` | Key value pairs of additional headers to be sent with the file | |
| cacheControl | `Boolean` or `String` | Enable or disable setting `cache-control` response header. Override value with custom string. | true |
| private | `Boolean` | Sets the `cache-control` to `private`. | false |
```javascript
res.sendFile('./img/logo.png')
res.sendFile('./img/logo.png', { maxAge: 3600000 })
res.sendFile('s3://my-bucket/path/to/file.png', { maxAge: 3600000 })
res.sendFile(<Buffer>, 'my-file.docx', { maxAge: 3600000 }, (err) => {
if (err) {
res.error('Custom File Error')
}
})
```
The `callback` function supports returning a promise, allowing you to perform additional tasks _after_ the file is successfully loaded from the source. This can be used to perform additional synchronous tasks before returning control to the API execution.
**NOTE:** In order to access S3 files, your Lambda function must have `GetObject` access to the files you're attempting to access.
See [Enabling Binary Support](#enabling-binary-support) for more information.
## Enabling Binary Support
To enable binary support, you need to add `*/*` under "Binary Media Types" in **API Gateway** -> **APIs** -> **[ your api ]** -> **Settings**. This will also `base64` encode all body content, but Lambda API will automatically decode it for you.

_Add_ `*/*` _to Binary Media Types_
## Path Parameters
Path parameters are extracted from the path sent in by API Gateway. Although API Gateway supports path parameters, the API doesn't use these values but insteads extracts them from the actual path. This gives you more flexibility with the API Gateway configuration. Path parameters are defined in routes using a colon `:` as a prefix.
```javascript
api.get('/users/:userId', (req, res) => {
res.send('User ID: ' + req.params.userId);
});
```
Path parameters act as wildcards that capture the value into the `params` object. The example above would match `/users/123` and `/users/test`. The system always looks for static paths first, so if you defined paths for `/users/test` and `/users/:userId`, exact path matches would take precedence. Path parameters only match the part of the path they are defined on. E.g. `/users/456/test` would not match `/users/:userId`. You would either need to define `/users/:userId/test` as its own path, or create another path with an additional path parameter, e.g. `/users/:userId/:anotherParam`.
A path can contain as many parameters as you want. E.g. `/users/:param1/:param2/:param3`.
## Wildcard Routes
Wildcard routes are supported for matching arbitrary paths. Wildcards only work at the _end of a route definition_ such as `/*` or `/users/*`. Wildcards within a path, e.g. `/users/*/posts` are not supported. Wildcard routes do support parameters, however, so `/users/:id/*` would capture the `:id` parameter in your wildcard handler.
Wildcard routes will match any deep paths after the wildcard. For example, a `GET` method for path `/users/*` would match `/users/1/posts/latest`. The only exception is for the `OPTIONS` method. A path **must** exist for a wildcard on an `OPTIONS` route in order to execute the handler. If a wildcard route is defined for another method higher up the path, then the `OPTIONS` handler will fire. For example, if there was a `POST` method defined on `/users/*`, then an `OPTIONS` method for `/users/2/posts/*` would fire as it assumes that the `POST` path would exist.
In most cases, [Path Parameters](#path-parameters) should be used in favor of wildcard routes. However, if you need to support unpredictable path lengths, or your are building single purpose functions and will be mapping routes from API Gateway, the wildcards are a powerful pattern. Another good use case is to use the `OPTIONS` method to provide CORS headers.
```javascript
api.options('/*', (req, res) => {
// Return CORS headers
res.cors().send({});
});
```
## Logging
Lambda API includes a robust logging engine specifically designed to utilize native JSON support for CloudWatch Logs. Not only is it ridiculously fast, but it's also highly configurable. Logging is disabled by default, but can be enabled by passing `{ logger: true }` when you create the Lambda API instance (or by passing a [Logging Configuration](#logging-configuration) definition).
The logger is attached to the `REQUEST` object and can be used anywhere the object is available (e.g. routes, middleware, and error handlers).
```javascript
const api = require('lambda-api')({ logger: true });
api.get('/status', (req, res) => {
req.log.info('Some info about this route');
res.send({ status: 'ok' });
});
```
In addition to manual logging, Lambda API can also generate "access" logs for your API requests. API Gateway can also provide access logs, but they are limited to contextual information about your request (see [here](https://docs.aws.amazon.com/apigateway/latest/developerguide/api-gateway-mapping-template-reference.html)). Lambda API allows you to capture the same data *