keydown-key
Version:
An utility to normalize the KeyboardEvent.key especially during IME
114 lines (73 loc) • 2.95 kB
Markdown
[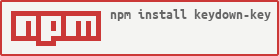](https://www.npmjs.com/package/keydown-key/)
An utility to normalize the KeyboardEvent.key especially during IME composition
To address the inconsistent behavior of [IME](https://en.wikipedia.org/wiki/Input_method) across different browsers and platforms:
When selecting a [CJK character](https://en.wikipedia.org/wiki/CJK_characters) using the Enter key with IME, the `keyDownEvent.key` value varies depending on the platform and browser combination.
**Example:** [Screenshot](https://imgur.com/63EJixc)
- **Chrome:**
- **Mac:** `key === "Enter"`
- **Windows:** `key === "Process"`
- **Firefox:**
- `key === "Process"` on both **Mac** and **Windows**
This discrepancy can cause inconsistencies when handling keyboard events in web applications.
- [Vanilla JS] https://codepen.io/seawind543/pen/gOWNVYR
- [React JS] https://codepen.io/seawind543/pen/xxdvZyE
1. Install the latest version of [keydown-key](https://github.com/seawind543/keydown-key):
```sh
yarn add keydown-key
```
2. Apply `keydown-key` in your application
```javascript
import keyDownKey from 'keydown-key';
// ... omit
function handleKeyDown(event: KeyboardEvent) {
const { key } = keyDownKey(event);
switch(key) {
case 'Enter':
// Do what you want for real `Enter` key
break;
case 'Process':
// The keyDown on "Enter" with IME will be here
break;
default:
}
}
inputBox.addEventListener('keydown', handleKeyDown);
```
```javascript
import React from "react";
import keydownKey from "keydown-key";
const handleKeyDown = (event: React.KeyboardEvent) => {
// use the `nativeEvent` attribute to get the browser KeyboardEvent
// https://reactjs.org/docs/events.html#overview
const { key } = keydownKey(event.nativeEvent);
switch(key) {
case 'Enter':
// Do what you want for real `Enter` key
break;
case 'Process':
// The keyDown on "Enter" with IME will be here
break;
default:
}
};
const App = () => {
return <input onKeyDown={handleKeyDown} />;
};
export default App;
```
[ ] IME https://en.wikipedia.org/wiki/Input_method
[ ] CJK characters https://en.wikipedia.org/wiki/CJK_characters
[ ] add support for SyntheticKeyboardEvent
[ ] Support IME https://github.com/seawind543/react-token-input/issues/1#issuecomment-896190656
[ ] Element: keydown event https://developer.mozilla.org/en-US/docs/Web/API/Document/keydown_event#ignoring_keydown_during_ime_composition
[ ](./LICENSE)