bulmil
Version:
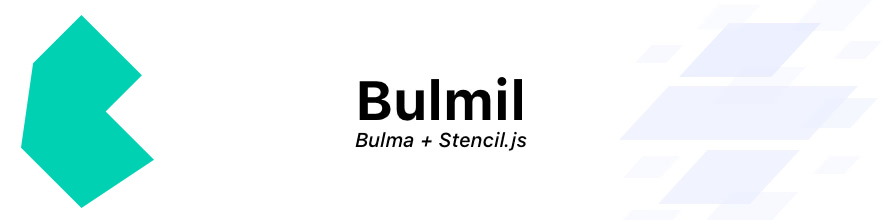
1,906 lines (1,905 loc) • 47.3 kB
TypeScript
/* eslint-disable */
/* tslint:disable */
/**
* This is an autogenerated file created by the Stencil compiler.
* It contains typing information for all components that exist in this project.
*/
import { HTMLStencilElement, JSXBase } from "./stencil-public-runtime";
export namespace Components {
interface BmBadge {
/**
* Color
*/
"color": | 'is-primary'
| 'is-info'
| 'is-success'
| 'is-danger'
| 'is-warning'
| 'is-white'
| 'is-dark'
| 'is-black';
/**
* Is light
*/
"isLight": boolean;
/**
* Is outlined
*/
"isOutlined": boolean;
/**
* Position
*/
"position": | 'is-top-left'
| 'is-top'
| 'is-top-right'
| 'is-right'
| 'is-bottom-right'
| 'is-bottom'
| 'is-bottom-left'
| 'is-left';
}
interface BmBox {
}
interface BmBreadcrumb {
/**
* Breadcrumb alignment
*/
"alignment": 'is-centered' | 'is-right';
/**
* Separator
*/
"separator": | 'has-arrow-separator'
| 'has-bullet-separator'
| 'has-dot-separator'
| 'has-succeeds-separator';
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmButton {
/**
* Color
*/
"color": | 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-danger'
| 'is-warning'
| 'is-white'
| 'is-light'
| 'is-dark'
| 'is-black'
| 'is-text';
/**
* Is disabled?
*/
"disabled": boolean;
/**
* Active state
*/
"isActive": boolean;
/**
* Focused state
*/
"isFocused": boolean;
/**
* Display the button in full-width
*/
"isFullwidth": boolean;
/**
* Hovered state
*/
"isHovered": boolean;
/**
* Is inverted
*/
"isInverted": boolean;
/**
* Is light
*/
"isLight": boolean;
/**
* Loading state
*/
"isLoading": boolean;
/**
* Is outlined
*/
"isOutlined": boolean;
/**
* Rounded button
*/
"isRounded": boolean;
/**
* Static
*/
"isStatic": boolean;
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large' | 'is-normal';
}
interface BmButtons {
/**
* Buttons size
*/
"size": 'are-small' | 'are-medium' | 'are-large';
}
interface BmCard {
}
interface BmCardContent {
}
interface BmCardFooter {
}
interface BmCardHeader {
}
interface BmCardImage {
}
interface BmCheckbox {
/**
* Checked
*/
"checked": boolean;
/**
* Disabled
*/
"disabled": boolean;
/**
* Input classes
*/
"inputClass": string;
/**
* Label classes
*/
"labelClass": string;
}
interface BmColumn {
/**
* Narrow column
*/
"isNarrow": boolean;
/**
* Sizes
*/
"sizes": string;
}
interface BmColumns {
/**
* Columns gaps
*/
"gaps": string;
/**
* Centered
*/
"isCentered": boolean;
/**
* Desktop
*/
"isDesktop": boolean;
/**
* Gapless
*/
"isGapless": boolean;
/**
* Mobile
*/
"isMobile": boolean;
/**
* Multiline
*/
"isMultiline": boolean;
/**
* Vertically centered
*/
"isVcentered": boolean;
}
interface BmContainer {
/**
* Container breakpoint
*/
"breakpoint": 'is-widescreen' | 'is-fullhd';
/**
* Fluid container
*/
"isFluid": boolean;
}
interface BmContent {
/**
* Content size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmDivider {
/**
* CSS Classes
*/
"content": string;
/**
* Orientation
*/
"orientation": 'is-horizontal' | 'is-vertical';
}
interface BmDropdown {
/**
* The dropdown visibility
*/
"isActive": boolean;
/**
* The dropdown will show up when hovering the dropdown-trigger
*/
"isHoverable": boolean;
/**
* Align the dropdown to the right
*/
"isRight": boolean;
/**
* Dropdown menu that appears above the dropdown button
*/
"isUp": boolean;
}
interface BmField {
/**
* Has addons
*/
"hasAddons": boolean;
/**
* Grouped field
*/
"isGrouped": boolean;
/**
* Grouped, on multiline
*/
"isGroupedMultiline": boolean;
/**
* Horizontal field
*/
"isHorizontal": boolean;
/**
* Label
*/
"label": string;
/**
* Help or error message
*/
"message": string;
/**
* Field size
*/
"size": 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
}
interface BmFile {
/**
* Alignment
*/
"alignment": 'is-centered' | 'is-right';
/**
* Color
*/
"color": | 'is-white'
| 'is-black'
| 'is-light'
| 'is-dark'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* File
*/
"file": File;
/**
* Add a placeholder for the selected file name
*/
"hasName": boolean;
/**
* Boxed block
*/
"isBoxed": boolean;
/**
* Expand the name to fill up the space
*/
"isFullwidth": boolean;
/**
* Move the CTA to the right side
*/
"isRight": boolean;
/**
* Name
*/
"name": string;
/**
* Placeholder
*/
"placeholder": string;
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmFooter {
}
interface BmIcon {
/**
* Color
*/
"color": 'has-text-info' | 'has-text-success' | 'has-text-warning' | 'has-text-danger';
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmImage {
/**
* Size
*/
"size": | 'is-16x16'
| 'is-24x24'
| 'is-32x32'
| 'is-48x48'
| 'is-64x64'
| 'is-96x96'
| 'is-128x128';
}
interface BmInput {
/**
* Color
*/
"color": 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control Classes
*/
"controlClass": string;
/**
* Disabled state
*/
"disabled": boolean;
/**
* Loading state
*/
"isLoading": boolean;
/**
* Rounded
*/
"isRounded": boolean;
/**
* Removes the background, border, shadow, and horizontal padding
*/
"isStatic": boolean;
/**
* Name
*/
"name": string;
/**
* Placeholder
*/
"placeholder": string;
/**
* The input will look similar to a normal one, but is not editable and has no shadow
*/
"readonly": boolean;
/**
* Required
*/
"required": boolean;
/**
* Size
*/
"size": 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state": 'is-hovered' | 'is-focused';
/**
* Type
*/
"type": 'text' | 'number' | 'email' | 'password';
/**
* Value
*/
"value": string | number;
}
interface BmMenu {
}
interface BmMessage {
/**
* Color
*/
"color": | 'is-dark'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmModal {
/**
* Modal Card
*/
"hasModalCard": boolean;
/**
* Is Active
*/
"isActive": boolean;
}
interface BmNavbar {
/**
* Color
*/
"color": | 'is-black'
| 'is-dark'
| 'is-light'
| 'is-white'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Fixed position
*/
"fixedPosition": 'is-fixed-top' | 'is-fixed-bottom';
/**
* Spaced
*/
"isSpaced": boolean;
/**
* Transparent
*/
"isTransparent": boolean;
}
interface BmNotification {
/**
* Color
*/
"color": 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Dismissable
*/
"dismissable": boolean;
}
interface BmPagination {
/**
* Alignment
*/
"alignment": 'is-centered' | 'is-right';
/**
* Rounded
*/
"isRounded": boolean;
/**
* Pagination size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmPanel {
/**
* Panel color
*/
"color": 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-danger' | 'is-warning';
}
interface BmProgress {
/**
* Color
*/
"color": 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Maximum value
*/
"max": number;
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
/**
* Value
*/
"value": number;
}
interface BmRadio {
/**
* Checked
*/
"checked": boolean;
/**
* Disabled
*/
"disabled": boolean;
/**
* Input class
*/
"inputClass": string;
/**
* Label Classes
*/
"labelClass": string;
/**
* Name
*/
"name": string;
}
interface BmSection {
/**
* Section size
*/
"size": 'is-medium' | 'is-large';
}
interface BmSelect {
/**
* Color
*/
"color": 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control classes
*/
"controlClass": string;
/**
* Icon
*/
"icon": string;
/**
* Loading state
*/
"isLoading": boolean;
/**
* Allows multiple selection
*/
"isMultiple": boolean;
/**
* Rounded
*/
"isRounded": boolean;
/**
* Size
*/
"size": 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state": 'is-hovered' | 'is-focused';
/**
* Value
*/
"value": string | number;
}
interface BmSlider {
/**
* Color
*/
"color": 'is-success' | 'is-warning' | 'is-danger' | 'is-info';
/**
* Disabled
*/
"disabled": boolean;
/**
* Circle
*/
"isCircle": boolean;
/**
* CSS Classes
*/
"max": number;
/**
* Min
*/
"min": number;
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
/**
* Step
*/
"step": number;
/**
* Value
*/
"value": number;
}
interface BmSwitch {
/**
* Checked
*/
"checked": boolean;
/**
* Color
*/
"color": 'is-success' | 'is-warning' | 'is-danger' | 'is-info';
/**
* Disabled
*/
"disabled": boolean;
/**
* Outlined
*/
"isOutlined": boolean;
/**
* Rounded
*/
"isRounded": boolean;
/**
* Thin
*/
"isThin": boolean;
/**
* Size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
}
interface BmTable {
/**
* Bordered
*/
"isBordered": boolean;
/**
* Fullwidth
*/
"isFullwidth": boolean;
/**
* Hoverable
*/
"isHoverable": boolean;
/**
* Narrow
*/
"isNarrow": boolean;
/**
* Scrollable
*/
"isScrollable": boolean;
/**
* Striped
*/
"isStriped": boolean;
}
interface BmTabs {
/**
* Alignment
*/
"alignment": 'is-centered' | 'is-right';
/**
* Full width
*/
"isFullwidth": boolean;
/**
* Rounded
*/
"isRounded": boolean;
/**
* Pagination size
*/
"size": 'is-small' | 'is-medium' | 'is-large';
/**
* Style
*/
"tabStyle": 'is-boxed' | 'is-toggle';
}
interface BmTag {
/**
* Color
*/
"color": | 'is-black'
| 'is-dark'
| 'is-light'
| 'is-white'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Modifier
*/
"modifier": 'is-rounded' | 'is-delete';
/**
* Size
*/
"size": 'is-normal' | 'is-medium' | 'is-large';
/**
* Tag
*/
"tag": string;
}
interface BmTags {
/**
* Has addons
*/
"hasAddons": boolean;
/**
* Size
*/
"size": 'are-medium' | 'are-large';
}
interface BmTextarea {
/**
* Color
*/
"color": 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control class
*/
"controlClass": string;
/**
* Disabled state
*/
"disabled": boolean;
/**
* Fixed size
*/
"hasFixedSize": boolean;
/**
* Loading state
*/
"isLoading": boolean;
/**
* Readonly state
*/
"readonly": boolean;
/**
* Rows
*/
"rows": number;
/**
* Size
*/
"size": 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state": 'is-hovered' | 'is-focused';
/**
* Value
*/
"value": string | number;
}
}
declare global {
interface HTMLBmBadgeElement extends Components.BmBadge, HTMLStencilElement {
}
var HTMLBmBadgeElement: {
prototype: HTMLBmBadgeElement;
new (): HTMLBmBadgeElement;
};
interface HTMLBmBoxElement extends Components.BmBox, HTMLStencilElement {
}
var HTMLBmBoxElement: {
prototype: HTMLBmBoxElement;
new (): HTMLBmBoxElement;
};
interface HTMLBmBreadcrumbElement extends Components.BmBreadcrumb, HTMLStencilElement {
}
var HTMLBmBreadcrumbElement: {
prototype: HTMLBmBreadcrumbElement;
new (): HTMLBmBreadcrumbElement;
};
interface HTMLBmButtonElement extends Components.BmButton, HTMLStencilElement {
}
var HTMLBmButtonElement: {
prototype: HTMLBmButtonElement;
new (): HTMLBmButtonElement;
};
interface HTMLBmButtonsElement extends Components.BmButtons, HTMLStencilElement {
}
var HTMLBmButtonsElement: {
prototype: HTMLBmButtonsElement;
new (): HTMLBmButtonsElement;
};
interface HTMLBmCardElement extends Components.BmCard, HTMLStencilElement {
}
var HTMLBmCardElement: {
prototype: HTMLBmCardElement;
new (): HTMLBmCardElement;
};
interface HTMLBmCardContentElement extends Components.BmCardContent, HTMLStencilElement {
}
var HTMLBmCardContentElement: {
prototype: HTMLBmCardContentElement;
new (): HTMLBmCardContentElement;
};
interface HTMLBmCardFooterElement extends Components.BmCardFooter, HTMLStencilElement {
}
var HTMLBmCardFooterElement: {
prototype: HTMLBmCardFooterElement;
new (): HTMLBmCardFooterElement;
};
interface HTMLBmCardHeaderElement extends Components.BmCardHeader, HTMLStencilElement {
}
var HTMLBmCardHeaderElement: {
prototype: HTMLBmCardHeaderElement;
new (): HTMLBmCardHeaderElement;
};
interface HTMLBmCardImageElement extends Components.BmCardImage, HTMLStencilElement {
}
var HTMLBmCardImageElement: {
prototype: HTMLBmCardImageElement;
new (): HTMLBmCardImageElement;
};
interface HTMLBmCheckboxElement extends Components.BmCheckbox, HTMLStencilElement {
}
var HTMLBmCheckboxElement: {
prototype: HTMLBmCheckboxElement;
new (): HTMLBmCheckboxElement;
};
interface HTMLBmColumnElement extends Components.BmColumn, HTMLStencilElement {
}
var HTMLBmColumnElement: {
prototype: HTMLBmColumnElement;
new (): HTMLBmColumnElement;
};
interface HTMLBmColumnsElement extends Components.BmColumns, HTMLStencilElement {
}
var HTMLBmColumnsElement: {
prototype: HTMLBmColumnsElement;
new (): HTMLBmColumnsElement;
};
interface HTMLBmContainerElement extends Components.BmContainer, HTMLStencilElement {
}
var HTMLBmContainerElement: {
prototype: HTMLBmContainerElement;
new (): HTMLBmContainerElement;
};
interface HTMLBmContentElement extends Components.BmContent, HTMLStencilElement {
}
var HTMLBmContentElement: {
prototype: HTMLBmContentElement;
new (): HTMLBmContentElement;
};
interface HTMLBmDividerElement extends Components.BmDivider, HTMLStencilElement {
}
var HTMLBmDividerElement: {
prototype: HTMLBmDividerElement;
new (): HTMLBmDividerElement;
};
interface HTMLBmDropdownElement extends Components.BmDropdown, HTMLStencilElement {
}
var HTMLBmDropdownElement: {
prototype: HTMLBmDropdownElement;
new (): HTMLBmDropdownElement;
};
interface HTMLBmFieldElement extends Components.BmField, HTMLStencilElement {
}
var HTMLBmFieldElement: {
prototype: HTMLBmFieldElement;
new (): HTMLBmFieldElement;
};
interface HTMLBmFileElement extends Components.BmFile, HTMLStencilElement {
}
var HTMLBmFileElement: {
prototype: HTMLBmFileElement;
new (): HTMLBmFileElement;
};
interface HTMLBmFooterElement extends Components.BmFooter, HTMLStencilElement {
}
var HTMLBmFooterElement: {
prototype: HTMLBmFooterElement;
new (): HTMLBmFooterElement;
};
interface HTMLBmIconElement extends Components.BmIcon, HTMLStencilElement {
}
var HTMLBmIconElement: {
prototype: HTMLBmIconElement;
new (): HTMLBmIconElement;
};
interface HTMLBmImageElement extends Components.BmImage, HTMLStencilElement {
}
var HTMLBmImageElement: {
prototype: HTMLBmImageElement;
new (): HTMLBmImageElement;
};
interface HTMLBmInputElement extends Components.BmInput, HTMLStencilElement {
}
var HTMLBmInputElement: {
prototype: HTMLBmInputElement;
new (): HTMLBmInputElement;
};
interface HTMLBmMenuElement extends Components.BmMenu, HTMLStencilElement {
}
var HTMLBmMenuElement: {
prototype: HTMLBmMenuElement;
new (): HTMLBmMenuElement;
};
interface HTMLBmMessageElement extends Components.BmMessage, HTMLStencilElement {
}
var HTMLBmMessageElement: {
prototype: HTMLBmMessageElement;
new (): HTMLBmMessageElement;
};
interface HTMLBmModalElement extends Components.BmModal, HTMLStencilElement {
}
var HTMLBmModalElement: {
prototype: HTMLBmModalElement;
new (): HTMLBmModalElement;
};
interface HTMLBmNavbarElement extends Components.BmNavbar, HTMLStencilElement {
}
var HTMLBmNavbarElement: {
prototype: HTMLBmNavbarElement;
new (): HTMLBmNavbarElement;
};
interface HTMLBmNotificationElement extends Components.BmNotification, HTMLStencilElement {
}
var HTMLBmNotificationElement: {
prototype: HTMLBmNotificationElement;
new (): HTMLBmNotificationElement;
};
interface HTMLBmPaginationElement extends Components.BmPagination, HTMLStencilElement {
}
var HTMLBmPaginationElement: {
prototype: HTMLBmPaginationElement;
new (): HTMLBmPaginationElement;
};
interface HTMLBmPanelElement extends Components.BmPanel, HTMLStencilElement {
}
var HTMLBmPanelElement: {
prototype: HTMLBmPanelElement;
new (): HTMLBmPanelElement;
};
interface HTMLBmProgressElement extends Components.BmProgress, HTMLStencilElement {
}
var HTMLBmProgressElement: {
prototype: HTMLBmProgressElement;
new (): HTMLBmProgressElement;
};
interface HTMLBmRadioElement extends Components.BmRadio, HTMLStencilElement {
}
var HTMLBmRadioElement: {
prototype: HTMLBmRadioElement;
new (): HTMLBmRadioElement;
};
interface HTMLBmSectionElement extends Components.BmSection, HTMLStencilElement {
}
var HTMLBmSectionElement: {
prototype: HTMLBmSectionElement;
new (): HTMLBmSectionElement;
};
interface HTMLBmSelectElement extends Components.BmSelect, HTMLStencilElement {
}
var HTMLBmSelectElement: {
prototype: HTMLBmSelectElement;
new (): HTMLBmSelectElement;
};
interface HTMLBmSliderElement extends Components.BmSlider, HTMLStencilElement {
}
var HTMLBmSliderElement: {
prototype: HTMLBmSliderElement;
new (): HTMLBmSliderElement;
};
interface HTMLBmSwitchElement extends Components.BmSwitch, HTMLStencilElement {
}
var HTMLBmSwitchElement: {
prototype: HTMLBmSwitchElement;
new (): HTMLBmSwitchElement;
};
interface HTMLBmTableElement extends Components.BmTable, HTMLStencilElement {
}
var HTMLBmTableElement: {
prototype: HTMLBmTableElement;
new (): HTMLBmTableElement;
};
interface HTMLBmTabsElement extends Components.BmTabs, HTMLStencilElement {
}
var HTMLBmTabsElement: {
prototype: HTMLBmTabsElement;
new (): HTMLBmTabsElement;
};
interface HTMLBmTagElement extends Components.BmTag, HTMLStencilElement {
}
var HTMLBmTagElement: {
prototype: HTMLBmTagElement;
new (): HTMLBmTagElement;
};
interface HTMLBmTagsElement extends Components.BmTags, HTMLStencilElement {
}
var HTMLBmTagsElement: {
prototype: HTMLBmTagsElement;
new (): HTMLBmTagsElement;
};
interface HTMLBmTextareaElement extends Components.BmTextarea, HTMLStencilElement {
}
var HTMLBmTextareaElement: {
prototype: HTMLBmTextareaElement;
new (): HTMLBmTextareaElement;
};
interface HTMLElementTagNameMap {
"bm-badge": HTMLBmBadgeElement;
"bm-box": HTMLBmBoxElement;
"bm-breadcrumb": HTMLBmBreadcrumbElement;
"bm-button": HTMLBmButtonElement;
"bm-buttons": HTMLBmButtonsElement;
"bm-card": HTMLBmCardElement;
"bm-card-content": HTMLBmCardContentElement;
"bm-card-footer": HTMLBmCardFooterElement;
"bm-card-header": HTMLBmCardHeaderElement;
"bm-card-image": HTMLBmCardImageElement;
"bm-checkbox": HTMLBmCheckboxElement;
"bm-column": HTMLBmColumnElement;
"bm-columns": HTMLBmColumnsElement;
"bm-container": HTMLBmContainerElement;
"bm-content": HTMLBmContentElement;
"bm-divider": HTMLBmDividerElement;
"bm-dropdown": HTMLBmDropdownElement;
"bm-field": HTMLBmFieldElement;
"bm-file": HTMLBmFileElement;
"bm-footer": HTMLBmFooterElement;
"bm-icon": HTMLBmIconElement;
"bm-image": HTMLBmImageElement;
"bm-input": HTMLBmInputElement;
"bm-menu": HTMLBmMenuElement;
"bm-message": HTMLBmMessageElement;
"bm-modal": HTMLBmModalElement;
"bm-navbar": HTMLBmNavbarElement;
"bm-notification": HTMLBmNotificationElement;
"bm-pagination": HTMLBmPaginationElement;
"bm-panel": HTMLBmPanelElement;
"bm-progress": HTMLBmProgressElement;
"bm-radio": HTMLBmRadioElement;
"bm-section": HTMLBmSectionElement;
"bm-select": HTMLBmSelectElement;
"bm-slider": HTMLBmSliderElement;
"bm-switch": HTMLBmSwitchElement;
"bm-table": HTMLBmTableElement;
"bm-tabs": HTMLBmTabsElement;
"bm-tag": HTMLBmTagElement;
"bm-tags": HTMLBmTagsElement;
"bm-textarea": HTMLBmTextareaElement;
}
}
declare namespace LocalJSX {
interface BmBadge {
/**
* Color
*/
"color"?: | 'is-primary'
| 'is-info'
| 'is-success'
| 'is-danger'
| 'is-warning'
| 'is-white'
| 'is-dark'
| 'is-black';
/**
* Is light
*/
"isLight"?: boolean;
/**
* Is outlined
*/
"isOutlined"?: boolean;
/**
* Position
*/
"position"?: | 'is-top-left'
| 'is-top'
| 'is-top-right'
| 'is-right'
| 'is-bottom-right'
| 'is-bottom'
| 'is-bottom-left'
| 'is-left';
}
interface BmBox {
}
interface BmBreadcrumb {
/**
* Breadcrumb alignment
*/
"alignment"?: 'is-centered' | 'is-right';
/**
* Separator
*/
"separator"?: | 'has-arrow-separator'
| 'has-bullet-separator'
| 'has-dot-separator'
| 'has-succeeds-separator';
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmButton {
/**
* Color
*/
"color"?: | 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-danger'
| 'is-warning'
| 'is-white'
| 'is-light'
| 'is-dark'
| 'is-black'
| 'is-text';
/**
* Is disabled?
*/
"disabled"?: boolean;
/**
* Active state
*/
"isActive"?: boolean;
/**
* Focused state
*/
"isFocused"?: boolean;
/**
* Display the button in full-width
*/
"isFullwidth"?: boolean;
/**
* Hovered state
*/
"isHovered"?: boolean;
/**
* Is inverted
*/
"isInverted"?: boolean;
/**
* Is light
*/
"isLight"?: boolean;
/**
* Loading state
*/
"isLoading"?: boolean;
/**
* Is outlined
*/
"isOutlined"?: boolean;
/**
* Rounded button
*/
"isRounded"?: boolean;
/**
* Static
*/
"isStatic"?: boolean;
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large' | 'is-normal';
}
interface BmButtons {
/**
* Buttons size
*/
"size"?: 'are-small' | 'are-medium' | 'are-large';
}
interface BmCard {
}
interface BmCardContent {
}
interface BmCardFooter {
}
interface BmCardHeader {
}
interface BmCardImage {
}
interface BmCheckbox {
/**
* Checked
*/
"checked"?: boolean;
/**
* Disabled
*/
"disabled"?: boolean;
/**
* Input classes
*/
"inputClass"?: string;
/**
* Label classes
*/
"labelClass"?: string;
}
interface BmColumn {
/**
* Narrow column
*/
"isNarrow"?: boolean;
/**
* Sizes
*/
"sizes"?: string;
}
interface BmColumns {
/**
* Columns gaps
*/
"gaps"?: string;
/**
* Centered
*/
"isCentered"?: boolean;
/**
* Desktop
*/
"isDesktop"?: boolean;
/**
* Gapless
*/
"isGapless"?: boolean;
/**
* Mobile
*/
"isMobile"?: boolean;
/**
* Multiline
*/
"isMultiline"?: boolean;
/**
* Vertically centered
*/
"isVcentered"?: boolean;
}
interface BmContainer {
/**
* Container breakpoint
*/
"breakpoint"?: 'is-widescreen' | 'is-fullhd';
/**
* Fluid container
*/
"isFluid"?: boolean;
}
interface BmContent {
/**
* Content size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmDivider {
/**
* CSS Classes
*/
"content"?: string;
/**
* Orientation
*/
"orientation"?: 'is-horizontal' | 'is-vertical';
}
interface BmDropdown {
/**
* The dropdown visibility
*/
"isActive"?: boolean;
/**
* The dropdown will show up when hovering the dropdown-trigger
*/
"isHoverable"?: boolean;
/**
* Align the dropdown to the right
*/
"isRight"?: boolean;
/**
* Dropdown menu that appears above the dropdown button
*/
"isUp"?: boolean;
}
interface BmField {
/**
* Has addons
*/
"hasAddons"?: boolean;
/**
* Grouped field
*/
"isGrouped"?: boolean;
/**
* Grouped, on multiline
*/
"isGroupedMultiline"?: boolean;
/**
* Horizontal field
*/
"isHorizontal"?: boolean;
/**
* Label
*/
"label"?: string;
/**
* Help or error message
*/
"message"?: string;
/**
* Field size
*/
"size"?: 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
}
interface BmFile {
/**
* Alignment
*/
"alignment"?: 'is-centered' | 'is-right';
/**
* Color
*/
"color"?: | 'is-white'
| 'is-black'
| 'is-light'
| 'is-dark'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* File
*/
"file"?: File;
/**
* Add a placeholder for the selected file name
*/
"hasName"?: boolean;
/**
* Boxed block
*/
"isBoxed"?: boolean;
/**
* Expand the name to fill up the space
*/
"isFullwidth"?: boolean;
/**
* Move the CTA to the right side
*/
"isRight"?: boolean;
/**
* Name
*/
"name"?: string;
/**
* Placeholder
*/
"placeholder"?: string;
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmFooter {
}
interface BmIcon {
/**
* Color
*/
"color"?: 'has-text-info' | 'has-text-success' | 'has-text-warning' | 'has-text-danger';
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmImage {
/**
* Size
*/
"size"?: | 'is-16x16'
| 'is-24x24'
| 'is-32x32'
| 'is-48x48'
| 'is-64x64'
| 'is-96x96'
| 'is-128x128';
}
interface BmInput {
/**
* Color
*/
"color"?: 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control Classes
*/
"controlClass"?: string;
/**
* Disabled state
*/
"disabled"?: boolean;
/**
* Loading state
*/
"isLoading"?: boolean;
/**
* Rounded
*/
"isRounded"?: boolean;
/**
* Removes the background, border, shadow, and horizontal padding
*/
"isStatic"?: boolean;
/**
* Name
*/
"name"?: string;
/**
* Placeholder
*/
"placeholder"?: string;
/**
* The input will look similar to a normal one, but is not editable and has no shadow
*/
"readonly"?: boolean;
/**
* Required
*/
"required"?: boolean;
/**
* Size
*/
"size"?: 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state"?: 'is-hovered' | 'is-focused';
/**
* Type
*/
"type"?: 'text' | 'number' | 'email' | 'password';
/**
* Value
*/
"value"?: string | number;
}
interface BmMenu {
}
interface BmMessage {
/**
* Color
*/
"color"?: | 'is-dark'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmModal {
/**
* Modal Card
*/
"hasModalCard"?: boolean;
/**
* Is Active
*/
"isActive"?: boolean;
}
interface BmNavbar {
/**
* Color
*/
"color"?: | 'is-black'
| 'is-dark'
| 'is-light'
| 'is-white'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Fixed position
*/
"fixedPosition"?: 'is-fixed-top' | 'is-fixed-bottom';
/**
* Spaced
*/
"isSpaced"?: boolean;
/**
* Transparent
*/
"isTransparent"?: boolean;
}
interface BmNotification {
/**
* Color
*/
"color"?: 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Dismissable
*/
"dismissable"?: boolean;
}
interface BmPagination {
/**
* Alignment
*/
"alignment"?: 'is-centered' | 'is-right';
/**
* Rounded
*/
"isRounded"?: boolean;
/**
* Pagination size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmPanel {
/**
* Panel color
*/
"color"?: 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-danger' | 'is-warning';
}
interface BmProgress {
/**
* Color
*/
"color"?: 'is-primary' | 'is-link' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Maximum value
*/
"max"?: number;
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
/**
* Value
*/
"value"?: number;
}
interface BmRadio {
/**
* Checked
*/
"checked"?: boolean;
/**
* Disabled
*/
"disabled"?: boolean;
/**
* Input class
*/
"inputClass"?: string;
/**
* Label Classes
*/
"labelClass"?: string;
/**
* Name
*/
"name"?: string;
}
interface BmSection {
/**
* Section size
*/
"size"?: 'is-medium' | 'is-large';
}
interface BmSelect {
/**
* Color
*/
"color"?: 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control classes
*/
"controlClass"?: string;
/**
* Icon
*/
"icon"?: string;
/**
* Loading state
*/
"isLoading"?: boolean;
/**
* Allows multiple selection
*/
"isMultiple"?: boolean;
/**
* Rounded
*/
"isRounded"?: boolean;
/**
* Size
*/
"size"?: 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state"?: 'is-hovered' | 'is-focused';
/**
* Value
*/
"value"?: string | number;
}
interface BmSlider {
/**
* Color
*/
"color"?: 'is-success' | 'is-warning' | 'is-danger' | 'is-info';
/**
* Disabled
*/
"disabled"?: boolean;
/**
* Circle
*/
"isCircle"?: boolean;
/**
* CSS Classes
*/
"max"?: number;
/**
* Min
*/
"min"?: number;
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
/**
* Step
*/
"step"?: number;
/**
* Value
*/
"value"?: number;
}
interface BmSwitch {
/**
* Checked
*/
"checked"?: boolean;
/**
* Color
*/
"color"?: 'is-success' | 'is-warning' | 'is-danger' | 'is-info';
/**
* Disabled
*/
"disabled"?: boolean;
/**
* Outlined
*/
"isOutlined"?: boolean;
/**
* Rounded
*/
"isRounded"?: boolean;
/**
* Thin
*/
"isThin"?: boolean;
/**
* Size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
}
interface BmTable {
/**
* Bordered
*/
"isBordered"?: boolean;
/**
* Fullwidth
*/
"isFullwidth"?: boolean;
/**
* Hoverable
*/
"isHoverable"?: boolean;
/**
* Narrow
*/
"isNarrow"?: boolean;
/**
* Scrollable
*/
"isScrollable"?: boolean;
/**
* Striped
*/
"isStriped"?: boolean;
}
interface BmTabs {
/**
* Alignment
*/
"alignment"?: 'is-centered' | 'is-right';
/**
* Full width
*/
"isFullwidth"?: boolean;
/**
* Rounded
*/
"isRounded"?: boolean;
/**
* Pagination size
*/
"size"?: 'is-small' | 'is-medium' | 'is-large';
/**
* Style
*/
"tabStyle"?: 'is-boxed' | 'is-toggle';
}
interface BmTag {
/**
* Color
*/
"color"?: | 'is-black'
| 'is-dark'
| 'is-light'
| 'is-white'
| 'is-primary'
| 'is-link'
| 'is-info'
| 'is-success'
| 'is-warning'
| 'is-danger';
/**
* Modifier
*/
"modifier"?: 'is-rounded' | 'is-delete';
/**
* Size
*/
"size"?: 'is-normal' | 'is-medium' | 'is-large';
/**
* Tag
*/
"tag"?: string;
}
interface BmTags {
/**
* Has addons
*/
"hasAddons"?: boolean;
/**
* Size
*/
"size"?: 'are-medium' | 'are-large';
}
interface BmTextarea {
/**
* Color
*/
"color"?: 'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger';
/**
* Control class
*/
"controlClass"?: string;
/**
* Disabled state
*/
"disabled"?: boolean;
/**
* Fixed size
*/
"hasFixedSize"?: boolean;
/**
* Loading state
*/
"isLoading"?: boolean;
/**
* Readonly state
*/
"readonly"?: boolean;
/**
* Rows
*/
"rows"?: number;
/**
* Size
*/
"size"?: 'is-small' | 'is-normal' | 'is-medium' | 'is-large';
/**
* State
*/
"state"?: 'is-hovered' | 'is-focused';
/**
* Value
*/
"value"?: string | number;
}
interface IntrinsicElements {
"bm-badge": BmBadge;
"bm-box": BmBox;
"bm-breadcrumb": BmBreadcrumb;
"bm-button": BmButton;
"bm-buttons": BmButtons;
"bm-card": BmCard;
"bm-card-content": BmCardContent;
"bm-card-footer": BmCardFooter;
"bm-card-header": BmCardHeader;
"bm-card-image": BmCardImage;
"bm-checkbox": BmCheckbox;
"bm-column": BmColumn;
"bm-columns": BmColumns;
"bm-container": BmContainer;
"bm-content": BmContent;
"bm-divider": BmDivider;
"bm-dropdown": BmDropdown;
"bm-field": BmField;
"bm-file": BmFile;
"bm-footer": BmFooter;
"bm-icon": BmIcon;
"bm-image": BmImage;
"bm-input": BmInput;
"bm-menu": BmMenu;
"bm-message": BmMessage;
"bm-modal": BmModal;
"bm-navbar": BmNavbar;
"bm-notification": BmNotification;
"bm-pagination": BmPagination;
"bm-panel": BmPanel;
"bm-progress": BmProgress;
"bm-radio": BmRadio;
"bm-section": BmSection;
"bm-select": BmSelect;
"bm-slider": BmSlider;
"bm-switch": BmSwitch;
"bm-table": BmTable;
"bm-tabs": BmTabs;
"bm-tag": BmTag;
"bm-tags": BmTags;
"bm-textarea": BmTextarea;
}
}
export { LocalJSX as JSX };
declare module "@stencil/core" {
export namespace JSX {
interface IntrinsicElements {
"bm-badge": LocalJSX.BmBadge & JSXBase.HTMLAttributes<HTMLBmBadgeElement>;
"bm-box": LocalJSX.BmBox & JSXBase.HTMLAttributes<HTMLBmBoxElement>;
"bm-breadcrumb": LocalJSX.BmBreadcrumb & JSXBase.HTMLAttributes<HTMLBmBreadcrumbElement>;
"bm-button": LocalJSX.BmButton & JSXBase.HTMLAttributes<HTMLBmButtonElement>;
"bm-buttons": LocalJSX.BmButtons & JSXBase.HTMLAttributes<HTMLBmButtonsElement>;
"bm-card": LocalJSX.BmCard & JSXBase.HTMLAttributes<HTMLBmCardElement>;
"bm-card-content": LocalJSX.BmCardContent & JSXBase.HTMLAttributes<HTMLBmCardContentElement>;
"bm-card-footer": LocalJSX.BmCardFooter & JSXBase.HTMLAttributes<HTMLBmCardFooterElement>;
"bm-card-header": LocalJSX.BmCardHeader & JSXBase.HTMLAttributes<HTMLBmCardHeaderElement>;
"bm-card-image": LocalJSX.BmCardImage & JSXBase.HTMLAttributes<HTMLBmCardImageElement>;
"bm-checkbox": LocalJSX.BmCheckbox & JSXBase.HTMLAttributes<HTMLBmCheckboxElement>;
"bm-column": LocalJSX.BmColumn & JSXBase.HTMLAttributes<HTMLBmColumnElement>;
"bm-columns": LocalJSX.BmColumns & JSXBase.HTMLAttributes<HTMLBmColumnsElement>;
"bm-container": LocalJSX.BmContainer & JSXBase.HTMLAttributes<HTMLBmContainerElement>;
"bm-content": LocalJSX.BmContent & JSXBase.HTMLAttributes<HTMLBmContentElement>;
"bm-divider": LocalJSX.BmDivider & JSXBase.HTMLAttributes<HTMLBmDividerElement>;
"bm-dropdown": LocalJSX.BmDropdown & JSXBase.HTMLAttributes<HTMLBmDropdownElement>;
"bm-field": LocalJSX.BmField & JSXBase.HTMLAttributes<HTMLBmFieldElement>;
"bm-file": LocalJSX.BmFile & JSXBase.HTMLAttributes<HTMLBmFileElement>;
"bm-footer": LocalJSX.BmFooter & JSXBase.HTMLAttributes<HTMLBmFooterElement>;
"bm-icon": LocalJSX.BmIcon & JSXBase.HTMLAttributes<HTMLBmIconElement>;
"bm-image": LocalJSX.BmImage & JSXBase.HTMLAttributes<HTMLBmImageElement>;
"bm-input": LocalJSX.BmInput & JSXBase.HTMLAttributes<HTMLBmInputElement>;
"bm-menu": LocalJSX.BmMenu & JSXBase.HTMLAttributes<HTMLBmMenuElement>;
"bm-message": LocalJSX.BmMessage & JSXBase.HTMLAttributes<HTMLBmMessageElement>;
"bm-modal": LocalJSX.BmModal & JSXBase.HTMLAttributes<HTMLBmModalElement>;
"bm-navbar": LocalJSX.BmNavbar & JSXBase.HTMLAttributes<HTMLBmNavbarElement>;
"bm-notification": LocalJSX.BmNotification & JSXBase.HTMLAttributes<HTMLBmNotificationElement>;
"bm-pagination": LocalJSX.BmPagination & JSXBase.HTMLAttributes<HTMLBmPaginationElement>;
"bm-panel": LocalJSX.BmPanel & JSXBase.HTMLAttributes<HTMLBmPanelElement>;
"bm-progress": LocalJSX.BmProgress & JSXBase.HTMLAttributes<HTMLBmProgressElement>;
"bm-radio": LocalJSX.BmRadio & JSXBase.HTMLAttributes<HTMLBmRadioElement>;
"bm-section": LocalJSX.BmSection & JSXBase.HTMLAttributes<HTMLBmSectionElement>;
"bm-select": LocalJSX.BmSelect & JSXBase.HTMLAttributes<HTMLBmSelectElement>;
"bm-slider": LocalJSX.BmSlider & JSXBase.HTMLAttributes<HTMLBmSliderElement>;
"bm-switch": LocalJSX.BmSwitch & JSXBase.HTMLAttributes<HTMLBmSwitchElement>;
"bm-table": LocalJSX.BmTable & JSXBase.HTMLAttributes<HTMLBmTableElement>;
"bm-tabs": LocalJSX.BmTabs & JSXBase.HTMLAttributes<HTMLBmTabsElement>;
"bm-tag": LocalJSX.BmTag & JSXBase.HTMLAttributes<HTMLBmTagElement>;
"bm-tags": LocalJSX.BmTags & JSXBase.HTMLAttributes<HTMLBmTagsElement>;
"bm-textarea": LocalJSX.BmTextarea & JSXBase.HTMLAttributes<HTMLBmTextareaElement>;
}
}
}