bulmil
Version:
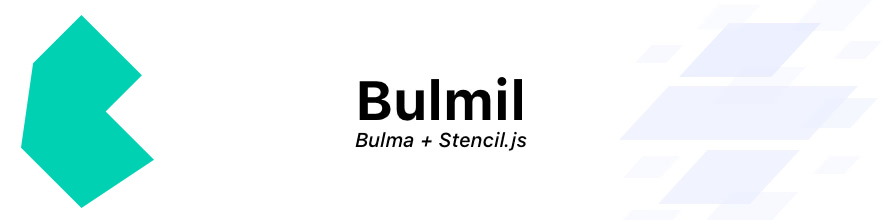
177 lines (176 loc) • 4.91 kB
JavaScript
import { Component, Prop, h, Host } from '@stencil/core';
export class Columns {
constructor() {
/**
* Centered
*/
this.isCentered = false;
/**
* Mobile
*/
this.isMobile = false;
/**
* Desktop
*/
this.isDesktop = false;
/**
* Multiline
*/
this.isMultiline = false;
/**
* Gapless
*/
this.isGapless = false;
/**
* Vertically centered
*/
this.isVcentered = false;
}
render() {
return (h(Host, { class: {
columns: true,
'is-centered': this.isCentered,
'is-mobile': this.isMobile,
'is-desktop': this.isDesktop,
'is-multiline': this.isMultiline,
'is-gapless': this.isGapless,
'is-vcentered': this.isVcentered,
'is-variable': Boolean(this.gaps),
[this.gaps]: Boolean(this.gaps),
} }));
}
static get is() { return "bm-columns"; }
static get originalStyleUrls() { return {
"$": ["columns.scss"]
}; }
static get styleUrls() { return {
"$": ["columns.css"]
}; }
static get properties() { return {
"isCentered": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Centered"
},
"attribute": "is-centered",
"reflect": false,
"defaultValue": "false"
},
"isMobile": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Mobile"
},
"attribute": "is-mobile",
"reflect": false,
"defaultValue": "false"
},
"isDesktop": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Desktop"
},
"attribute": "is-desktop",
"reflect": false,
"defaultValue": "false"
},
"isMultiline": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Multiline"
},
"attribute": "is-multiline",
"reflect": false,
"defaultValue": "false"
},
"isGapless": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Gapless"
},
"attribute": "is-gapless",
"reflect": false,
"defaultValue": "false"
},
"isVcentered": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Vertically centered"
},
"attribute": "is-vcentered",
"reflect": false,
"defaultValue": "false"
},
"gaps": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Columns gaps"
},
"attribute": "gaps",
"reflect": false
}
}; }
}