bulmil
Version:
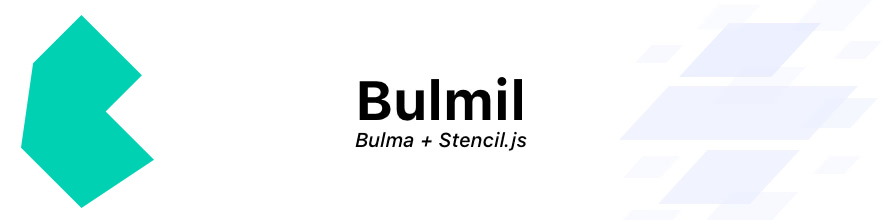
175 lines (174 loc) • 5.02 kB
JavaScript
import { Component, Prop, h, Host } from '@stencil/core';
export class Switch {
constructor() {
/**
* Thin
*/
this.isThin = false;
/**
* Rounded
*/
this.isRounded = false;
/**
* Outlined
*/
this.isOutlined = false;
/**
* Disabled
*/
this.disabled = false;
/**
* Checked
*/
this.checked = false;
this.onClick = () => {
this.checked = !this.checked;
};
}
render() {
return (h(Host, { onClick: this.onClick },
h("input", { type: "checkbox", class: {
switch: true,
[this.color]: Boolean(this.color),
[this.size]: Boolean(this.size),
'is-thin': this.isThin,
'is-rounded': this.isRounded,
'is-outlined': this.isOutlined,
}, checked: this.checked, disabled: this.disabled }),
h("label", null,
h("slot", null))));
}
static get is() { return "bm-switch"; }
static get originalStyleUrls() { return {
"$": ["switch.scss"]
}; }
static get styleUrls() { return {
"$": ["switch.css"]
}; }
static get properties() { return {
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-success' | 'is-warning' | 'is-danger' | 'is-info'",
"resolved": "\"is-danger\" | \"is-info\" | \"is-success\" | \"is-warning\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"size": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-small' | 'is-medium' | 'is-large'",
"resolved": "\"is-large\" | \"is-medium\" | \"is-small\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Size"
},
"attribute": "size",
"reflect": false
},
"isThin": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Thin"
},
"attribute": "is-thin",
"reflect": false,
"defaultValue": "false"
},
"isRounded": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Rounded"
},
"attribute": "is-rounded",
"reflect": false,
"defaultValue": "false"
},
"isOutlined": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Outlined"
},
"attribute": "is-outlined",
"reflect": false,
"defaultValue": "false"
},
"disabled": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Disabled"
},
"attribute": "disabled",
"reflect": false,
"defaultValue": "false"
},
"checked": {
"type": "boolean",
"mutable": true,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Checked"
},
"attribute": "checked",
"reflect": false,
"defaultValue": "false"
}
}; }
}