bulmil
Version:
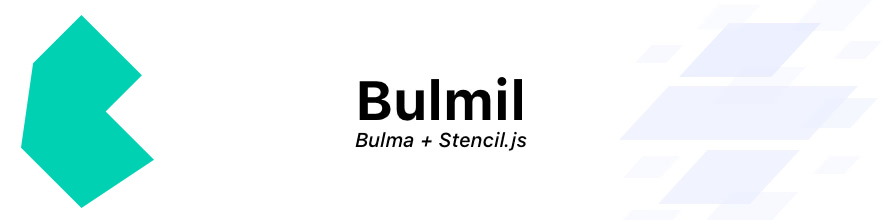
213 lines (212 loc) • 6.4 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class Select {
constructor() {
/**
* Control classes
*/
this.controlClass = '';
/**
* Allows multiple selection
*/
this.isMultiple = false;
/**
* Loading state
*/
this.isLoading = false;
/**
* Rounded
*/
this.isRounded = false;
}
render() {
return (h("div", { class: {
control: true,
'has-icons-left': Boolean(this.icon),
[this.controlClass]: Boolean(this.controlClass),
} },
h("div", { class: {
select: true,
[this.color]: Boolean(this.color),
[this.size]: Boolean(this.size),
'is-multiple': this.isMultiple,
'is-rounded': this.isRounded,
'is-loading': this.isLoading,
} },
h("select", { multiple: this.isMultiple, class: {
[this.state]: Boolean(this.state),
} },
h("slot", null))),
Boolean(this.icon) && (h("span", { class: {
icon: true,
'is-left': true,
[this.size]: Boolean(this.size),
} },
h("i", { class: this.icon })))));
}
static get is() { return "bm-select"; }
static get originalStyleUrls() { return {
"$": ["select.scss"]
}; }
static get styleUrls() { return {
"$": ["select.css"]
}; }
static get properties() { return {
"controlClass": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Control classes"
},
"attribute": "control-class",
"reflect": false,
"defaultValue": "''"
},
"value": {
"type": "any",
"mutable": false,
"complexType": {
"original": "string | number",
"resolved": "number | string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Value"
},
"attribute": "value",
"reflect": false
},
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger'",
"resolved": "\"is-danger\" | \"is-info\" | \"is-primary\" | \"is-success\" | \"is-warning\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"size": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-small' | 'is-normal' | 'is-medium' | 'is-large'",
"resolved": "\"is-large\" | \"is-medium\" | \"is-normal\" | \"is-small\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Size"
},
"attribute": "size",
"reflect": false
},
"state": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-hovered' | 'is-focused'",
"resolved": "\"is-focused\" | \"is-hovered\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "State"
},
"attribute": "state",
"reflect": false
},
"isMultiple": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Allows multiple selection"
},
"attribute": "is-multiple",
"reflect": false,
"defaultValue": "false"
},
"isLoading": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Loading state"
},
"attribute": "is-loading",
"reflect": false,
"defaultValue": "false"
},
"isRounded": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Rounded"
},
"attribute": "is-rounded",
"reflect": false,
"defaultValue": "false"
},
"icon": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Icon"
},
"attribute": "icon",
"reflect": false
}
}; }
}