bulmil
Version:
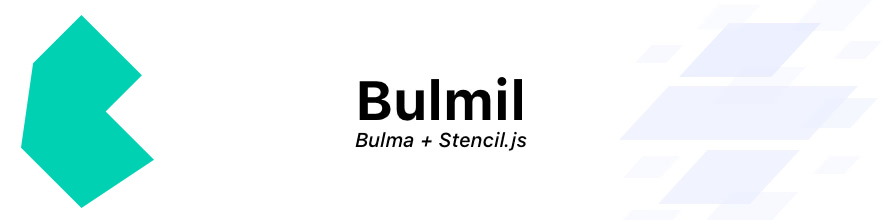
130 lines (129 loc) • 3.57 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class Radio {
constructor() {
/**
* Input class
*/
this.inputClass = '';
/**
* Label Classes
*/
this.labelClass = '';
/**
* Checked
*/
this.checked = false;
/**
* Disabled
*/
this.disabled = false;
}
render() {
return (h("label", { class: {
radio: true,
[this.labelClass]: Boolean(this.labelClass),
} },
h("input", { class: {
[this.inputClass]: Boolean(this.inputClass),
}, type: "radio", name: this.name, disabled: this.disabled, checked: this.checked }),
h("slot", null)));
}
static get is() { return "bm-radio"; }
static get originalStyleUrls() { return {
"$": ["radio.scss"]
}; }
static get styleUrls() { return {
"$": ["radio.css"]
}; }
static get properties() { return {
"inputClass": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Input class"
},
"attribute": "input-class",
"reflect": false,
"defaultValue": "''"
},
"labelClass": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Label Classes"
},
"attribute": "label-class",
"reflect": false,
"defaultValue": "''"
},
"name": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Name"
},
"attribute": "name",
"reflect": false
},
"checked": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Checked"
},
"attribute": "checked",
"reflect": false,
"defaultValue": "false"
},
"disabled": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Disabled"
},
"attribute": "disabled",
"reflect": false,
"defaultValue": "false"
}
}; }
}