bulmil
Version:
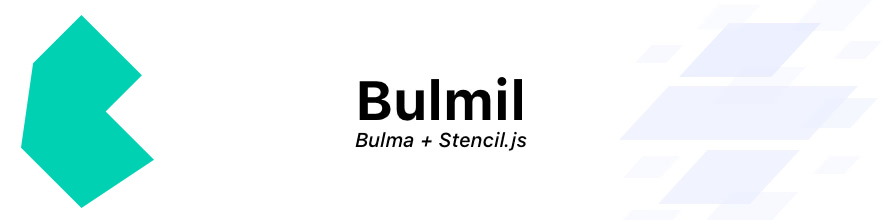
313 lines (312 loc) • 9.34 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class Input {
constructor() {
/**
* Control Classes
*/
this.controlClass = '';
/**
* Type
*/
this.type = 'text';
/**
* Name
*/
this.name = '';
/**
* Required
*/
this.required = false;
/**
* Disabled state
*/
this.disabled = false;
/**
* The input will look similar to a normal one, but is not editable and has no shadow
*/
this.readonly = false;
/**
* Rounded
*/
this.isRounded = false;
/**
* Loading state
*/
this.isLoading = false;
/**
* Removes the background, border, shadow, and horizontal padding
*/
this.isStatic = false;
}
render() {
return (h("div", { class: {
control: true,
'is-loading': this.isLoading,
[this.controlClass]: Boolean(this.controlClass),
} },
h("input", { class: {
input: true,
[this.color]: Boolean(this.color),
[this.size]: Boolean(this.size),
[this.state]: Boolean(this.state),
'is-rounded': this.isRounded,
'is-static': this.isStatic,
}, placeholder: this.placeholder, required: this.required, disabled: this.disabled, readonly: this.readonly, name: this.name, type: this.type, value: this.value })));
}
static get is() { return "bm-input"; }
static get originalStyleUrls() { return {
"$": ["input.scss"]
}; }
static get styleUrls() { return {
"$": ["input.css"]
}; }
static get properties() { return {
"controlClass": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Control Classes"
},
"attribute": "control-class",
"reflect": false,
"defaultValue": "''"
},
"placeholder": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Placeholder"
},
"attribute": "placeholder",
"reflect": false
},
"value": {
"type": "any",
"mutable": false,
"complexType": {
"original": "string | number",
"resolved": "number | string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Value"
},
"attribute": "value",
"reflect": false
},
"type": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'text' | 'number' | 'email' | 'password'",
"resolved": "\"email\" | \"number\" | \"password\" | \"text\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Type"
},
"attribute": "type",
"reflect": false,
"defaultValue": "'text'"
},
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-primary' | 'is-info' | 'is-success' | 'is-warning' | 'is-danger'",
"resolved": "\"is-danger\" | \"is-info\" | \"is-primary\" | \"is-success\" | \"is-warning\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"size": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-small' | 'is-normal' | 'is-medium' | 'is-large'",
"resolved": "\"is-large\" | \"is-medium\" | \"is-normal\" | \"is-small\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Size"
},
"attribute": "size",
"reflect": false
},
"state": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-hovered' | 'is-focused'",
"resolved": "\"is-focused\" | \"is-hovered\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "State"
},
"attribute": "state",
"reflect": false
},
"name": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Name"
},
"attribute": "name",
"reflect": false,
"defaultValue": "''"
},
"required": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Required"
},
"attribute": "required",
"reflect": false,
"defaultValue": "false"
},
"disabled": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Disabled state"
},
"attribute": "disabled",
"reflect": false,
"defaultValue": "false"
},
"readonly": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "The input will look similar to a normal one, but is not editable and has no shadow"
},
"attribute": "readonly",
"reflect": false,
"defaultValue": "false"
},
"isRounded": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Rounded"
},
"attribute": "is-rounded",
"reflect": false,
"defaultValue": "false"
},
"isLoading": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Loading state"
},
"attribute": "is-loading",
"reflect": false,
"defaultValue": "false"
},
"isStatic": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Removes the background, border, shadow, and horizontal padding"
},
"attribute": "is-static",
"reflect": false,
"defaultValue": "false"
}
}; }
}