bulmil
Version:
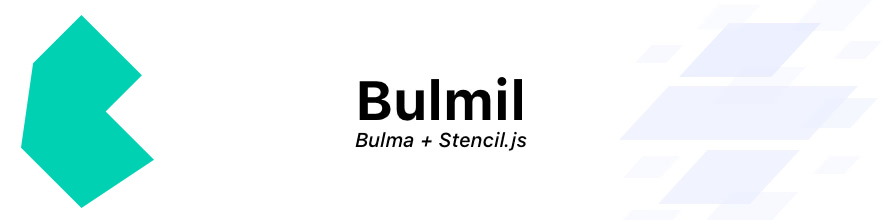
245 lines (244 loc) • 7.61 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class FileInput {
constructor() {
/**
* Name
*/
this.name = '';
/**
* Placeholder
*/
this.placeholder = 'Choose a file…';
/**
* Add a placeholder for the selected file name
*/
this.hasName = false;
/**
* Move the CTA to the right side
*/
this.isRight = false;
/**
* Expand the name to fill up the space
*/
this.isFullwidth = false;
/**
* Boxed block
*/
this.isBoxed = false;
this.handleFileChange = (event) => {
this.file = event.target.files[0];
};
}
get fileName() {
return this.file && this.file.name;
}
get displayName() {
return this.hasName && Boolean(this.fileName);
}
render() {
return (h("div", { class: {
file: true,
[this.size]: Boolean(this.size),
[this.color]: Boolean(this.color),
[this.alignment]: Boolean(this.alignment),
'has-name': this.displayName,
'is-right': this.isRight,
'is-fullwidth': this.isFullwidth,
'is-boxed': this.isBoxed,
} },
h("label", { class: "file-label" },
h("input", { onChange: this.handleFileChange, class: "file-input", type: "file", name: this.name }),
h("span", { class: "file-cta" },
h("span", { class: "file-icon" },
h("i", { class: "fas fa-upload" })),
h("span", { class: "file-label" }, this.placeholder)),
this.displayName && h("span", { class: "file-name" }, this.fileName))));
}
static get is() { return "bm-file"; }
static get originalStyleUrls() { return {
"$": ["file.scss"]
}; }
static get styleUrls() { return {
"$": ["file.css"]
}; }
static get properties() { return {
"name": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Name"
},
"attribute": "name",
"reflect": false,
"defaultValue": "''"
},
"placeholder": {
"type": "string",
"mutable": false,
"complexType": {
"original": "string",
"resolved": "string",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Placeholder"
},
"attribute": "placeholder",
"reflect": false,
"defaultValue": "'Choose a file\u2026'"
},
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "| 'is-white'\n | 'is-black'\n | 'is-light'\n | 'is-dark'\n | 'is-primary'\n | 'is-link'\n | 'is-info'\n | 'is-success'\n | 'is-warning'\n | 'is-danger'",
"resolved": "\"is-black\" | \"is-danger\" | \"is-dark\" | \"is-info\" | \"is-light\" | \"is-link\" | \"is-primary\" | \"is-success\" | \"is-warning\" | \"is-white\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"size": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-small' | 'is-medium' | 'is-large'",
"resolved": "\"is-large\" | \"is-medium\" | \"is-small\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Size"
},
"attribute": "size",
"reflect": false
},
"alignment": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-centered' | 'is-right'",
"resolved": "\"is-centered\" | \"is-right\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Alignment"
},
"attribute": "alignment",
"reflect": false
},
"hasName": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Add a placeholder for the selected file name"
},
"attribute": "has-name",
"reflect": false,
"defaultValue": "false"
},
"isRight": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Move the CTA to the right side"
},
"attribute": "is-right",
"reflect": false,
"defaultValue": "false"
},
"isFullwidth": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Expand the name to fill up the space"
},
"attribute": "is-fullwidth",
"reflect": false,
"defaultValue": "false"
},
"file": {
"type": "unknown",
"mutable": true,
"complexType": {
"original": "File",
"resolved": "File",
"references": {
"File": {
"location": "global"
}
}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "File"
}
},
"isBoxed": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Boxed block"
},
"attribute": "is-boxed",
"reflect": false,
"defaultValue": "false"
}
}; }
}