bulmil
Version:
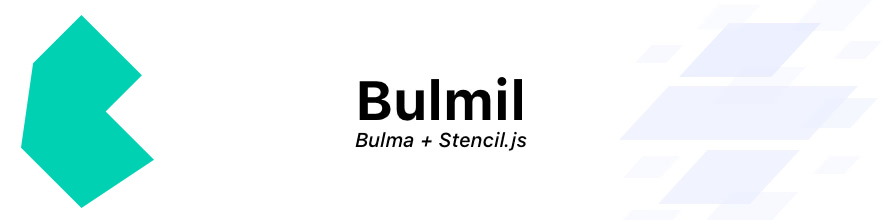
309 lines (308 loc) • 9.13 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class Button {
constructor() {
/**
* Is disabled?
*/
this.disabled = false;
/**
* Display the button in full-width
*/
this.isFullwidth = false;
/**
* Is outlined
*/
this.isOutlined = false;
/**
* Is light
*/
this.isLight = false;
/**
* Is inverted
*/
this.isInverted = false;
/**
* Rounded button
*/
this.isRounded = false;
/**
* Hovered state
*/
this.isHovered = false;
/**
* Focused state
*/
this.isFocused = false;
/**
* Active state
*/
this.isActive = false;
/**
* Static
*/
this.isStatic = false;
/**
* Loading state
*/
this.isLoading = false;
}
render() {
return (h("button", { class: {
button: true,
[this.color]: Boolean(this.color),
[this.size]: Boolean(this.size),
'is-fullwidth': this.isFullwidth,
'is-active': this.isActive,
'is-light': this.isLight,
'is-focused': this.isFocused,
'is-static': this.isStatic,
'is-hovered': this.isHovered,
'is-inverted': this.isInverted,
'is-rounded': this.isRounded,
'is-loading': this.isLoading,
'is-outlined': this.isOutlined,
}, disabled: this.disabled, "aria-disabled": this.disabled ? 'true' : null },
h("slot", null)));
}
static get is() { return "bm-button"; }
static get originalStyleUrls() { return {
"$": ["button.scss"]
}; }
static get styleUrls() { return {
"$": ["button.css"]
}; }
static get properties() { return {
"disabled": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is disabled?"
},
"attribute": "disabled",
"reflect": false,
"defaultValue": "false"
},
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "| 'is-primary'\n | 'is-link'\n | 'is-info'\n | 'is-success'\n | 'is-danger'\n | 'is-warning'\n | 'is-white'\n | 'is-light'\n | 'is-dark'\n | 'is-black'\n | 'is-text'",
"resolved": "\"is-black\" | \"is-danger\" | \"is-dark\" | \"is-info\" | \"is-light\" | \"is-link\" | \"is-primary\" | \"is-success\" | \"is-text\" | \"is-warning\" | \"is-white\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"size": {
"type": "string",
"mutable": false,
"complexType": {
"original": "'is-small' | 'is-medium' | 'is-large' | 'is-normal'",
"resolved": "\"is-large\" | \"is-medium\" | \"is-normal\" | \"is-small\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Size"
},
"attribute": "size",
"reflect": false
},
"isFullwidth": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Display the button in full-width"
},
"attribute": "is-fullwidth",
"reflect": false,
"defaultValue": "false"
},
"isOutlined": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is outlined"
},
"attribute": "is-outlined",
"reflect": false,
"defaultValue": "false"
},
"isLight": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is light"
},
"attribute": "is-light",
"reflect": false,
"defaultValue": "false"
},
"isInverted": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is inverted"
},
"attribute": "is-inverted",
"reflect": false,
"defaultValue": "false"
},
"isRounded": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Rounded button"
},
"attribute": "is-rounded",
"reflect": false,
"defaultValue": "false"
},
"isHovered": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Hovered state"
},
"attribute": "is-hovered",
"reflect": false,
"defaultValue": "false"
},
"isFocused": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Focused state"
},
"attribute": "is-focused",
"reflect": false,
"defaultValue": "false"
},
"isActive": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Active state"
},
"attribute": "is-active",
"reflect": false,
"defaultValue": "false"
},
"isStatic": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Static"
},
"attribute": "is-static",
"reflect": false,
"defaultValue": "false"
},
"isLoading": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Loading state"
},
"attribute": "is-loading",
"reflect": false,
"defaultValue": "false"
}
}; }
}