bulmil
Version:
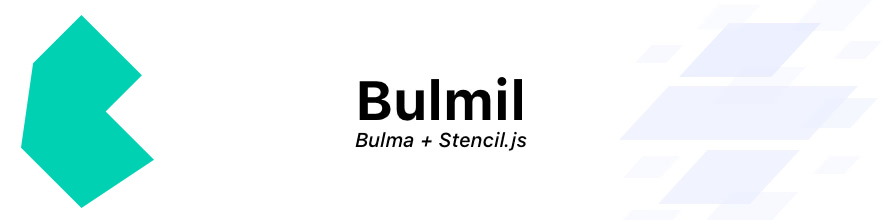
103 lines (102 loc) • 3.34 kB
JavaScript
import { Component, Prop, h } from '@stencil/core';
export class Badge {
constructor() {
/**
* Is outlined
*/
this.isOutlined = false;
/**
* Is light
*/
this.isLight = false;
}
render() {
return (h("span", { class: {
badge: true,
[this.color]: Boolean(this.color),
[this.position]: Boolean(this.position),
'is-light': this.isLight,
'is-outlined': this.isOutlined,
} },
h("slot", null)));
}
static get is() { return "bm-badge"; }
static get originalStyleUrls() { return {
"$": ["badge.scss"]
}; }
static get styleUrls() { return {
"$": ["badge.css"]
}; }
static get properties() { return {
"color": {
"type": "string",
"mutable": false,
"complexType": {
"original": "| 'is-primary'\n | 'is-info'\n | 'is-success'\n | 'is-danger'\n | 'is-warning'\n | 'is-white'\n | 'is-dark'\n | 'is-black'",
"resolved": "\"is-black\" | \"is-danger\" | \"is-dark\" | \"is-info\" | \"is-primary\" | \"is-success\" | \"is-warning\" | \"is-white\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Color"
},
"attribute": "color",
"reflect": false
},
"position": {
"type": "string",
"mutable": false,
"complexType": {
"original": "| 'is-top-left'\n | 'is-top'\n | 'is-top-right'\n | 'is-right'\n | 'is-bottom-right'\n | 'is-bottom'\n | 'is-bottom-left'\n | 'is-left'",
"resolved": "\"is-bottom\" | \"is-bottom-left\" | \"is-bottom-right\" | \"is-left\" | \"is-right\" | \"is-top\" | \"is-top-left\" | \"is-top-right\"",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Position"
},
"attribute": "position",
"reflect": false
},
"isOutlined": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is outlined"
},
"attribute": "is-outlined",
"reflect": false,
"defaultValue": "false"
},
"isLight": {
"type": "boolean",
"mutable": false,
"complexType": {
"original": "boolean",
"resolved": "boolean",
"references": {}
},
"required": false,
"optional": false,
"docs": {
"tags": [],
"text": "Is light"
},
"attribute": "is-light",
"reflect": false,
"defaultValue": "false"
}
}; }
}