bulmil
Version:
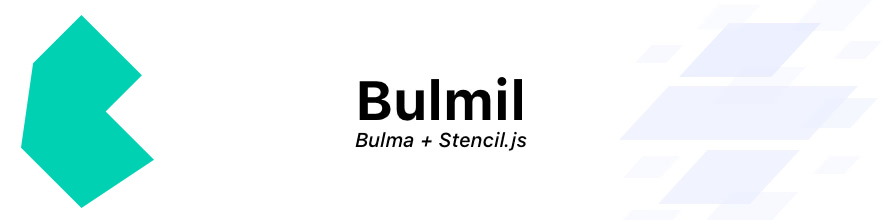
1,237 lines (1,234 loc) • 68.4 kB
JavaScript
'use strict';
function _interopNamespace(e) {
if (e && e.__esModule) { return e; } else {
var n = {};
if (e) {
Object.keys(e).forEach(function (k) {
var d = Object.getOwnPropertyDescriptor(e, k);
Object.defineProperty(n, k, d.get ? d : {
enumerable: true,
get: function () {
return e[k];
}
});
});
}
n['default'] = e;
return n;
}
}
const NAMESPACE = 'bulmil';
let contentRef;
let hostTagName;
let useNativeShadowDom = false;
let checkSlotFallbackVisibility = false;
let checkSlotRelocate = false;
let isSvgMode = false;
let queueCongestion = 0;
let queuePending = false;
const win = typeof window !== 'undefined' ? window : {};
const CSS = win.CSS ;
const doc = win.document || { head: {} };
const plt = {
$flags$: 0,
$resourcesUrl$: '',
jmp: h => h(),
raf: h => requestAnimationFrame(h),
ael: (el, eventName, listener, opts) => el.addEventListener(eventName, listener, opts),
rel: (el, eventName, listener, opts) => el.removeEventListener(eventName, listener, opts),
ce: (eventName, opts) => new CustomEvent(eventName, opts),
};
const supportsShadow = true;
const promiseResolve = (v) => Promise.resolve(v);
const supportsConstructibleStylesheets = /*@__PURE__*/ (() => {
try {
new CSSStyleSheet();
return true;
}
catch (e) { }
return false;
})()
;
const CONTENT_REF_ID = 'r';
const ORG_LOCATION_ID = 'o';
const SLOT_NODE_ID = 's';
const TEXT_NODE_ID = 't';
const HYDRATE_ID = 's-id';
const HYDRATED_STYLE_ID = 'sty-id';
const HYDRATE_CHILD_ID = 'c-id';
const HYDRATED_CSS = '{visibility:hidden}.hydrated{visibility:inherit}';
const createTime = (fnName, tagName = '') => {
{
return () => {
return;
};
}
};
const uniqueTime = (key, measureText) => {
{
return () => {
return;
};
}
};
const rootAppliedStyles = new WeakMap();
const registerStyle = (scopeId, cssText, allowCS) => {
let style = styles.get(scopeId);
if (supportsConstructibleStylesheets && allowCS) {
style = (style || new CSSStyleSheet());
style.replace(cssText);
}
else {
style = cssText;
}
styles.set(scopeId, style);
};
const addStyle = (styleContainerNode, cmpMeta, mode, hostElm) => {
let scopeId = getScopeId(cmpMeta);
let style = styles.get(scopeId);
// if an element is NOT connected then getRootNode() will return the wrong root node
// so the fallback is to always use the document for the root node in those cases
styleContainerNode = styleContainerNode.nodeType === 11 /* DocumentFragment */ ? styleContainerNode : doc;
if (style) {
if (typeof style === 'string') {
styleContainerNode = styleContainerNode.head || styleContainerNode;
let appliedStyles = rootAppliedStyles.get(styleContainerNode);
let styleElm;
if (!appliedStyles) {
rootAppliedStyles.set(styleContainerNode, (appliedStyles = new Set()));
}
if (!appliedStyles.has(scopeId)) {
if ( styleContainerNode.host && (styleElm = styleContainerNode.querySelector(`[${HYDRATED_STYLE_ID}="${scopeId}"]`))) {
// This is only happening on native shadow-dom, do not needs CSS var shim
styleElm.innerHTML = style;
}
else {
if ( plt.$cssShim$) {
styleElm = plt.$cssShim$.createHostStyle(hostElm, scopeId, style, !!(cmpMeta.$flags$ & 10 /* needsScopedEncapsulation */));
const newScopeId = styleElm['s-sc'];
if (newScopeId) {
scopeId = newScopeId;
// we don't want to add this styleID to the appliedStyles Set
// since the cssVarShim might need to apply several different
// stylesheets for the same component
appliedStyles = null;
}
}
else {
styleElm = doc.createElement('style');
styleElm.innerHTML = style;
}
styleContainerNode.insertBefore(styleElm, styleContainerNode.querySelector('link'));
}
if (appliedStyles) {
appliedStyles.add(scopeId);
}
}
}
else if ( !styleContainerNode.adoptedStyleSheets.includes(style)) {
styleContainerNode.adoptedStyleSheets = [...styleContainerNode.adoptedStyleSheets, style];
}
}
return scopeId;
};
const attachStyles = (hostRef) => {
const cmpMeta = hostRef.$cmpMeta$;
const elm = hostRef.$hostElement$;
const endAttachStyles = createTime('attachStyles', cmpMeta.$tagName$);
const scopeId = addStyle( elm.getRootNode(), cmpMeta, hostRef.$modeName$, elm);
endAttachStyles();
};
const getScopeId = (cmp, mode) => 'sc-' + ( cmp.$tagName$);
/**
* Default style mode id
*/
/**
* Reusable empty obj/array
* Don't add values to these!!
*/
const EMPTY_OBJ = {};
const noop = () => {
/* noop*/
};
const isComplexType = (o) => {
// https://jsperf.com/typeof-fn-object/5
o = typeof o;
return o === 'object' || o === 'function';
};
const IS_DENO_ENV = typeof Deno !== 'undefined';
const IS_NODE_ENV = !IS_DENO_ENV &&
typeof global !== 'undefined' &&
typeof require === 'function' &&
!!global.process &&
typeof __filename === 'string' &&
(!global.origin || typeof global.origin !== 'string');
const IS_DENO_WINDOWS_ENV = IS_DENO_ENV && Deno.build.os === 'windows';
const getCurrentDirectory = IS_NODE_ENV ? process.cwd : IS_DENO_ENV ? Deno.cwd : () => '/';
const exit = IS_NODE_ENV ? process.exit : IS_DENO_ENV ? Deno.exit : noop;
/**
* Production h() function based on Preact by
* Jason Miller (@developit)
* Licensed under the MIT License
* https://github.com/developit/preact/blob/master/LICENSE
*
* Modified for Stencil's compiler and vdom
*/
// const stack: any[] = [];
// export function h(nodeName: string | d.FunctionalComponent, vnodeData: d.PropsType, child?: d.ChildType): d.VNode;
// export function h(nodeName: string | d.FunctionalComponent, vnodeData: d.PropsType, ...children: d.ChildType[]): d.VNode;
const h = (nodeName, vnodeData, ...children) => {
let child = null;
let slotName = null;
let simple = false;
let lastSimple = false;
let vNodeChildren = [];
const walk = (c) => {
for (let i = 0; i < c.length; i++) {
child = c[i];
if (Array.isArray(child)) {
walk(child);
}
else if (child != null && typeof child !== 'boolean') {
if ((simple = typeof nodeName !== 'function' && !isComplexType(child))) {
child = String(child);
}
if (simple && lastSimple) {
// If the previous child was simple (string), we merge both
vNodeChildren[vNodeChildren.length - 1].$text$ += child;
}
else {
// Append a new vNode, if it's text, we create a text vNode
vNodeChildren.push(simple ? newVNode(null, child) : child);
}
lastSimple = simple;
}
}
};
walk(children);
if (vnodeData) {
if ( vnodeData.name) {
slotName = vnodeData.name;
}
{
const classData = vnodeData.className || vnodeData.class;
if (classData) {
vnodeData.class =
typeof classData !== 'object'
? classData
: Object.keys(classData)
.filter(k => classData[k])
.join(' ');
}
}
}
if ( typeof nodeName === 'function') {
// nodeName is a functional component
return nodeName(vnodeData === null ? {} : vnodeData, vNodeChildren, vdomFnUtils);
}
const vnode = newVNode(nodeName, null);
vnode.$attrs$ = vnodeData;
if (vNodeChildren.length > 0) {
vnode.$children$ = vNodeChildren;
}
{
vnode.$name$ = slotName;
}
return vnode;
};
const newVNode = (tag, text) => {
const vnode = {
$flags$: 0,
$tag$: tag,
$text$: text,
$elm$: null,
$children$: null,
};
{
vnode.$attrs$ = null;
}
{
vnode.$name$ = null;
}
return vnode;
};
const Host = {};
const isHost = (node) => node && node.$tag$ === Host;
const vdomFnUtils = {
forEach: (children, cb) => children.map(convertToPublic).forEach(cb),
map: (children, cb) => children
.map(convertToPublic)
.map(cb)
.map(convertToPrivate),
};
const convertToPublic = (node) => ({
vattrs: node.$attrs$,
vchildren: node.$children$,
vkey: node.$key$,
vname: node.$name$,
vtag: node.$tag$,
vtext: node.$text$,
});
const convertToPrivate = (node) => {
if (typeof node.vtag === 'function') {
const vnodeData = Object.assign({}, node.vattrs);
if (node.vkey) {
vnodeData.key = node.vkey;
}
if (node.vname) {
vnodeData.name = node.vname;
}
return h(node.vtag, vnodeData, ...node.vchildren || []);
}
const vnode = newVNode(node.vtag, node.vtext);
vnode.$attrs$ = node.vattrs;
vnode.$children$ = node.vchildren;
vnode.$key$ = node.vkey;
vnode.$name$ = node.vname;
return vnode;
};
/**
* Production setAccessor() function based on Preact by
* Jason Miller (@developit)
* Licensed under the MIT License
* https://github.com/developit/preact/blob/master/LICENSE
*
* Modified for Stencil's compiler and vdom
*/
const setAccessor = (elm, memberName, oldValue, newValue, isSvg, flags) => {
if (oldValue !== newValue) {
let isProp = isMemberInElement(elm, memberName);
let ln = memberName.toLowerCase();
if ( memberName === 'class') {
const classList = elm.classList;
const oldClasses = parseClassList(oldValue);
const newClasses = parseClassList(newValue);
classList.remove(...oldClasses.filter(c => c && !newClasses.includes(c)));
classList.add(...newClasses.filter(c => c && !oldClasses.includes(c)));
}
else if ( ( !isProp ) && memberName[0] === 'o' && memberName[1] === 'n') {
// Event Handlers
// so if the member name starts with "on" and the 3rd characters is
// a capital letter, and it's not already a member on the element,
// then we're assuming it's an event listener
if (memberName[2] === '-') {
// on- prefixed events
// allows to be explicit about the dom event to listen without any magic
// under the hood:
// <my-cmp on-click> // listens for "click"
// <my-cmp on-Click> // listens for "Click"
// <my-cmp on-ionChange> // listens for "ionChange"
// <my-cmp on-EVENTS> // listens for "EVENTS"
memberName = memberName.slice(3);
}
else if (isMemberInElement(win, ln)) {
// standard event
// the JSX attribute could have been "onMouseOver" and the
// member name "onmouseover" is on the window's prototype
// so let's add the listener "mouseover", which is all lowercased
memberName = ln.slice(2);
}
else {
// custom event
// the JSX attribute could have been "onMyCustomEvent"
// so let's trim off the "on" prefix and lowercase the first character
// and add the listener "myCustomEvent"
// except for the first character, we keep the event name case
memberName = ln[2] + memberName.slice(3);
}
if (oldValue) {
plt.rel(elm, memberName, oldValue, false);
}
if (newValue) {
plt.ael(elm, memberName, newValue, false);
}
}
else {
// Set property if it exists and it's not a SVG
const isComplex = isComplexType(newValue);
if ((isProp || (isComplex && newValue !== null)) && !isSvg) {
try {
if (!elm.tagName.includes('-')) {
let n = newValue == null ? '' : newValue;
// Workaround for Safari, moving the <input> caret when re-assigning the same valued
if (memberName === 'list') {
isProp = false;
// tslint:disable-next-line: triple-equals
}
else if (oldValue == null || elm[memberName] != n) {
elm[memberName] = n;
}
}
else {
elm[memberName] = newValue;
}
}
catch (e) { }
}
if (newValue == null || newValue === false) {
if (newValue !== false || elm.getAttribute(memberName) === '') {
{
elm.removeAttribute(memberName);
}
}
}
else if ((!isProp || flags & 4 /* isHost */ || isSvg) && !isComplex) {
newValue = newValue === true ? '' : newValue;
{
elm.setAttribute(memberName, newValue);
}
}
}
}
};
const parseClassListRegex = /\s/;
const parseClassList = (value) => (!value ? [] : value.split(parseClassListRegex));
const updateElement = (oldVnode, newVnode, isSvgMode, memberName) => {
// if the element passed in is a shadow root, which is a document fragment
// then we want to be adding attrs/props to the shadow root's "host" element
// if it's not a shadow root, then we add attrs/props to the same element
const elm = newVnode.$elm$.nodeType === 11 /* DocumentFragment */ && newVnode.$elm$.host ? newVnode.$elm$.host : newVnode.$elm$;
const oldVnodeAttrs = (oldVnode && oldVnode.$attrs$) || EMPTY_OBJ;
const newVnodeAttrs = newVnode.$attrs$ || EMPTY_OBJ;
{
// remove attributes no longer present on the vnode by setting them to undefined
for (memberName in oldVnodeAttrs) {
if (!(memberName in newVnodeAttrs)) {
setAccessor(elm, memberName, oldVnodeAttrs[memberName], undefined, isSvgMode, newVnode.$flags$);
}
}
}
// add new & update changed attributes
for (memberName in newVnodeAttrs) {
setAccessor(elm, memberName, oldVnodeAttrs[memberName], newVnodeAttrs[memberName], isSvgMode, newVnode.$flags$);
}
};
const createElm = (oldParentVNode, newParentVNode, childIndex, parentElm) => {
// tslint:disable-next-line: prefer-const
let newVNode = newParentVNode.$children$[childIndex];
let i = 0;
let elm;
let childNode;
let oldVNode;
if ( !useNativeShadowDom) {
// remember for later we need to check to relocate nodes
checkSlotRelocate = true;
if (newVNode.$tag$ === 'slot') {
newVNode.$flags$ |= newVNode.$children$
? // slot element has fallback content
2 /* isSlotFallback */
: // slot element does not have fallback content
1 /* isSlotReference */;
}
}
if ( newVNode.$text$ !== null) {
// create text node
elm = newVNode.$elm$ = doc.createTextNode(newVNode.$text$);
}
else if ( newVNode.$flags$ & 1 /* isSlotReference */) {
// create a slot reference node
elm = newVNode.$elm$ = doc.createTextNode('');
}
else {
// create element
elm = newVNode.$elm$ = ( doc.createElement( newVNode.$flags$ & 2 /* isSlotFallback */ ? 'slot-fb' : newVNode.$tag$));
// add css classes, attrs, props, listeners, etc.
{
updateElement(null, newVNode, isSvgMode);
}
if (newVNode.$children$) {
for (i = 0; i < newVNode.$children$.length; ++i) {
// create the node
childNode = createElm(oldParentVNode, newVNode, i);
// return node could have been null
if (childNode) {
// append our new node
elm.appendChild(childNode);
}
}
}
}
{
elm['s-hn'] = hostTagName;
if (newVNode.$flags$ & (2 /* isSlotFallback */ | 1 /* isSlotReference */)) {
// remember the content reference comment
elm['s-sr'] = true;
// remember the content reference comment
elm['s-cr'] = contentRef;
// remember the slot name, or empty string for default slot
elm['s-sn'] = newVNode.$name$ || '';
// check if we've got an old vnode for this slot
oldVNode = oldParentVNode && oldParentVNode.$children$ && oldParentVNode.$children$[childIndex];
if (oldVNode && oldVNode.$tag$ === newVNode.$tag$ && oldParentVNode.$elm$) {
// we've got an old slot vnode and the wrapper is being replaced
// so let's move the old slot content back to it's original location
putBackInOriginalLocation(oldParentVNode.$elm$, false);
}
}
}
return elm;
};
const putBackInOriginalLocation = (parentElm, recursive) => {
plt.$flags$ |= 1 /* isTmpDisconnected */;
const oldSlotChildNodes = parentElm.childNodes;
for (let i = oldSlotChildNodes.length - 1; i >= 0; i--) {
const childNode = oldSlotChildNodes[i];
if (childNode['s-hn'] !== hostTagName && childNode['s-ol']) {
// // this child node in the old element is from another component
// // remove this node from the old slot's parent
// childNode.remove();
// and relocate it back to it's original location
parentReferenceNode(childNode).insertBefore(childNode, referenceNode(childNode));
// remove the old original location comment entirely
// later on the patch function will know what to do
// and move this to the correct spot in need be
childNode['s-ol'].remove();
childNode['s-ol'] = undefined;
checkSlotRelocate = true;
}
if (recursive) {
putBackInOriginalLocation(childNode, recursive);
}
}
plt.$flags$ &= ~1 /* isTmpDisconnected */;
};
const addVnodes = (parentElm, before, parentVNode, vnodes, startIdx, endIdx) => {
let containerElm = (( parentElm['s-cr'] && parentElm['s-cr'].parentNode) || parentElm);
let childNode;
for (; startIdx <= endIdx; ++startIdx) {
if (vnodes[startIdx]) {
childNode = createElm(null, parentVNode, startIdx);
if (childNode) {
vnodes[startIdx].$elm$ = childNode;
containerElm.insertBefore(childNode, referenceNode(before) );
}
}
}
};
const removeVnodes = (vnodes, startIdx, endIdx, vnode, elm) => {
for (; startIdx <= endIdx; ++startIdx) {
if ((vnode = vnodes[startIdx])) {
elm = vnode.$elm$;
{
// we're removing this element
// so it's possible we need to show slot fallback content now
checkSlotFallbackVisibility = true;
if (elm['s-ol']) {
// remove the original location comment
elm['s-ol'].remove();
}
else {
// it's possible that child nodes of the node
// that's being removed are slot nodes
putBackInOriginalLocation(elm, true);
}
}
// remove the vnode's element from the dom
elm.remove();
}
}
};
const updateChildren = (parentElm, oldCh, newVNode, newCh) => {
let oldStartIdx = 0;
let newStartIdx = 0;
let oldEndIdx = oldCh.length - 1;
let oldStartVnode = oldCh[0];
let oldEndVnode = oldCh[oldEndIdx];
let newEndIdx = newCh.length - 1;
let newStartVnode = newCh[0];
let newEndVnode = newCh[newEndIdx];
let node;
while (oldStartIdx <= oldEndIdx && newStartIdx <= newEndIdx) {
if (oldStartVnode == null) {
// Vnode might have been moved left
oldStartVnode = oldCh[++oldStartIdx];
}
else if (oldEndVnode == null) {
oldEndVnode = oldCh[--oldEndIdx];
}
else if (newStartVnode == null) {
newStartVnode = newCh[++newStartIdx];
}
else if (newEndVnode == null) {
newEndVnode = newCh[--newEndIdx];
}
else if (isSameVnode(oldStartVnode, newStartVnode)) {
patch(oldStartVnode, newStartVnode);
oldStartVnode = oldCh[++oldStartIdx];
newStartVnode = newCh[++newStartIdx];
}
else if (isSameVnode(oldEndVnode, newEndVnode)) {
patch(oldEndVnode, newEndVnode);
oldEndVnode = oldCh[--oldEndIdx];
newEndVnode = newCh[--newEndIdx];
}
else if (isSameVnode(oldStartVnode, newEndVnode)) {
// Vnode moved right
if ( (oldStartVnode.$tag$ === 'slot' || newEndVnode.$tag$ === 'slot')) {
putBackInOriginalLocation(oldStartVnode.$elm$.parentNode, false);
}
patch(oldStartVnode, newEndVnode);
parentElm.insertBefore(oldStartVnode.$elm$, oldEndVnode.$elm$.nextSibling);
oldStartVnode = oldCh[++oldStartIdx];
newEndVnode = newCh[--newEndIdx];
}
else if (isSameVnode(oldEndVnode, newStartVnode)) {
// Vnode moved left
if ( (oldStartVnode.$tag$ === 'slot' || newEndVnode.$tag$ === 'slot')) {
putBackInOriginalLocation(oldEndVnode.$elm$.parentNode, false);
}
patch(oldEndVnode, newStartVnode);
parentElm.insertBefore(oldEndVnode.$elm$, oldStartVnode.$elm$);
oldEndVnode = oldCh[--oldEndIdx];
newStartVnode = newCh[++newStartIdx];
}
else {
{
// new element
node = createElm(oldCh && oldCh[newStartIdx], newVNode, newStartIdx);
newStartVnode = newCh[++newStartIdx];
}
if (node) {
{
parentReferenceNode(oldStartVnode.$elm$).insertBefore(node, referenceNode(oldStartVnode.$elm$));
}
}
}
}
if (oldStartIdx > oldEndIdx) {
addVnodes(parentElm, newCh[newEndIdx + 1] == null ? null : newCh[newEndIdx + 1].$elm$, newVNode, newCh, newStartIdx, newEndIdx);
}
else if ( newStartIdx > newEndIdx) {
removeVnodes(oldCh, oldStartIdx, oldEndIdx);
}
};
const isSameVnode = (vnode1, vnode2) => {
// compare if two vnode to see if they're "technically" the same
// need to have the same element tag, and same key to be the same
if (vnode1.$tag$ === vnode2.$tag$) {
if ( vnode1.$tag$ === 'slot') {
return vnode1.$name$ === vnode2.$name$;
}
return true;
}
return false;
};
const referenceNode = (node) => {
// this node was relocated to a new location in the dom
// because of some other component's slot
// but we still have an html comment in place of where
// it's original location was according to it's original vdom
return (node && node['s-ol']) || node;
};
const parentReferenceNode = (node) => (node['s-ol'] ? node['s-ol'] : node).parentNode;
const patch = (oldVNode, newVNode) => {
const elm = (newVNode.$elm$ = oldVNode.$elm$);
const oldChildren = oldVNode.$children$;
const newChildren = newVNode.$children$;
const tag = newVNode.$tag$;
const text = newVNode.$text$;
let defaultHolder;
if ( text === null) {
// element node
{
if ( tag === 'slot')
;
else {
// either this is the first render of an element OR it's an update
// AND we already know it's possible it could have changed
// this updates the element's css classes, attrs, props, listeners, etc.
updateElement(oldVNode, newVNode, isSvgMode);
}
}
if ( oldChildren !== null && newChildren !== null) {
// looks like there's child vnodes for both the old and new vnodes
updateChildren(elm, oldChildren, newVNode, newChildren);
}
else if (newChildren !== null) {
// no old child vnodes, but there are new child vnodes to add
if ( oldVNode.$text$ !== null) {
// the old vnode was text, so be sure to clear it out
elm.textContent = '';
}
// add the new vnode children
addVnodes(elm, null, newVNode, newChildren, 0, newChildren.length - 1);
}
else if ( oldChildren !== null) {
// no new child vnodes, but there are old child vnodes to remove
removeVnodes(oldChildren, 0, oldChildren.length - 1);
}
}
else if ( (defaultHolder = elm['s-cr'])) {
// this element has slotted content
defaultHolder.parentNode.textContent = text;
}
else if ( oldVNode.$text$ !== text) {
// update the text content for the text only vnode
// and also only if the text is different than before
elm.data = text;
}
};
const updateFallbackSlotVisibility = (elm) => {
// tslint:disable-next-line: prefer-const
let childNodes = elm.childNodes;
let childNode;
let i;
let ilen;
let j;
let slotNameAttr;
let nodeType;
for (i = 0, ilen = childNodes.length; i < ilen; i++) {
childNode = childNodes[i];
if (childNode.nodeType === 1 /* ElementNode */) {
if (childNode['s-sr']) {
// this is a slot fallback node
// get the slot name for this slot reference node
slotNameAttr = childNode['s-sn'];
// by default always show a fallback slot node
// then hide it if there are other slots in the light dom
childNode.hidden = false;
for (j = 0; j < ilen; j++) {
if (childNodes[j]['s-hn'] !== childNode['s-hn']) {
// this sibling node is from a different component
nodeType = childNodes[j].nodeType;
if (slotNameAttr !== '') {
// this is a named fallback slot node
if (nodeType === 1 /* ElementNode */ && slotNameAttr === childNodes[j].getAttribute('slot')) {
childNode.hidden = true;
break;
}
}
else {
// this is a default fallback slot node
// any element or text node (with content)
// should hide the default fallback slot node
if (nodeType === 1 /* ElementNode */ || (nodeType === 3 /* TextNode */ && childNodes[j].textContent.trim() !== '')) {
childNode.hidden = true;
break;
}
}
}
}
}
// keep drilling down
updateFallbackSlotVisibility(childNode);
}
}
};
const relocateNodes = [];
const relocateSlotContent = (elm) => {
// tslint:disable-next-line: prefer-const
let childNode;
let node;
let hostContentNodes;
let slotNameAttr;
let relocateNodeData;
let j;
let i = 0;
let childNodes = elm.childNodes;
let ilen = childNodes.length;
for (; i < ilen; i++) {
childNode = childNodes[i];
if (childNode['s-sr'] && (node = childNode['s-cr'])) {
// first got the content reference comment node
// then we got it's parent, which is where all the host content is in now
hostContentNodes = node.parentNode.childNodes;
slotNameAttr = childNode['s-sn'];
for (j = hostContentNodes.length - 1; j >= 0; j--) {
node = hostContentNodes[j];
if (!node['s-cn'] && !node['s-nr'] && node['s-hn'] !== childNode['s-hn']) {
// let's do some relocating to its new home
// but never relocate a content reference node
// that is suppose to always represent the original content location
if (isNodeLocatedInSlot(node, slotNameAttr)) {
// it's possible we've already decided to relocate this node
relocateNodeData = relocateNodes.find(r => r.$nodeToRelocate$ === node);
// made some changes to slots
// let's make sure we also double check
// fallbacks are correctly hidden or shown
checkSlotFallbackVisibility = true;
node['s-sn'] = node['s-sn'] || slotNameAttr;
if (relocateNodeData) {
// previously we never found a slot home for this node
// but turns out we did, so let's remember it now
relocateNodeData.$slotRefNode$ = childNode;
}
else {
// add to our list of nodes to relocate
relocateNodes.push({
$slotRefNode$: childNode,
$nodeToRelocate$: node,
});
}
if (node['s-sr']) {
relocateNodes.map(relocateNode => {
if (isNodeLocatedInSlot(relocateNode.$nodeToRelocate$, node['s-sn'])) {
relocateNodeData = relocateNodes.find(r => r.$nodeToRelocate$ === node);
if (relocateNodeData && !relocateNode.$slotRefNode$) {
relocateNode.$slotRefNode$ = relocateNodeData.$slotRefNode$;
}
}
});
}
}
else if (!relocateNodes.some(r => r.$nodeToRelocate$ === node)) {
// so far this element does not have a slot home, not setting slotRefNode on purpose
// if we never find a home for this element then we'll need to hide it
relocateNodes.push({
$nodeToRelocate$: node,
});
}
}
}
}
if (childNode.nodeType === 1 /* ElementNode */) {
relocateSlotContent(childNode);
}
}
};
const isNodeLocatedInSlot = (nodeToRelocate, slotNameAttr) => {
if (nodeToRelocate.nodeType === 1 /* ElementNode */) {
if (nodeToRelocate.getAttribute('slot') === null && slotNameAttr === '') {
return true;
}
if (nodeToRelocate.getAttribute('slot') === slotNameAttr) {
return true;
}
return false;
}
if (nodeToRelocate['s-sn'] === slotNameAttr) {
return true;
}
return slotNameAttr === '';
};
const renderVdom = (hostRef, renderFnResults) => {
const hostElm = hostRef.$hostElement$;
const cmpMeta = hostRef.$cmpMeta$;
const oldVNode = hostRef.$vnode$ || newVNode(null, null);
const rootVnode = isHost(renderFnResults) ? renderFnResults : h(null, null, renderFnResults);
hostTagName = hostElm.tagName;
if ( cmpMeta.$attrsToReflect$) {
rootVnode.$attrs$ = rootVnode.$attrs$ || {};
cmpMeta.$attrsToReflect$.map(([propName, attribute]) => (rootVnode.$attrs$[attribute] = hostElm[propName]));
}
rootVnode.$tag$ = null;
rootVnode.$flags$ |= 4 /* isHost */;
hostRef.$vnode$ = rootVnode;
rootVnode.$elm$ = oldVNode.$elm$ = ( hostElm);
{
contentRef = hostElm['s-cr'];
useNativeShadowDom = (cmpMeta.$flags$ & 1 /* shadowDomEncapsulation */) !== 0;
// always reset
checkSlotFallbackVisibility = false;
}
// synchronous patch
patch(oldVNode, rootVnode);
{
// while we're moving nodes around existing nodes, temporarily disable
// the disconnectCallback from working
plt.$flags$ |= 1 /* isTmpDisconnected */;
if (checkSlotRelocate) {
relocateSlotContent(rootVnode.$elm$);
let relocateData;
let nodeToRelocate;
let orgLocationNode;
let parentNodeRef;
let insertBeforeNode;
let refNode;
let i = 0;
for (; i < relocateNodes.length; i++) {
relocateData = relocateNodes[i];
nodeToRelocate = relocateData.$nodeToRelocate$;
if (!nodeToRelocate['s-ol']) {
// add a reference node marking this node's original location
// keep a reference to this node for later lookups
orgLocationNode = doc.createTextNode('');
orgLocationNode['s-nr'] = nodeToRelocate;
nodeToRelocate.parentNode.insertBefore((nodeToRelocate['s-ol'] = orgLocationNode), nodeToRelocate);
}
}
for (i = 0; i < relocateNodes.length; i++) {
relocateData = relocateNodes[i];
nodeToRelocate = relocateData.$nodeToRelocate$;
if (relocateData.$slotRefNode$) {
// by default we're just going to insert it directly
// after the slot reference node
parentNodeRef = relocateData.$slotRefNode$.parentNode;
insertBeforeNode = relocateData.$slotRefNode$.nextSibling;
orgLocationNode = nodeToRelocate['s-ol'];
while ((orgLocationNode = orgLocationNode.previousSibling)) {
refNode = orgLocationNode['s-nr'];
if (refNode && refNode['s-sn'] === nodeToRelocate['s-sn'] && parentNodeRef === refNode.parentNode) {
refNode = refNode.nextSibling;
if (!refNode || !refNode['s-nr']) {
insertBeforeNode = refNode;
break;
}
}
}
if ((!insertBeforeNode && parentNodeRef !== nodeToRelocate.parentNode) || nodeToRelocate.nextSibling !== insertBeforeNode) {
// we've checked that it's worth while to relocate
// since that the node to relocate
// has a different next sibling or parent relocated
if (nodeToRelocate !== insertBeforeNode) {
if (!nodeToRelocate['s-hn'] && nodeToRelocate['s-ol']) {
// probably a component in the index.html that doesn't have it's hostname set
nodeToRelocate['s-hn'] = nodeToRelocate['s-ol'].parentNode.nodeName;
}
// add it back to the dom but in its new home
parentNodeRef.insertBefore(nodeToRelocate, insertBeforeNode);
}
}
}
else {
// this node doesn't have a slot home to go to, so let's hide it
if (nodeToRelocate.nodeType === 1 /* ElementNode */) {
nodeToRelocate.hidden = true;
}
}
}
}
if (checkSlotFallbackVisibility) {
updateFallbackSlotVisibility(rootVnode.$elm$);
}
// done moving nodes around
// allow the disconnect callback to work again
plt.$flags$ &= ~1 /* isTmpDisconnected */;
// always reset
relocateNodes.length = 0;
}
};
const emitEvent = (elm, name, opts) => {
const ev = plt.ce(name, opts);
elm.dispatchEvent(ev);
return ev;
};
const attachToAncestor = (hostRef, ancestorComponent) => {
if ( ancestorComponent && !hostRef.$onRenderResolve$ && ancestorComponent['s-p']) {
ancestorComponent['s-p'].push(new Promise(r => (hostRef.$onRenderResolve$ = r)));
}
};
const scheduleUpdate = (hostRef, isInitialLoad) => {
{
hostRef.$flags$ |= 16 /* isQueuedForUpdate */;
}
if ( hostRef.$flags$ & 4 /* isWaitingForChildren */) {
hostRef.$flags$ |= 512 /* needsRerender */;
return;
}
attachToAncestor(hostRef, hostRef.$ancestorComponent$);
// there is no ancestorc omponent or the ancestor component
// has already fired off its lifecycle update then
// fire off the initial update
const dispatch = () => dispatchHooks(hostRef, isInitialLoad);
return writeTask(dispatch) ;
};
const dispatchHooks = (hostRef, isInitialLoad) => {
const endSchedule = createTime('scheduleUpdate', hostRef.$cmpMeta$.$tagName$);
const instance = hostRef.$lazyInstance$ ;
let promise;
endSchedule();
return then(promise, () => updateComponent(hostRef, instance, isInitialLoad));
};
const updateComponent = (hostRef, instance, isInitialLoad) => {
// updateComponent
const elm = hostRef.$hostElement$;
const endUpdate = createTime('update', hostRef.$cmpMeta$.$tagName$);
const rc = elm['s-rc'];
if ( isInitialLoad) {
// DOM WRITE!
attachStyles(hostRef);
}
const endRender = createTime('render', hostRef.$cmpMeta$.$tagName$);
{
{
// looks like we've got child nodes to render into this host element
// or we need to update the css class/attrs on the host element
// DOM WRITE!
renderVdom(hostRef, callRender(hostRef, instance));
}
}
if ( plt.$cssShim$) {
plt.$cssShim$.updateHost(elm);
}
if ( rc) {
// ok, so turns out there are some child host elements
// waiting on this parent element to load
// let's fire off all update callbacks waiting
rc.map(cb => cb());
elm['s-rc'] = undefined;
}
endRender();
endUpdate();
{
const childrenPromises = elm['s-p'];
const postUpdate = () => postUpdateComponent(hostRef);
if (childrenPromises.length === 0) {
postUpdate();
}
else {
Promise.all(childrenPromises).then(postUpdate);
hostRef.$flags$ |= 4 /* isWaitingForChildren */;
childrenPromises.length = 0;
}
}
};
const callRender = (hostRef, instance) => {
try {
instance = instance.render() ;
{
hostRef.$flags$ &= ~16 /* isQueuedForUpdate */;
}
{
hostRef.$flags$ |= 2 /* hasRendered */;
}
}
catch (e) {
consoleError(e);
}
return instance;
};
const postUpdateComponent = (hostRef) => {
const tagName = hostRef.$cmpMeta$.$tagName$;
const elm = hostRef.$hostElement$;
const endPostUpdate = createTime('postUpdate', tagName);
const ancestorComponent = hostRef.$ancestorComponent$;
if (!(hostRef.$flags$ & 64 /* hasLoadedComponent */)) {
hostRef.$flags$ |= 64 /* hasLoadedComponent */;
{
// DOM WRITE!
addHydratedFlag(elm);
}
endPostUpdate();
{
hostRef.$onReadyResolve$(elm);
if (!ancestorComponent) {
appDidLoad();
}
}
}
else {
endPostUpdate();
}
// load events fire from bottom to top
// the deepest elements load first then bubbles up
{
if (hostRef.$onRenderResolve$) {
hostRef.$onRenderResolve$();
hostRef.$onRenderResolve$ = undefined;
}
if (hostRef.$flags$ & 512 /* needsRerender */) {
nextTick(() => scheduleUpdate(hostRef, false));
}
hostRef.$flags$ &= ~(4 /* isWaitingForChildren */ | 512 /* needsRerender */);
}
// ( •_•)
// ( •_•)>⌐■-■
// (⌐■_■)
};
const forceUpdate = (ref) => {
{
const hostRef = getHostRef(ref);
const isConnected = hostRef.$hostElement$.isConnected;
if (isConnected && (hostRef.$flags$ & (2 /* hasRendered */ | 16 /* isQueuedForUpdate */)) === 2 /* hasRendered */) {
scheduleUpdate(hostRef, false);
}
// Returns "true" when the forced update was successfully scheduled
return isConnected;
}
};
const appDidLoad = (who) => {
// on appload
// we have finish the first big initial render
{
addHydratedFlag(doc.documentElement);
}
{
plt.$flags$ |= 2 /* appLoaded */;
}
nextTick(() => emitEvent(win, 'appload', { detail: { namespace: NAMESPACE } }));
};
const then = (promise, thenFn) => {
return promise && promise.then ? promise.then(thenFn) : thenFn();
};
const addHydratedFlag = (elm) => ( elm.classList.add('hydrated') );
const initializeClientHydrate = (hostElm, tagName, hostId, hostRef) => {
const endHydrate = createTime('hydrateClient', tagName);
const shadowRoot = hostElm.shadowRoot;
const childRenderNodes = [];
const slotNodes = [];
const shadowRootNodes = null;
const vnode = (hostRef.$vnode$ = newVNode(tagName, null));
if (!plt.$orgLocNodes$) {
initializeDocumentHydrate(doc.body, (plt.$orgLocNodes$ = new Map()));
}
hostElm[HYDRATE_ID] = hostId;
hostElm.removeAttribute(HYDRATE_ID);
clientHydrate(vnode, childRenderNodes, slotNodes, shadowRootNodes, hostElm, hostElm, hostId);
childRenderNodes.map(c => {
const orgLocationId = c.$hostId$ + '.' + c.$nodeId$;
const orgLocationNode = plt.$orgLocNodes$.get(orgLocationId);
const node = c.$elm$;
if (orgLocationNode && supportsShadow && orgLocationNode['s-en'] === '') {
orgLocationNode.parentNode.insertBefore(node, orgLocationNode.nextSibling);
}
if (!shadowRoot) {
node['s-hn'] = tagName;
if (orgLocationNode) {
node['s-ol'] = orgLocationNode;
node['s-ol']['s-nr'] = node;
}
}
plt.$orgLocNodes$.delete(orgLocationId);
});
endHydrate();
};
const clientHydrate = (parentVNode, childRenderNodes, slotNodes, shadowRootNodes, hostElm, node, hostId) => {
let childNodeType;
let childIdSplt;
let childVNode;
let i;
if (node.nodeType === 1 /* ElementNode */) {
childNodeType = node.getAttribute(HYDRATE_CHILD_ID);
if (childNodeType) {
// got the node data from the element's attribute
// `${hostId}.${nodeId}.${depth}.${index}`
childIdSplt = childNodeType.split('.');
if (childIdSplt[0] === hostId || childIdSplt[0] === '0') {
childVNode = {
$flags$: 0,
$hostId$: childIdSplt[0],
$nodeId$: childIdSplt[1],
$depth$: childIdSplt[2],
$index$: childIdSplt[3],
$tag$: node.tagName.toLowerCase(),
$elm$: node,
$attrs$: null,
$children$: null,
$key$: null,
$name$: null,
$text$: null,
};
childRenderNodes.push(childVNode);
node.removeAttribute(HYDRATE_CHILD_ID);
// this is a new child vnode
// so ensure its parent vnode has the vchildren array
if (!parentVNode.$children$) {
parentVNode.$children$ = [];
}
// add our child vnode to a specific index of the vnode's children
parentVNode.$children$[childVNode.$index$] = childVNode;
// this is now the new parent vnode for all the next child checks
parentVNode = childVNode;
if (shadowRootNodes && childVNode.$depth$ === '0') {
shadowRootNodes[childVNode.$index$] = childVNode.$elm$;
}
}
}
// recursively drill down, end to start so we can remove nodes
for (i = node.childNodes.length - 1; i >= 0; i--) {
clientHydrate(parentVNode, childRenderNodes, slotNodes, shadowRootNodes, hostElm, node.childNodes[i], hostId);
}
if (node.shadowRoot) {
// keep drilling down through the shadow root nodes
for (i = node.shadowRoot.childNodes.length - 1; i >= 0; i--) {
clientHydrate(parentVNode, childRenderNodes, slotNodes, shadowRootNodes, hostElm, node.shadowRoot.childNodes[i], hostId);
}
}
}
else if (node.nodeType === 8 /* CommentNode */) {
// `${COMMENT_TYPE}.${hostId}.${nodeId}.${depth}.${index}`
childIdSplt = node.nodeValue.split('.');
if (childIdSplt[1] === hostId || childIdSplt[1] === '0') {
// comment node for either the host id or a 0 host id
childNodeType = childIdSplt[0];
childVNode = {
$flags$: 0,
$hostId$: childIdSplt[1],
$nodeId$: childIdSplt[2],
$depth$: childIdSplt[3],
$index$: childIdSplt[4],
$elm$: node,
$attrs$: null,
$children$: null,
$key$: null,
$name$: null,
$tag$: null,
$text$: null,
};
if (childNodeType === TEXT_NODE_ID) {
childVNode.$elm$ = node.nextSibling;
if (childVNode.$elm$ && childVNode.$elm$.nodeType === 3 /* TextNode */) {
childVNode.$text$ = childVNode.$elm$.textContent;
childRenderNodes.push(childVNode);
// remove the text comment since it's no longer needed
node.remove();
if (!parentVNode.$children$) {
parentVNode.$children$ = [];
}
parentVNode.$children$[childVNode.$index$] = childVNode;
if (shadowRootNodes && childVNode.$depth$ === '0') {
shadowRootNodes[childVNode.$index$] = childVNode.$elm$;
}
}
}
else if (childVNode.$hostId$ === hostId) {
// this comment node is specifcally for this host id
if (childNodeType === SLOT_NODE_ID) {
// `${SLOT_NODE_ID}.${hostId}.${nodeId}.${depth}.${index}.${slotName}`;
childVNode.$tag$ = 'slot';
if (childIdSplt[5]) {
node['s-sn'] = childVNode.$name$ = childIdSplt[5];
}
else {
node['s-sn'] = '';
}
node['s-sr'] = true;
slotNodes.push(childVNode);
if (!parentVNode.$children$) {
parentVNode.$children$ = [];
}
parentVNode.$children$[childVNode.$index$] = childVNode;
}
else if (childNodeType === CONTENT_REF_ID) {
// `${CONTENT_REF_ID}.${hostId}`;
{
hostElm['s-cr'] = node;
node['s-cn'] = true;
}
}
}
}
}
else if (parentVNode && parentVNode.$tag$ === 'style') {
const vnode = newVNode(null, node.textContent);
vnode.$elm$ = node;
vnode.$index$ = '0';
parentVNode.$children$ = [vnode];
}
};
const initializeDocumentHydrate = (node, orgLocNodes) => {
if (node.nodeType === 1 /* ElementNode */) {
let i = 0;
for (; i < node.childNodes.length; i++) {
initializeDocumentHydrate(node.childNodes[i], orgLocNodes);
}
if (node.shadowRoot) {
for (i = 0; i < node.shadowRoot.childNodes.length; i++) {
initializeDocumentHydrate(node.shadowRoot.childNodes[i], orgLocNodes);
}
}
}
else if (node.nodeType === 8 /* CommentNode */) {
const childIdSplt = node.nodeValue.split('.');
if (childIdSplt[0] === ORG_LOCATION_ID) {
orgLocNodes.set(childIdSplt[1] + '.' + childIdSplt[2], node);
node.nodeValue = '';
// useful to know if the original location is
// the root light-dom of a shadow dom component
node['s-en'] = childIdSplt[3];
}
}
};
const parsePropertyValue = (propValue, propType) => {
// ensure this value is of the correct prop type
if (propValue