bulmil
Version:
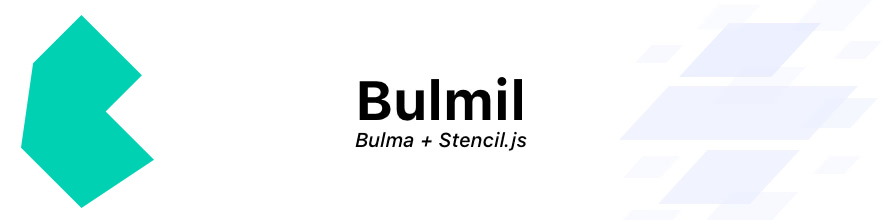
876 lines (875 loc) • 165 kB
JavaScript
var __extends = (this && this.__extends) || (function () {
var extendStatics = function (d, b) {
extendStatics = Object.setPrototypeOf ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||
function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; };
return extendStatics(d, b);
};
return function (d, b) {
extendStatics(d, b);
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
})();
var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) {
function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }
return new (P || (P = Promise))(function (resolve, reject) {
function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }
function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } }
function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
};
var __generator = (this && this.__generator) || function (thisArg, body) {
var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g;
return g = { next: verb(0), "throw": verb(1), "return": verb(2) }, typeof Symbol === "function" && (g[Symbol.iterator] = function() { return this; }), g;
function verb(n) { return function (v) { return step([n, v]); }; }
function step(op) {
if (f) throw new TypeError("Generator is already executing.");
while (_) try {
if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done) return t;
if (y = 0, t) op = [op[0] & 2, t.value];
switch (op[0]) {
case 0: case 1: t = op; break;
case 4: _.label++; return { value: op[1], done: false };
case 5: _.label++; y = op[1]; op = [0]; continue;
case 7: op = _.ops.pop(); _.trys.pop(); continue;
default:
if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; }
if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; }
if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; }
if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; }
if (t[2]) _.ops.pop();
_.trys.pop(); continue;
}
op = body.call(thisArg, _);
} catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; }
if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true };
}
};
var __spreadArrays = (this && this.__spreadArrays) || function () {
for (var s = 0, i = 0, il = arguments.length; i < il; i++) s += arguments[i].length;
for (var r = Array(s), k = 0, i = 0; i < il; i++)
for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)
r[k] = a[j];
return r;
};
System.register([], function (exports, module) {
'use strict';
return {
execute: function () {
var _this = this;
var NAMESPACE = exports('N', 'bulmil');
var BUILD = exports('B', /* bulmil */ { allRenderFn: true, appendChildSlotFix: false, asyncLoading: true, asyncQueue: true, attachStyles: true, cloneNodeFix: false, cmpDidLoad: false, cmpDidRender: false, cmpDidUnload: false, cmpDidUpdate: false, cmpShouldUpdate: false, cmpWillLoad: false, cmpWillRender: false, cmpWillUpdate: false, connectedCallback: false, constructableCSS: true, cssAnnotations: true, cssVarShim: true, devTools: false, disconnectedCallback: false, dynamicImportShim: true, element: false, event: false, hasRenderFn: true, hostListener: false, hostListenerTarget: false, hostListenerTargetBody: false, hostListenerTargetDocument: false, hostListenerTargetParent: false, hostListenerTargetWindow: false, hotModuleReplacement: false, hydrateClientSide: true, hydrateServerSide: false, hydratedAttribute: false, hydratedClass: true, initializeNextTick: true, isDebug: false, isDev: true, isTesting: true, lazyLoad: true, lifecycle: false, lifecycleDOMEvents: true, member: true, method: false, mode: false, observeAttribute: true, profile: false, prop: true, propBoolean: true, propMutable: true, propNumber: true, propString: true, reflect: true, safari10: true, scoped: false, scriptDataOpts: true, shadowDelegatesFocus: false, shadowDom: false, shadowDomShim: true, slot: true, slotChildNodesFix: false, slotRelocation: true, state: false, style: true, svg: false, taskQueue: true, transformTagName: false, updatable: true, vdomAttribute: true, vdomClass: true, vdomFunctional: true, vdomKey: false, vdomListener: true, vdomPropOrAttr: true, vdomRef: false, vdomRender: true, vdomStyle: false, vdomText: true, vdomXlink: false, watchCallback: false });
var scopeId;
var contentRef;
var hostTagName;
var i = 0;
var useNativeShadowDom = false;
var checkSlotFallbackVisibility = false;
var checkSlotRelocate = false;
var isSvgMode = false;
var renderingRef = null;
var queueCongestion = 0;
var queuePending = false;
var win = exports('w', typeof window !== 'undefined' ? window : {});
var CSS = exports('C', BUILD.cssVarShim ? win.CSS : null);
var doc = exports('d', win.document || { head: {} });
var H = exports('H', (win.HTMLElement || /** @class */ (function () {
function HTMLElement() {
}
return HTMLElement;
}())));
var plt = exports('p', {
$flags$: 0,
$resourcesUrl$: '',
jmp: function (h) { return h(); },
raf: function (h) { return requestAnimationFrame(h); },
ael: function (el, eventName, listener, opts) { return el.addEventListener(eventName, listener, opts); },
rel: function (el, eventName, listener, opts) { return el.removeEventListener(eventName, listener, opts); },
ce: function (eventName, opts) { return new CustomEvent(eventName, opts); },
});
var supportsShadow = BUILD.shadowDomShim && BUILD.shadowDom ? /*@__PURE__*/ (function () { return (doc.head.attachShadow + '').indexOf('[native') > -1; })() : true;
var supportsListenerOptions = /*@__PURE__*/ (function () {
var supportsListenerOptions = false;
try {
doc.addEventListener('e', null, Object.defineProperty({}, 'passive', {
get: function () {
supportsListenerOptions = true;
},
}));
}
catch (e) { }
return supportsListenerOptions;
})();
var promiseResolve = exports('a', function (v) { return Promise.resolve(v); });
var supportsConstructibleStylesheets = BUILD.constructableCSS
? /*@__PURE__*/ (function () {
try {
new CSSStyleSheet();
return true;
}
catch (e) { }
return false;
})()
: false;
var Context = {};
var addHostEventListeners = function (elm, hostRef, listeners, attachParentListeners) {
if (BUILD.hostListener && listeners) {
// this is called immediately within the element's constructor
// initialize our event listeners on the host element
// we do this now so that we can listen to events that may
// have fired even before the instance is ready
if (BUILD.hostListenerTargetParent) {
// this component may have event listeners that should be attached to the parent
if (attachParentListeners) {
// this is being ran from within the connectedCallback
// which is important so that we know the host element actually has a parent element
// filter out the listeners to only have the ones that ARE being attached to the parent
listeners = listeners.filter(function (_a) {
var flags = _a[0];
return flags & 16;
} /* TargetParent */);
}
else {
// this is being ran from within the component constructor
// everything BUT the parent element listeners should be attached at this time
// filter out the listeners that are NOT being attached to the parent
listeners = listeners.filter(function (_a) {
var flags = _a[0];
return !(flags & 16 /* TargetParent */);
});
}
}
listeners.map(function (_a) {
var flags = _a[0], name = _a[1], method = _a[2];
var target = BUILD.hostListenerTarget ? getHostListenerTarget(elm, flags) : elm;
var handler = hostListenerProxy(hostRef, method);
var opts = hostListenerOpts(flags);
plt.ael(target, name, handler, opts);
(hostRef.$rmListeners$ = hostRef.$rmListeners$ || []).push(function () { return plt.rel(target, name, handler, opts); });
});
}
};
var hostListenerProxy = function (hostRef, methodName) { return function (ev) {
if (BUILD.lazyLoad) {
if (hostRef.$flags$ & 256 /* isListenReady */) {
// instance is ready, let's call it's member method for this event
hostRef.$lazyInstance$[methodName](ev);
}
else {
(hostRef.$queuedListeners$ = hostRef.$queuedListeners$ || []).push([methodName, ev]);
}
}
else {
hostRef.$hostElement$[methodName](ev);
}
}; };
var getHostListenerTarget = function (elm, flags) {
if (BUILD.hostListenerTargetDocument && flags & 4 /* TargetDocument */)
return doc;
if (BUILD.hostListenerTargetWindow && flags & 8 /* TargetWindow */)
return win;
if (BUILD.hostListenerTargetBody && flags & 32 /* TargetBody */)
return doc.body;
if (BUILD.hostListenerTargetParent && flags & 16 /* TargetParent */)
return elm.parentElement;
return elm;
};
// prettier-ignore
var hostListenerOpts = function (flags) { return supportsListenerOptions
? ({
passive: (flags & 1 /* Passive */) !== 0,
capture: (flags & 2 /* Capture */) !== 0,
})
: (flags & 2 /* Capture */) !== 0; };
var CONTENT_REF_ID = 'r';
var ORG_LOCATION_ID = 'o';
var SLOT_NODE_ID = 's';
var TEXT_NODE_ID = 't';
var HYDRATE_ID = 's-id';
var HYDRATED_STYLE_ID = 'sty-id';
var HYDRATE_CHILD_ID = 'c-id';
var HYDRATED_CSS = '{visibility:hidden}.hydrated{visibility:inherit}';
var XLINK_NS = 'http://www.w3.org/1999/xlink';
var createTime = function (fnName, tagName) {
if (tagName === void 0) { tagName = ''; }
if (BUILD.profile && performance.mark) {
var key_1 = "st:" + fnName + ":" + tagName + ":" + i++;
// Start
performance.mark(key_1);
// End
return function () { return performance.measure("[Stencil] " + fnName + "() <" + tagName + ">", key_1); };
}
else {
return function () {
return;
};
}
};
var uniqueTime = function (key, measureText) {
if (BUILD.profile && performance.mark) {
if (performance.getEntriesByName(key).length === 0) {
performance.mark(key);
}
return function () {
if (performance.getEntriesByName(measureText).length === 0) {
performance.measure(measureText, key);
}
};
}
else {
return function () {
return;
};
}
};
var inspect = function (ref) {
var _a;
var hostRef = getHostRef(ref);
if (!hostRef) {
return undefined;
}
var flags = hostRef.$flags$;
var hostElement = hostRef.$hostElement$;
return _a = {
renderCount: hostRef.$renderCount$,
flags: {
hasRendered: !!(flags & 2 /* hasRendered */),
hasConnected: !!(flags & 1 /* hasConnected */),
isWaitingForChildren: !!(flags & 4 /* isWaitingForChildren */),
isConstructingInstance: !!(flags & 8 /* isConstructingInstance */),
isQueuedForUpdate: !!(flags & 16 /* isQueuedForUpdate */),
hasInitializedComponent: !!(flags & 32 /* hasInitializedComponent */),
hasLoadedComponent: !!(flags & 64 /* hasLoadedComponent */),
isWatchReady: !!(flags & 128 /* isWatchReady */),
isListenReady: !!(flags & 256 /* isListenReady */),
needsRerender: !!(flags & 512 /* needsRerender */),
},
instanceValues: hostRef.$instanceValues$,
ancestorComponent: hostRef.$ancestorComponent$,
hostElement: hostElement,
lazyInstance: hostRef.$lazyInstance$,
vnode: hostRef.$vnode$,
modeName: hostRef.$modeName$,
onReadyPromise: hostRef.$onReadyPromise$,
onReadyResolve: hostRef.$onReadyResolve$,
onInstancePromise: hostRef.$onInstancePromise$,
onInstanceResolve: hostRef.$onInstanceResolve$,
onRenderResolve: hostRef.$onRenderResolve$,
queuedListeners: hostRef.$queuedListeners$,
rmListeners: hostRef.$rmListeners$
},
_a['s-id'] = hostElement['s-id'],
_a['s-cr'] = hostElement['s-cr'],
_a['s-lr'] = hostElement['s-lr'],
_a['s-p'] = hostElement['s-p'],
_a['s-rc'] = hostElement['s-rc'],
_a['s-sc'] = hostElement['s-sc'],
_a;
};
var installDevTools = function () {
if (BUILD.devTools) {
var stencil = (win.stencil = win.stencil || {});
var originalInspect_1 = stencil.inspect;
stencil.inspect = function (ref) {
var result = inspect(ref);
if (!result && typeof originalInspect_1 === 'function') {
result = originalInspect_1(ref);
}
return result;
};
}
};
var rootAppliedStyles = new WeakMap();
var registerStyle = function (scopeId, cssText, allowCS) {
var style = styles.get(scopeId);
if (supportsConstructibleStylesheets && allowCS) {
style = (style || new CSSStyleSheet());
style.replace(cssText);
}
else {
style = cssText;
}
styles.set(scopeId, style);
};
var addStyle = function (styleContainerNode, cmpMeta, mode, hostElm) {
var scopeId = getScopeId(cmpMeta, mode);
var style = styles.get(scopeId);
if (!BUILD.attachStyles) {
return scopeId;
}
// if an element is NOT connected then getRootNode() will return the wrong root node
// so the fallback is to always use the document for the root node in those cases
styleContainerNode = styleContainerNode.nodeType === 11 /* DocumentFragment */ ? styleContainerNode : doc;
if (style) {
if (typeof style === 'string') {
styleContainerNode = styleContainerNode.head || styleContainerNode;
var appliedStyles = rootAppliedStyles.get(styleContainerNode);
var styleElm = void 0;
if (!appliedStyles) {
rootAppliedStyles.set(styleContainerNode, (appliedStyles = new Set()));
}
if (!appliedStyles.has(scopeId)) {
if (BUILD.hydrateClientSide && styleContainerNode.host && (styleElm = styleContainerNode.querySelector("[" + HYDRATED_STYLE_ID + "=\"" + scopeId + "\"]"))) {
// This is only happening on native shadow-dom, do not needs CSS var shim
styleElm.innerHTML = style;
}
else {
if (BUILD.cssVarShim && plt.$cssShim$) {
styleElm = plt.$cssShim$.createHostStyle(hostElm, scopeId, style, !!(cmpMeta.$flags$ & 10 /* needsScopedEncapsulation */));
var newScopeId = styleElm['s-sc'];
if (newScopeId) {
scopeId = newScopeId;
// we don't want to add this styleID to the appliedStyles Set
// since the cssVarShim might need to apply several different
// stylesheets for the same component
appliedStyles = null;
}
}
else {
styleElm = doc.createElement('style');
styleElm.innerHTML = style;
}
if (BUILD.hydrateServerSide || BUILD.hotModuleReplacement) {
styleElm.setAttribute(HYDRATED_STYLE_ID, scopeId);
}
styleContainerNode.insertBefore(styleElm, styleContainerNode.querySelector('link'));
}
if (appliedStyles) {
appliedStyles.add(scopeId);
}
}
}
else if (BUILD.constructableCSS && !styleContainerNode.adoptedStyleSheets.includes(style)) {
styleContainerNode.adoptedStyleSheets = __spreadArrays(styleContainerNode.adoptedStyleSheets, [style]);
}
}
return scopeId;
};
var attachStyles = function (hostRef) {
var cmpMeta = hostRef.$cmpMeta$;
var elm = hostRef.$hostElement$;
var flags = cmpMeta.$flags$;
var endAttachStyles = createTime('attachStyles', cmpMeta.$tagName$);
var scopeId = addStyle(BUILD.shadowDom && supportsShadow && elm.shadowRoot ? elm.shadowRoot : elm.getRootNode(), cmpMeta, hostRef.$modeName$, elm);
if ((BUILD.shadowDom || BUILD.scoped) && BUILD.cssAnnotations && flags & 10 /* needsScopedEncapsulation */) {
// only required when we're NOT using native shadow dom (slot)
// or this browser doesn't support native shadow dom
// and this host element was NOT created with SSR
// let's pick out the inner content for slot projection
// create a node to represent where the original
// content was first placed, which is useful later on
// DOM WRITE!!
elm['s-sc'] = scopeId;
elm.classList.add(scopeId + '-h');
if (BUILD.scoped && flags & 2 /* scopedCssEncapsulation */) {
elm.classList.add(scopeId + '-s');
}
}
endAttachStyles();
};
var getScopeId = function (cmp, mode) { return 'sc-' + (BUILD.mode && mode && cmp.$flags$ & 32 /* hasMode */ ? cmp.$tagName$ + '-' + mode : cmp.$tagName$); };
var convertScopedToShadow = function (css) { return css.replace(/\/\*!@([^\/]+)\*\/[^\{]+\{/g, '$1{'); };
// Private
var computeMode = function (elm) { return modeResolutionChain.map(function (h) { return h(elm); }).find(function (m) { return !!m; }); };
// Public
var setMode = function (handler) { return modeResolutionChain.push(handler); };
var getMode = function (ref) { return getHostRef(ref).$modeName$; };
/**
* Default style mode id
*/
/**
* Reusable empty obj/array
* Don't add values to these!!
*/
var EMPTY_OBJ = {};
/**
* Namespaces
*/
var SVG_NS = 'http://www.w3.org/2000/svg';
var HTML_NS = 'http://www.w3.org/1999/xhtml';
var isDef = function (v) { return v != null; };
var noop = function () {
/* noop*/
};
var isComplexType = function (o) {
// https://jsperf.com/typeof-fn-object/5
o = typeof o;
return o === 'object' || o === 'function';
};
var IS_DENO_ENV = typeof Deno !== 'undefined';
var IS_NODE_ENV = !IS_DENO_ENV &&
typeof global !== 'undefined' &&
typeof require === 'function' &&
!!global.process &&
typeof __filename === 'string' &&
(!global.origin || typeof global.origin !== 'string');
var IS_DENO_WINDOWS_ENV = IS_DENO_ENV && Deno.build.os === 'windows';
var getCurrentDirectory = IS_NODE_ENV ? process.cwd : IS_DENO_ENV ? Deno.cwd : function () { return '/'; };
var exit = IS_NODE_ENV ? process.exit : IS_DENO_ENV ? Deno.exit : noop;
/**
* Production h() function based on Preact by
* Jason Miller (@developit)
* Licensed under the MIT License
* https://github.com/developit/preact/blob/master/LICENSE
*
* Modified for Stencil's compiler and vdom
*/
// const stack: any[] = [];
// export function h(nodeName: string | d.FunctionalComponent, vnodeData: d.PropsType, child?: d.ChildType): d.VNode;
// export function h(nodeName: string | d.FunctionalComponent, vnodeData: d.PropsType, ...children: d.ChildType[]): d.VNode;
var h = exports('h', function (nodeName, vnodeData) {
var children = [];
for (var _i = 2; _i < arguments.length; _i++) {
children[_i - 2] = arguments[_i];
}
var child = null;
var key = null;
var slotName = null;
var simple = false;
var lastSimple = false;
var vNodeChildren = [];
var walk = function (c) {
for (var i_1 = 0; i_1 < c.length; i_1++) {
child = c[i_1];
if (Array.isArray(child)) {
walk(child);
}
else if (child != null && typeof child !== 'boolean') {
if ((simple = typeof nodeName !== 'function' && !isComplexType(child))) {
child = String(child);
}
else if (BUILD.isDev && typeof nodeName !== 'function' && child.$flags$ === undefined) {
consoleDevError("vNode passed as children has unexpected type.\nMake sure it's using the correct h() function.\nEmpty objects can also be the cause, look for JSX comments that became objects.");
}
if (simple && lastSimple) {
// If the previous child was simple (string), we merge both
vNodeChildren[vNodeChildren.length - 1].$text$ += child;
}
else {
// Append a new vNode, if it's text, we create a text vNode
vNodeChildren.push(simple ? newVNode(null, child) : child);
}
lastSimple = simple;
}
}
};
walk(children);
if (vnodeData) {
if (BUILD.isDev && nodeName === 'input') {
validateInputProperties(vnodeData);
}
// normalize class / classname attributes
if (BUILD.vdomKey && vnodeData.key) {
key = vnodeData.key;
}
if (BUILD.slotRelocation && vnodeData.name) {
slotName = vnodeData.name;
}
if (BUILD.vdomClass) {
var classData_1 = vnodeData.className || vnodeData.class;
if (classData_1) {
vnodeData.class =
typeof classData_1 !== 'object'
? classData_1
: Object.keys(classData_1)
.filter(function (k) { return classData_1[k]; })
.join(' ');
}
}
}
if (BUILD.isDev && vNodeChildren.some(isHost)) {
consoleDevError("The <Host> must be the single root component. Make sure:\n- You are NOT using hostData() and <Host> in the same component.\n- <Host> is used once, and it's the single root component of the render() function.");
}
if (BUILD.vdomFunctional && typeof nodeName === 'function') {
// nodeName is a functional component
return nodeName(vnodeData === null ? {} : vnodeData, vNodeChildren, vdomFnUtils);
}
var vnode = newVNode(nodeName, null);
vnode.$attrs$ = vnodeData;
if (vNodeChildren.length > 0) {
vnode.$children$ = vNodeChildren;
}
if (BUILD.vdomKey) {
vnode.$key$ = key;
}
if (BUILD.slotRelocation) {
vnode.$name$ = slotName;
}
return vnode;
});
var newVNode = function (tag, text) {
var vnode = {
$flags$: 0,
$tag$: tag,
$text$: text,
$elm$: null,
$children$: null,
};
if (BUILD.vdomAttribute) {
vnode.$attrs$ = null;
}
if (BUILD.vdomKey) {
vnode.$key$ = null;
}
if (BUILD.slotRelocation) {
vnode.$name$ = null;
}
return vnode;
};
var Host = exports('e', {});
var isHost = function (node) { return node && node.$tag$ === Host; };
var vdomFnUtils = {
forEach: function (children, cb) { return children.map(convertToPublic).forEach(cb); },
map: function (children, cb) { return children
.map(convertToPublic)
.map(cb)
.map(convertToPrivate); },
};
var convertToPublic = function (node) { return ({
vattrs: node.$attrs$,
vchildren: node.$children$,
vkey: node.$key$,
vname: node.$name$,
vtag: node.$tag$,
vtext: node.$text$,
}); };
var convertToPrivate = function (node) {
if (typeof node.vtag === 'function') {
var vnodeData = Object.assign({}, node.vattrs);
if (node.vkey) {
vnodeData.key = node.vkey;
}
if (node.vname) {
vnodeData.name = node.vname;
}
return h.apply(void 0, __spreadArrays([node.vtag, vnodeData], node.vchildren || []));
}
var vnode = newVNode(node.vtag, node.vtext);
vnode.$attrs$ = node.vattrs;
vnode.$children$ = node.vchildren;
vnode.$key$ = node.vkey;
vnode.$name$ = node.vname;
return vnode;
};
var validateInputProperties = function (vnodeData) {
var props = Object.keys(vnodeData);
var typeIndex = props.indexOf('type');
var minIndex = props.indexOf('min');
var maxIndex = props.indexOf('max');
var stepIndex = props.indexOf('min');
var value = props.indexOf('value');
if (value === -1) {
return;
}
if (value < typeIndex || value < minIndex || value < maxIndex || value < stepIndex) {
consoleDevWarn("The \"value\" prop of <input> should be set after \"min\", \"max\", \"type\" and \"step\"");
}
};
/**
* Production setAccessor() function based on Preact by
* Jason Miller (@developit)
* Licensed under the MIT License
* https://github.com/developit/preact/blob/master/LICENSE
*
* Modified for Stencil's compiler and vdom
*/
var setAccessor = function (elm, memberName, oldValue, newValue, isSvg, flags) {
if (oldValue !== newValue) {
var isProp = isMemberInElement(elm, memberName);
var ln = memberName.toLowerCase();
if (BUILD.vdomClass && memberName === 'class') {
var classList = elm.classList;
var oldClasses_1 = parseClassList(oldValue);
var newClasses_1 = parseClassList(newValue);
classList.remove.apply(classList, oldClasses_1.filter(function (c) { return c && !newClasses_1.includes(c); }));
classList.add.apply(classList, newClasses_1.filter(function (c) { return c && !oldClasses_1.includes(c); }));
}
else if (BUILD.vdomStyle && memberName === 'style') {
// update style attribute, css properties and values
if (BUILD.updatable) {
for (var prop in oldValue) {
if (!newValue || newValue[prop] == null) {
if (!BUILD.hydrateServerSide && prop.includes('-')) {
elm.style.removeProperty(prop);
}
else {
elm.style[prop] = '';
}
}
}
}
for (var prop in newValue) {
if (!oldValue || newValue[prop] !== oldValue[prop]) {
if (!BUILD.hydrateServerSide && prop.includes('-')) {
elm.style.setProperty(prop, newValue[prop]);
}
else {
elm.style[prop] = newValue[prop];
}
}
}
}
else if (BUILD.vdomKey && memberName === 'key')
;
else if (BUILD.vdomRef && memberName === 'ref') {
// minifier will clean this up
if (newValue) {
newValue(elm);
}
}
else if (BUILD.vdomListener && (BUILD.lazyLoad ? !isProp : !elm.__lookupSetter__(memberName)) && memberName[0] === 'o' && memberName[1] === 'n') {
// Event Handlers
// so if the member name starts with "on" and the 3rd characters is
// a capital letter, and it's not already a member on the element,
// then we're assuming it's an event listener
if (memberName[2] === '-') {
// on- prefixed events
// allows to be explicit about the dom event to listen without any magic
// under the hood:
// <my-cmp on-click> // listens for "click"
// <my-cmp on-Click> // listens for "Click"
// <my-cmp on-ionChange> // listens for "ionChange"
// <my-cmp on-EVENTS> // listens for "EVENTS"
memberName = memberName.slice(3);
}
else if (isMemberInElement(win, ln)) {
// standard event
// the JSX attribute could have been "onMouseOver" and the
// member name "onmouseover" is on the window's prototype
// so let's add the listener "mouseover", which is all lowercased
memberName = ln.slice(2);
}
else {
// custom event
// the JSX attribute could have been "onMyCustomEvent"
// so let's trim off the "on" prefix and lowercase the first character
// and add the listener "myCustomEvent"
// except for the first character, we keep the event name case
memberName = ln[2] + memberName.slice(3);
}
if (oldValue) {
plt.rel(elm, memberName, oldValue, false);
}
if (newValue) {
plt.ael(elm, memberName, newValue, false);
}
}
else if (BUILD.vdomPropOrAttr) {
// Set property if it exists and it's not a SVG
var isComplex = isComplexType(newValue);
if ((isProp || (isComplex && newValue !== null)) && !isSvg) {
try {
if (!elm.tagName.includes('-')) {
var n = newValue == null ? '' : newValue;
// Workaround for Safari, moving the <input> caret when re-assigning the same valued
if (memberName === 'list') {
isProp = false;
// tslint:disable-next-line: triple-equals
}
else if (oldValue == null || elm[memberName] != n) {
elm[memberName] = n;
}
}
else {
elm[memberName] = newValue;
}
}
catch (e) { }
}
/**
* Need to manually update attribute if:
* - memberName is not an attribute
* - if we are rendering the host element in order to reflect attribute
* - if it's a SVG, since properties might not work in <svg>
* - if the newValue is null/undefined or 'false'.
*/
var xlink = false;
if (BUILD.vdomXlink) {
if (ln !== (ln = ln.replace(/^xlink\:?/, ''))) {
memberName = ln;
xlink = true;
}
}
if (newValue == null || newValue === false) {
if (newValue !== false || elm.getAttribute(memberName) === '') {
if (BUILD.vdomXlink && xlink) {
elm.removeAttributeNS(XLINK_NS, memberName);
}
else {
elm.removeAttribute(memberName);
}
}
}
else if ((!isProp || flags & 4 /* isHost */ || isSvg) && !isComplex) {
newValue = newValue === true ? '' : newValue;
if (BUILD.vdomXlink && xlink) {
elm.setAttributeNS(XLINK_NS, memberName, newValue);
}
else {
elm.setAttribute(memberName, newValue);
}
}
}
}
};
var parseClassListRegex = /\s/;
var parseClassList = function (value) { return (!value ? [] : value.split(parseClassListRegex)); };
var updateElement = function (oldVnode, newVnode, isSvgMode, memberName) {
// if the element passed in is a shadow root, which is a document fragment
// then we want to be adding attrs/props to the shadow root's "host" element
// if it's not a shadow root, then we add attrs/props to the same element
var elm = newVnode.$elm$.nodeType === 11 /* DocumentFragment */ && newVnode.$elm$.host ? newVnode.$elm$.host : newVnode.$elm$;
var oldVnodeAttrs = (oldVnode && oldVnode.$attrs$) || EMPTY_OBJ;
var newVnodeAttrs = newVnode.$attrs$ || EMPTY_OBJ;
if (BUILD.updatable) {
// remove attributes no longer present on the vnode by setting them to undefined
for (memberName in oldVnodeAttrs) {
if (!(memberName in newVnodeAttrs)) {
setAccessor(elm, memberName, oldVnodeAttrs[memberName], undefined, isSvgMode, newVnode.$flags$);
}
}
}
// add new & update changed attributes
for (memberName in newVnodeAttrs) {
setAccessor(elm, memberName, oldVnodeAttrs[memberName], newVnodeAttrs[memberName], isSvgMode, newVnode.$flags$);
}
};
var createElm = function (oldParentVNode, newParentVNode, childIndex, parentElm) {
// tslint:disable-next-line: prefer-const
var newVNode = newParentVNode.$children$[childIndex];
var i = 0;
var elm;
var childNode;
var oldVNode;
if (BUILD.slotRelocation && !useNativeShadowDom) {
// remember for later we need to check to relocate nodes
checkSlotRelocate = true;
if (newVNode.$tag$ === 'slot') {
if (scopeId) {
// scoped css needs to add its scoped id to the parent element
parentElm.classList.add(scopeId + '-s');
}
newVNode.$flags$ |= newVNode.$children$
? // slot element has fallback content
2 /* isSlotFallback */
: // slot element does not have fallback content
1 /* isSlotReference */;
}
}
if (BUILD.isDev && newVNode.$elm$) {
consoleError("The JSX " + (newVNode.$text$ !== null ? "\"" + newVNode.$text$ + "\" text" : "\"" + newVNode.$tag$ + "\" element") + " node should not be shared within the same renderer. The renderer caches element lookups in order to improve performance. However, a side effect from this is that the exact same JSX node should not be reused. For more information please see https://stenciljs.com/docs/templating-jsx#avoid-shared-jsx-nodes");
}
if (BUILD.vdomText && newVNode.$text$ !== null) {
// create text node
elm = newVNode.$elm$ = doc.createTextNode(newVNode.$text$);
}
else if (BUILD.slotRelocation && newVNode.$flags$ & 1 /* isSlotReference */) {
// create a slot reference node
elm = newVNode.$elm$ = BUILD.isDebug || BUILD.hydrateServerSide ? slotReferenceDebugNode(newVNode) : doc.createTextNode('');
}
else {
if (BUILD.svg && !isSvgMode) {
isSvgMode = newVNode.$tag$ === 'svg';
}
// create element
elm = newVNode.$elm$ = (BUILD.svg
? doc.createElementNS(isSvgMode ? SVG_NS : HTML_NS, BUILD.slotRelocation && newVNode.$flags$ & 2 /* isSlotFallback */ ? 'slot-fb' : newVNode.$tag$)
: doc.createElement(BUILD.slotRelocation && newVNode.$flags$ & 2 /* isSlotFallback */ ? 'slot-fb' : newVNode.$tag$));
if (BUILD.svg && isSvgMode && newVNode.$tag$ === 'foreignObject') {
isSvgMode = false;
}
// add css classes, attrs, props, listeners, etc.
if (BUILD.vdomAttribute) {
updateElement(null, newVNode, isSvgMode);
}
if ((BUILD.shadowDom || BUILD.scoped) && isDef(scopeId) && elm['s-si'] !== scopeId) {
// if there is a scopeId and this is the initial render
// then let's add the scopeId as a css class
elm.classList.add((elm['s-si'] = scopeId));
}
if (newVNode.$children$) {
for (i = 0; i < newVNode.$children$.length; ++i) {
// create the node
childNode = createElm(oldParentVNode, newVNode, i, elm);
// return node could have been null
if (childNode) {
// append our new node
elm.appendChild(childNode);
}
}
}
if (BUILD.svg) {
if (newVNode.$tag$ === 'svg') {
// Only reset the SVG context when we're exiting <svg> element
isSvgMode = false;
}
else if (elm.tagName === 'foreignObject') {
// Reenter SVG context when we're exiting <foreignObject> element
isSvgMode = true;
}
}
}
if (BUILD.slotRelocation) {
elm['s-hn'] = hostTagName;
if (newVNode.$flags$ & (2 /* isSlotFallback */ | 1 /* isSlotReference */)) {
// remember the content reference comment
elm['s-sr'] = true;
// remember the content reference comment
elm['s-cr'] = contentRef;
// remember the slot name, or empty string for default slot
elm['s-sn'] = newVNode.$name$ || '';
// check if we've got an old vnode for this slot
oldVNode = oldParentVNode && oldParentVNode.$children$ && oldParentVNode.$children$[childIndex];
if (oldVNode && oldVNode.$tag$ === newVNode.$tag$ && oldParentVNode.$elm$) {
// we've got an old slot vnode and the wrapper is being replaced
// so let's move the old slot content back to it's original location
putBackInOriginalLocation(oldParentVNode.$elm$, false);
}
}
}
return elm;
};
var putBackInOriginalLocation = function (parentElm, recursive) {
plt.$flags$ |= 1 /* isTmpDisconnected */;
var oldSlotChildNodes = parentElm.childNodes;
for (var i_2 = oldSlotChildNodes.length - 1; i_2 >= 0; i_2--) {
var childNode = oldSlotChildNodes[i_2];
if (childNode['s-hn'] !== hostTagName && childNode['s-ol']) {
// // this child node in the old element is from another component
// // remove this node from the old slot's parent
// childNode.remove();
// and relocate it back to it's original location
parentReferenceNode(childNode).insertBefore(childNode, referenceNode(childNode));
// remove the old original location comment entirely
// later on the patch function will know what to do
// and move this to the correct spot in need be
childNode['s-ol'].remove();
childNode['s-ol'] = undefined;
checkSlotRelocate = true;
}
if (recursive) {
putBackInOriginalLocation(childNode, recursive);
}
}
plt.$flags$ &= ~1 /* isTmpDisconnected */;
};
var addVnodes = function (parentElm, before, parentVNode, vnodes, startIdx, endIdx) {
var containerElm = ((BUILD.slotRelocation && parentElm['s-cr'] && parentElm['s-cr'].parentNode) || parentElm);
var childNode;
if (BUILD.shadowDom && containerElm.shadowRoot && containerElm.tagName === hostTagName) {
containerElm = containerElm.shadowRoot;
}
for (; startIdx <= endIdx; ++startIdx) {
if (vnodes[startIdx]) {
childNode = createElm(null, parentVNode, startIdx, parentElm);
if (childNode) {
vnodes[startIdx].$elm$ = childNode;
containerElm.insertBefore(childNode, BUILD.slotRelocation ? referenceNode(before) : before);
}
}
}
};
var removeVnodes = function (vnodes, startIdx, endIdx, vnode, elm) {
for (; startIdx <= endIdx; ++startIdx) {