@pulumi/databricks
Version:
A Pulumi package for creating and managing databricks cloud resources.
332 lines • 18.9 kB
JavaScript
// *** WARNING: this file was generated by the Pulumi Terraform Bridge (tfgen) Tool. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
Object.defineProperty(exports, "__esModule", { value: true });
exports.MwsWorkspaces = void 0;
const pulumi = require("@pulumi/pulumi");
const utilities = require("./utilities");
/**
* ## Example Usage
*
* ### Creating a Databricks on AWS workspace
*
* !Simplest multiworkspace
*
* To get workspace running, you have to configure a couple of things:
*
* * databricks.MwsCredentials - You can share a credentials (cross-account IAM role) configuration ID with multiple workspaces. It is not required to create a new one for each workspace.
* * databricks.MwsStorageConfigurations - You can share a root S3 bucket with multiple workspaces in a single account. You do not have to create new ones for each workspace. If you share a root S3 bucket for multiple workspaces in an account, data on the root S3 bucket is partitioned into separate directories by workspace.
* * databricks.MwsNetworks - (optional, but recommended) You can share one [customer-managed VPC](https://docs.databricks.com/administration-guide/cloud-configurations/aws/customer-managed-vpc.html) with multiple workspaces in a single account. However, Databricks recommends using unique subnets and security groups for each workspace. If you plan to share one VPC with multiple workspaces, be sure to size your VPC and subnets accordingly. Because a Databricks databricks.MwsNetworks encapsulates this information, you cannot reuse it across workspaces.
* * databricks.MwsCustomerManagedKeys - You can share a customer-managed key across workspaces.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as databricks from "@pulumi/databricks";
*
* const config = new pulumi.Config();
* // Account ID that can be found in the dropdown under the email address in the upper-right corner of https://accounts.cloud.databricks.com/
* const databricksAccountId = config.requireObject<any>("databricksAccountId");
* // register cross-account ARN
* const _this = new databricks.MwsCredentials("this", {
* accountId: databricksAccountId,
* credentialsName: `${prefix}-creds`,
* roleArn: crossaccountArn,
* });
* // register root bucket
* const thisMwsStorageConfigurations = new databricks.MwsStorageConfigurations("this", {
* accountId: databricksAccountId,
* storageConfigurationName: `${prefix}-storage`,
* bucketName: rootBucket,
* });
* // register VPC
* const thisMwsNetworks = new databricks.MwsNetworks("this", {
* accountId: databricksAccountId,
* networkName: `${prefix}-network`,
* vpcId: vpcId,
* subnetIds: subnetsPrivate,
* securityGroupIds: [securityGroup],
* });
* // create workspace in given VPC with DBFS on root bucket
* const thisMwsWorkspaces = new databricks.MwsWorkspaces("this", {
* accountId: databricksAccountId,
* workspaceName: prefix,
* awsRegion: region,
* credentialsId: _this.credentialsId,
* storageConfigurationId: thisMwsStorageConfigurations.storageConfigurationId,
* networkId: thisMwsNetworks.networkId,
* token: {},
* });
* export const databricksToken = thisMwsWorkspaces.token.apply(token => token?.tokenValue);
* ```
*
* ### Creating a Databricks on AWS workspace with Databricks-Managed VPC
*
* 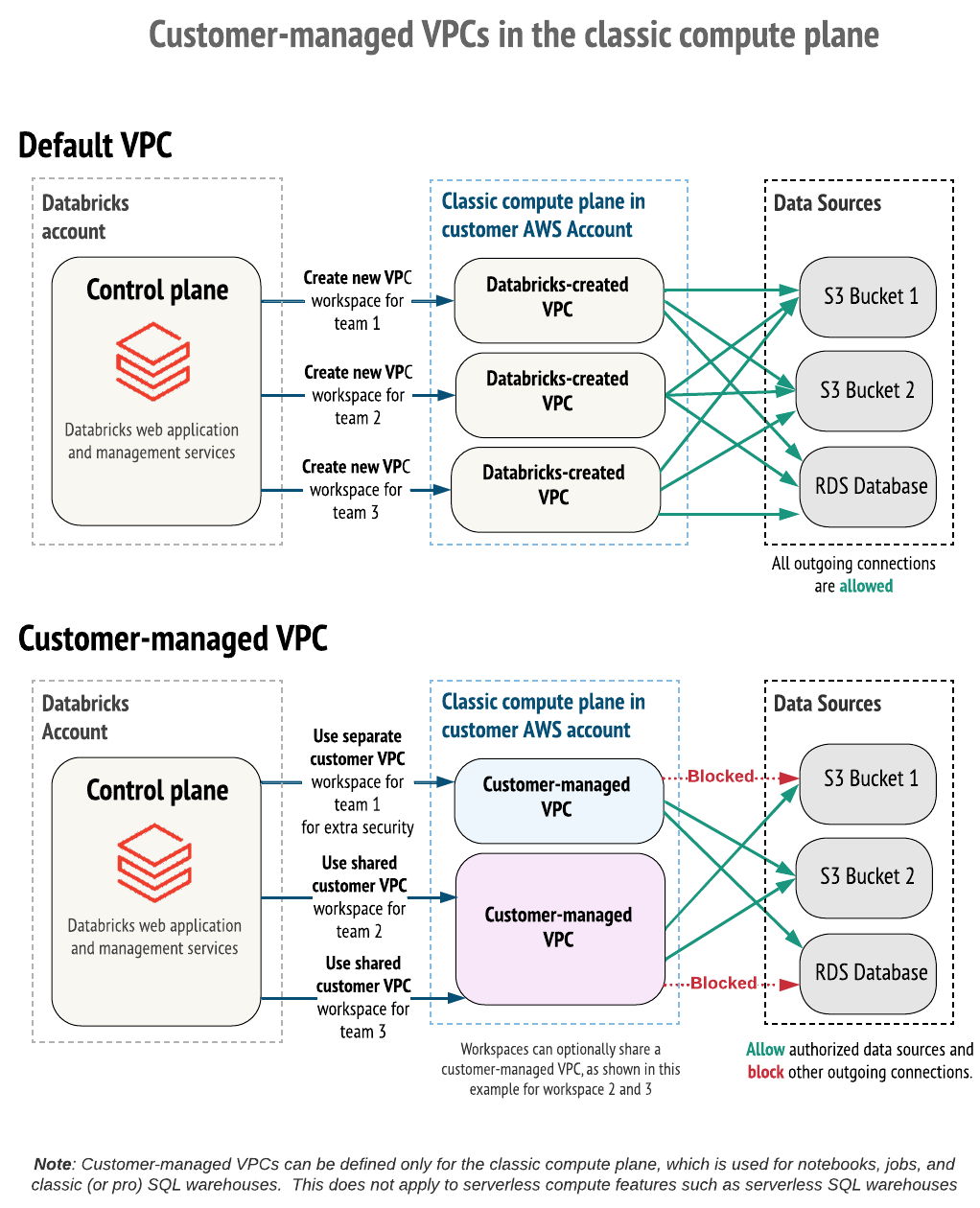
*
* By default, Databricks creates a VPC in your AWS account for each workspace. Databricks uses it for running clusters in the workspace. Optionally, you can use your VPC for the workspace, using the feature customer-managed VPC. Databricks recommends that you provide your VPC with databricks.MwsNetworks so that you can configure it according to your organization’s enterprise cloud standards while still conforming to Databricks requirements. You cannot migrate an existing workspace to your VPC. Please see the difference described through IAM policy actions [on this page](https://docs.databricks.com/administration-guide/account-api/iam-role.html).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as databricks from "@pulumi/databricks";
* import * as random from "@pulumi/random";
*
* const config = new pulumi.Config();
* // Account Id that could be found in the top right corner of https://accounts.cloud.databricks.com/
* const databricksAccountId = config.requireObject<any>("databricksAccountId");
* const naming = new random.index.String("naming", {
* special: false,
* upper: false,
* length: 6,
* });
* const prefix = `dltp${naming.result}`;
* const _this = databricks.getAwsAssumeRolePolicy({
* externalId: databricksAccountId,
* });
* const crossAccountRole = new aws.iam.Role("cross_account_role", {
* name: `${prefix}-crossaccount`,
* assumeRolePolicy: _this.then(_this => _this.json),
* tags: tags,
* });
* const thisGetAwsCrossAccountPolicy = databricks.getAwsCrossAccountPolicy({});
* const thisRolePolicy = new aws.iam.RolePolicy("this", {
* name: `${prefix}-policy`,
* role: crossAccountRole.id,
* policy: thisGetAwsCrossAccountPolicy.then(thisGetAwsCrossAccountPolicy => thisGetAwsCrossAccountPolicy.json),
* });
* const thisMwsCredentials = new databricks.MwsCredentials("this", {
* accountId: databricksAccountId,
* credentialsName: `${prefix}-creds`,
* roleArn: crossAccountRole.arn,
* });
* const rootStorageBucket = new aws.s3.BucketV2("root_storage_bucket", {
* bucket: `${prefix}-rootbucket`,
* acl: "private",
* forceDestroy: true,
* tags: tags,
* });
* const rootVersioning = new aws.s3.BucketVersioningV2("root_versioning", {
* bucket: rootStorageBucket.id,
* versioningConfiguration: {
* status: "Disabled",
* },
* });
* const rootStorageBucketBucketServerSideEncryptionConfigurationV2 = new aws.s3.BucketServerSideEncryptionConfigurationV2("root_storage_bucket", {
* bucket: rootStorageBucket.bucket,
* rules: [{
* applyServerSideEncryptionByDefault: {
* sseAlgorithm: "AES256",
* },
* }],
* });
* const rootStorageBucketBucketPublicAccessBlock = new aws.s3.BucketPublicAccessBlock("root_storage_bucket", {
* bucket: rootStorageBucket.id,
* blockPublicAcls: true,
* blockPublicPolicy: true,
* ignorePublicAcls: true,
* restrictPublicBuckets: true,
* }, {
* dependsOn: [rootStorageBucket],
* });
* const thisGetAwsBucketPolicy = databricks.getAwsBucketPolicyOutput({
* bucket: rootStorageBucket.bucket,
* });
* const rootBucketPolicy = new aws.s3.BucketPolicy("root_bucket_policy", {
* bucket: rootStorageBucket.id,
* policy: thisGetAwsBucketPolicy.apply(thisGetAwsBucketPolicy => thisGetAwsBucketPolicy.json),
* }, {
* dependsOn: [rootStorageBucketBucketPublicAccessBlock],
* });
* const thisMwsStorageConfigurations = new databricks.MwsStorageConfigurations("this", {
* accountId: databricksAccountId,
* storageConfigurationName: `${prefix}-storage`,
* bucketName: rootStorageBucket.bucket,
* });
* const thisMwsWorkspaces = new databricks.MwsWorkspaces("this", {
* accountId: databricksAccountId,
* workspaceName: prefix,
* awsRegion: "us-east-1",
* credentialsId: thisMwsCredentials.credentialsId,
* storageConfigurationId: thisMwsStorageConfigurations.storageConfigurationId,
* token: {},
* customTags: {
* SoldToCode: "1234",
* },
* });
* export const databricksToken = thisMwsWorkspaces.token.apply(token => token?.tokenValue);
* ```
*
* In order to create a [Databricks Workspace that leverages AWS PrivateLink](https://docs.databricks.com/administration-guide/cloud-configurations/aws/privatelink.html) please ensure that you have read and understood the [Enable Private Link](https://docs.databricks.com/administration-guide/cloud-configurations/aws/privatelink.html) documentation and then customise the example above with the relevant examples from mws_vpc_endpoint, mwsPrivateAccessSettings and mws_networks.
*
* ### Creating a Databricks on GCP workspace
*
* To get workspace running, you have to configure a network object:
*
* * databricks.MwsNetworks - (optional, but recommended) You can share one [customer-managed VPC](https://docs.gcp.databricks.com/administration-guide/cloud-configurations/gcp/customer-managed-vpc.html) with multiple workspaces in a single account. You do not have to create a new VPC for each workspace. However, you cannot reuse subnets with other resources, including other workspaces or non-Databricks resources. If you plan to share one VPC with multiple workspaces, be sure to size your VPC and subnets accordingly. Because a Databricks databricks.MwsNetworks encapsulates this information, you cannot reuse it across workspaces.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as databricks from "@pulumi/databricks";
*
* const config = new pulumi.Config();
* // Account Id that could be found in the top right corner of https://accounts.cloud.databricks.com/
* const databricksAccountId = config.requireObject<any>("databricksAccountId");
* const databricksGoogleServiceAccount = config.requireObject<any>("databricksGoogleServiceAccount");
* const googleProject = config.requireObject<any>("googleProject");
* // register VPC
* const _this = new databricks.MwsNetworks("this", {
* accountId: databricksAccountId,
* networkName: `${prefix}-network`,
* gcpNetworkInfo: {
* networkProjectId: googleProject,
* vpcId: vpcId,
* subnetId: subnetId,
* subnetRegion: subnetRegion,
* podIpRangeName: "pods",
* serviceIpRangeName: "svc",
* },
* });
* // create workspace in given VPC
* const thisMwsWorkspaces = new databricks.MwsWorkspaces("this", {
* accountId: databricksAccountId,
* workspaceName: prefix,
* location: subnetRegion,
* cloudResourceContainer: {
* gcp: {
* projectId: googleProject,
* },
* },
* networkId: _this.networkId,
* gkeConfig: {
* connectivityType: "PRIVATE_NODE_PUBLIC_MASTER",
* masterIpRange: "10.3.0.0/28",
* },
* token: {},
* });
* export const databricksToken = thisMwsWorkspaces.token.apply(token => token?.tokenValue);
* ```
*
* In order to create a [Databricks Workspace that leverages GCP Private Service Connect](https://docs.gcp.databricks.com/administration-guide/cloud-configurations/gcp/private-service-connect.html) please ensure that you have read and understood the [Enable Private Service Connect](https://docs.gcp.databricks.com/administration-guide/cloud-configurations/gcp/private-service-connect.html) documentation and then customise the example above with the relevant examples from mws_vpc_endpoint, mwsPrivateAccessSettings and mws_networks.
*
* ## Import
*
* This resource can be imported by Databricks account ID and workspace ID.
*
* ```sh
* $ pulumi import databricks:index/mwsWorkspaces:MwsWorkspaces this '<account_id>/<workspace_id>'
* ```
*
* ~> Not all fields of `databricks_mws_workspaces` can be updated without causing the workspace to be recreated.
*
* If the configuration for these immutable fields does not match the existing workspace, the workspace will
*
* be deleted and recreated in the next `pulumi up`. After importing, verify that the configuration
*
* matches the existing resource by running `pulumi preview`. The only fields that can be updated are
*
* `credentials_id`, `network_id`, `storage_customer_managed_key_id`, `private_access_settings_id`,
*
* `managed_services_customer_managed_key_id`, and `custom_tags`.
*/
class MwsWorkspaces extends pulumi.CustomResource {
/**
* Get an existing MwsWorkspaces resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state Any extra arguments used during the lookup.
* @param opts Optional settings to control the behavior of the CustomResource.
*/
static get(name, id, state, opts) {
return new MwsWorkspaces(name, state, Object.assign(Object.assign({}, opts), { id: id }));
}
/**
* Returns true if the given object is an instance of MwsWorkspaces. This is designed to work even
* when multiple copies of the Pulumi SDK have been loaded into the same process.
*/
static isInstance(obj) {
if (obj === undefined || obj === null) {
return false;
}
return obj['__pulumiType'] === MwsWorkspaces.__pulumiType;
}
constructor(name, argsOrState, opts) {
let resourceInputs = {};
opts = opts || {};
if (opts.id) {
const state = argsOrState;
resourceInputs["accountId"] = state ? state.accountId : undefined;
resourceInputs["awsRegion"] = state ? state.awsRegion : undefined;
resourceInputs["cloud"] = state ? state.cloud : undefined;
resourceInputs["cloudResourceContainer"] = state ? state.cloudResourceContainer : undefined;
resourceInputs["creationTime"] = state ? state.creationTime : undefined;
resourceInputs["credentialsId"] = state ? state.credentialsId : undefined;
resourceInputs["customTags"] = state ? state.customTags : undefined;
resourceInputs["customerManagedKeyId"] = state ? state.customerManagedKeyId : undefined;
resourceInputs["deploymentName"] = state ? state.deploymentName : undefined;
resourceInputs["externalCustomerInfo"] = state ? state.externalCustomerInfo : undefined;
resourceInputs["gcpManagedNetworkConfig"] = state ? state.gcpManagedNetworkConfig : undefined;
resourceInputs["gcpWorkspaceSa"] = state ? state.gcpWorkspaceSa : undefined;
resourceInputs["gkeConfig"] = state ? state.gkeConfig : undefined;
resourceInputs["isNoPublicIpEnabled"] = state ? state.isNoPublicIpEnabled : undefined;
resourceInputs["location"] = state ? state.location : undefined;
resourceInputs["managedServicesCustomerManagedKeyId"] = state ? state.managedServicesCustomerManagedKeyId : undefined;
resourceInputs["networkId"] = state ? state.networkId : undefined;
resourceInputs["pricingTier"] = state ? state.pricingTier : undefined;
resourceInputs["privateAccessSettingsId"] = state ? state.privateAccessSettingsId : undefined;
resourceInputs["storageConfigurationId"] = state ? state.storageConfigurationId : undefined;
resourceInputs["storageCustomerManagedKeyId"] = state ? state.storageCustomerManagedKeyId : undefined;
resourceInputs["token"] = state ? state.token : undefined;
resourceInputs["workspaceId"] = state ? state.workspaceId : undefined;
resourceInputs["workspaceName"] = state ? state.workspaceName : undefined;
resourceInputs["workspaceStatus"] = state ? state.workspaceStatus : undefined;
resourceInputs["workspaceStatusMessage"] = state ? state.workspaceStatusMessage : undefined;
resourceInputs["workspaceUrl"] = state ? state.workspaceUrl : undefined;
}
else {
const args = argsOrState;
if ((!args || args.accountId === undefined) && !opts.urn) {
throw new Error("Missing required property 'accountId'");
}
if ((!args || args.workspaceName === undefined) && !opts.urn) {
throw new Error("Missing required property 'workspaceName'");
}
resourceInputs["accountId"] = (args === null || args === void 0 ? void 0 : args.accountId) ? pulumi.secret(args.accountId) : undefined;
resourceInputs["awsRegion"] = args ? args.awsRegion : undefined;
resourceInputs["cloud"] = args ? args.cloud : undefined;
resourceInputs["cloudResourceContainer"] = args ? args.cloudResourceContainer : undefined;
resourceInputs["creationTime"] = args ? args.creationTime : undefined;
resourceInputs["credentialsId"] = args ? args.credentialsId : undefined;
resourceInputs["customTags"] = args ? args.customTags : undefined;
resourceInputs["customerManagedKeyId"] = args ? args.customerManagedKeyId : undefined;
resourceInputs["deploymentName"] = args ? args.deploymentName : undefined;
resourceInputs["externalCustomerInfo"] = args ? args.externalCustomerInfo : undefined;
resourceInputs["gcpManagedNetworkConfig"] = args ? args.gcpManagedNetworkConfig : undefined;
resourceInputs["gkeConfig"] = args ? args.gkeConfig : undefined;
resourceInputs["isNoPublicIpEnabled"] = args ? args.isNoPublicIpEnabled : undefined;
resourceInputs["location"] = args ? args.location : undefined;
resourceInputs["managedServicesCustomerManagedKeyId"] = args ? args.managedServicesCustomerManagedKeyId : undefined;
resourceInputs["networkId"] = args ? args.networkId : undefined;
resourceInputs["pricingTier"] = args ? args.pricingTier : undefined;
resourceInputs["privateAccessSettingsId"] = args ? args.privateAccessSettingsId : undefined;
resourceInputs["storageConfigurationId"] = args ? args.storageConfigurationId : undefined;
resourceInputs["storageCustomerManagedKeyId"] = args ? args.storageCustomerManagedKeyId : undefined;
resourceInputs["token"] = args ? args.token : undefined;
resourceInputs["workspaceId"] = args ? args.workspaceId : undefined;
resourceInputs["workspaceName"] = args ? args.workspaceName : undefined;
resourceInputs["workspaceStatus"] = args ? args.workspaceStatus : undefined;
resourceInputs["workspaceStatusMessage"] = args ? args.workspaceStatusMessage : undefined;
resourceInputs["workspaceUrl"] = args ? args.workspaceUrl : undefined;
resourceInputs["gcpWorkspaceSa"] = undefined /*out*/;
}
opts = pulumi.mergeOptions(utilities.resourceOptsDefaults(), opts);
const secretOpts = { additionalSecretOutputs: ["accountId"] };
opts = pulumi.mergeOptions(opts, secretOpts);
super(MwsWorkspaces.__pulumiType, name, resourceInputs, opts);
}
}
exports.MwsWorkspaces = MwsWorkspaces;
/** @internal */
MwsWorkspaces.__pulumiType = 'databricks:index/mwsWorkspaces:MwsWorkspaces';
//# sourceMappingURL=mwsWorkspaces.js.map
;